find words that start with specific letter
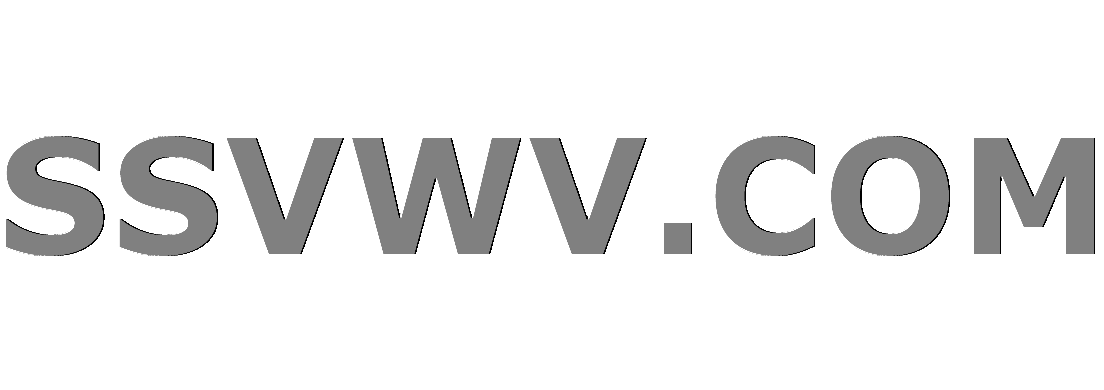
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty height:90px;width:728px;box-sizing:border-box;
I'm trying to read file.txt with some content like this:
jone
sia
alex
jad
and using node js I want to find the words that start with letter j
this is what I write:
let fs = require('fs');
fs.readFile('./file.txt', (err, info)=>
let j;
let con = 0;
console.log(err)
if(err) return;
for (j=0; j < info.length; ++j)
if (info[j] == 10)
con++;
console.log(con)
if(info.indexOf('j') > -1)
console.log(info.toString())
)
I want to print the number of lines in text file and the words that start with letter j
but the counter result is 3 while it should be 4 and it prints all info:
jone
sia
alex
jad
while it should print jone and jad only
how solve this
node.js
add a comment |
I'm trying to read file.txt with some content like this:
jone
sia
alex
jad
and using node js I want to find the words that start with letter j
this is what I write:
let fs = require('fs');
fs.readFile('./file.txt', (err, info)=>
let j;
let con = 0;
console.log(err)
if(err) return;
for (j=0; j < info.length; ++j)
if (info[j] == 10)
con++;
console.log(con)
if(info.indexOf('j') > -1)
console.log(info.toString())
)
I want to print the number of lines in text file and the words that start with letter j
but the counter result is 3 while it should be 4 and it prints all info:
jone
sia
alex
jad
while it should print jone and jad only
how solve this
node.js
add a comment |
I'm trying to read file.txt with some content like this:
jone
sia
alex
jad
and using node js I want to find the words that start with letter j
this is what I write:
let fs = require('fs');
fs.readFile('./file.txt', (err, info)=>
let j;
let con = 0;
console.log(err)
if(err) return;
for (j=0; j < info.length; ++j)
if (info[j] == 10)
con++;
console.log(con)
if(info.indexOf('j') > -1)
console.log(info.toString())
)
I want to print the number of lines in text file and the words that start with letter j
but the counter result is 3 while it should be 4 and it prints all info:
jone
sia
alex
jad
while it should print jone and jad only
how solve this
node.js
I'm trying to read file.txt with some content like this:
jone
sia
alex
jad
and using node js I want to find the words that start with letter j
this is what I write:
let fs = require('fs');
fs.readFile('./file.txt', (err, info)=>
let j;
let con = 0;
console.log(err)
if(err) return;
for (j=0; j < info.length; ++j)
if (info[j] == 10)
con++;
console.log(con)
if(info.indexOf('j') > -1)
console.log(info.toString())
)
I want to print the number of lines in text file and the words that start with letter j
but the counter result is 3 while it should be 4 and it prints all info:
jone
sia
alex
jad
while it should print jone and jad only
how solve this
node.js
node.js
asked Nov 13 '18 at 20:55
GNDevsGNDevs
18210
18210
add a comment |
add a comment |
4 Answers
4
active
oldest
votes
Here is your file.txt:
jone
sia
alex
jad
And here is the code:
const fs = require('fs');
const output = fs
.readFileSync('file.txt', 'utf8')
.trim()
.split('n')
.filter(word => word.includes('j'));
console.log(output);
This will get you everything that includes 'j'
If you want something that will start with j you can write your own filter function like so:
const fs = require('fs');
const output = fs
.readFileSync('file.txt', 'utf8')
.trim()
.split('n');
function filter(names, index, letter)
return names.filter(word => word.charAt(index) === letter);
console.log('Length of originanl list: ', output.length);
console.log('Filtered List: ', filter(output, 0, 'j'));
console.log('Length of filtered list: ', filter(output, 0, 'j').length);
Live Demo Here
it works fine but what about the counter of words?
– GNDevs
Nov 13 '18 at 21:40
What do you mean by counter? You mean how many words?
– SakoBu
Nov 13 '18 at 21:45
I mean number of lines in file which should be 4 here
– GNDevs
Nov 13 '18 at 21:56
So the number of lines in the original file, not the filtered results? You can simply call.length
on the output...
– SakoBu
Nov 13 '18 at 21:58
thanks I accept your answer but if you can please help me with how to get the number of lines which not start with letter j which should be 2 here
– GNDevs
Nov 13 '18 at 22:46
|
show 1 more comment
You can use the readline
build in core module and do something like this:
var rl = require('readline'), fs = require('fs')
var lineReader = rl.createInterface( input: fs.createReadStream('file.txt') )
lineReader.on('line', function (name)
if(name.startsWith('j'))
console.log(name)
)
See it working here
add a comment |
I suggest using the regular expressions approach.
info
should be of type string
, therefore it is possible for you to use the String.prototype
function match()
, like so:
let matches = info.match(expression);
or in your case: let matches = info.match(/bjw+/g);
(example)
you can use regex to detect how many lines the text files has by detecting how many line breaks
you have in your raw data (if possible):
let lines = info.match(/n/g).length + 1;
(example)
add a comment |
All these answers are incredibly over-complicated.
Simply iterate through all words and check if it start with the letter:
for (const word of wordList.split("n"))
if (word.startsWith("j")) console.log(`$word start with the letter j`);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53289362%2ffind-words-that-start-with-specific-letter%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
Here is your file.txt:
jone
sia
alex
jad
And here is the code:
const fs = require('fs');
const output = fs
.readFileSync('file.txt', 'utf8')
.trim()
.split('n')
.filter(word => word.includes('j'));
console.log(output);
This will get you everything that includes 'j'
If you want something that will start with j you can write your own filter function like so:
const fs = require('fs');
const output = fs
.readFileSync('file.txt', 'utf8')
.trim()
.split('n');
function filter(names, index, letter)
return names.filter(word => word.charAt(index) === letter);
console.log('Length of originanl list: ', output.length);
console.log('Filtered List: ', filter(output, 0, 'j'));
console.log('Length of filtered list: ', filter(output, 0, 'j').length);
Live Demo Here
it works fine but what about the counter of words?
– GNDevs
Nov 13 '18 at 21:40
What do you mean by counter? You mean how many words?
– SakoBu
Nov 13 '18 at 21:45
I mean number of lines in file which should be 4 here
– GNDevs
Nov 13 '18 at 21:56
So the number of lines in the original file, not the filtered results? You can simply call.length
on the output...
– SakoBu
Nov 13 '18 at 21:58
thanks I accept your answer but if you can please help me with how to get the number of lines which not start with letter j which should be 2 here
– GNDevs
Nov 13 '18 at 22:46
|
show 1 more comment
Here is your file.txt:
jone
sia
alex
jad
And here is the code:
const fs = require('fs');
const output = fs
.readFileSync('file.txt', 'utf8')
.trim()
.split('n')
.filter(word => word.includes('j'));
console.log(output);
This will get you everything that includes 'j'
If you want something that will start with j you can write your own filter function like so:
const fs = require('fs');
const output = fs
.readFileSync('file.txt', 'utf8')
.trim()
.split('n');
function filter(names, index, letter)
return names.filter(word => word.charAt(index) === letter);
console.log('Length of originanl list: ', output.length);
console.log('Filtered List: ', filter(output, 0, 'j'));
console.log('Length of filtered list: ', filter(output, 0, 'j').length);
Live Demo Here
it works fine but what about the counter of words?
– GNDevs
Nov 13 '18 at 21:40
What do you mean by counter? You mean how many words?
– SakoBu
Nov 13 '18 at 21:45
I mean number of lines in file which should be 4 here
– GNDevs
Nov 13 '18 at 21:56
So the number of lines in the original file, not the filtered results? You can simply call.length
on the output...
– SakoBu
Nov 13 '18 at 21:58
thanks I accept your answer but if you can please help me with how to get the number of lines which not start with letter j which should be 2 here
– GNDevs
Nov 13 '18 at 22:46
|
show 1 more comment
Here is your file.txt:
jone
sia
alex
jad
And here is the code:
const fs = require('fs');
const output = fs
.readFileSync('file.txt', 'utf8')
.trim()
.split('n')
.filter(word => word.includes('j'));
console.log(output);
This will get you everything that includes 'j'
If you want something that will start with j you can write your own filter function like so:
const fs = require('fs');
const output = fs
.readFileSync('file.txt', 'utf8')
.trim()
.split('n');
function filter(names, index, letter)
return names.filter(word => word.charAt(index) === letter);
console.log('Length of originanl list: ', output.length);
console.log('Filtered List: ', filter(output, 0, 'j'));
console.log('Length of filtered list: ', filter(output, 0, 'j').length);
Live Demo Here
Here is your file.txt:
jone
sia
alex
jad
And here is the code:
const fs = require('fs');
const output = fs
.readFileSync('file.txt', 'utf8')
.trim()
.split('n')
.filter(word => word.includes('j'));
console.log(output);
This will get you everything that includes 'j'
If you want something that will start with j you can write your own filter function like so:
const fs = require('fs');
const output = fs
.readFileSync('file.txt', 'utf8')
.trim()
.split('n');
function filter(names, index, letter)
return names.filter(word => word.charAt(index) === letter);
console.log('Length of originanl list: ', output.length);
console.log('Filtered List: ', filter(output, 0, 'j'));
console.log('Length of filtered list: ', filter(output, 0, 'j').length);
Live Demo Here
edited Nov 13 '18 at 23:14
answered Nov 13 '18 at 21:10


SakoBuSakoBu
1,848621
1,848621
it works fine but what about the counter of words?
– GNDevs
Nov 13 '18 at 21:40
What do you mean by counter? You mean how many words?
– SakoBu
Nov 13 '18 at 21:45
I mean number of lines in file which should be 4 here
– GNDevs
Nov 13 '18 at 21:56
So the number of lines in the original file, not the filtered results? You can simply call.length
on the output...
– SakoBu
Nov 13 '18 at 21:58
thanks I accept your answer but if you can please help me with how to get the number of lines which not start with letter j which should be 2 here
– GNDevs
Nov 13 '18 at 22:46
|
show 1 more comment
it works fine but what about the counter of words?
– GNDevs
Nov 13 '18 at 21:40
What do you mean by counter? You mean how many words?
– SakoBu
Nov 13 '18 at 21:45
I mean number of lines in file which should be 4 here
– GNDevs
Nov 13 '18 at 21:56
So the number of lines in the original file, not the filtered results? You can simply call.length
on the output...
– SakoBu
Nov 13 '18 at 21:58
thanks I accept your answer but if you can please help me with how to get the number of lines which not start with letter j which should be 2 here
– GNDevs
Nov 13 '18 at 22:46
it works fine but what about the counter of words?
– GNDevs
Nov 13 '18 at 21:40
it works fine but what about the counter of words?
– GNDevs
Nov 13 '18 at 21:40
What do you mean by counter? You mean how many words?
– SakoBu
Nov 13 '18 at 21:45
What do you mean by counter? You mean how many words?
– SakoBu
Nov 13 '18 at 21:45
I mean number of lines in file which should be 4 here
– GNDevs
Nov 13 '18 at 21:56
I mean number of lines in file which should be 4 here
– GNDevs
Nov 13 '18 at 21:56
So the number of lines in the original file, not the filtered results? You can simply call
.length
on the output...– SakoBu
Nov 13 '18 at 21:58
So the number of lines in the original file, not the filtered results? You can simply call
.length
on the output...– SakoBu
Nov 13 '18 at 21:58
thanks I accept your answer but if you can please help me with how to get the number of lines which not start with letter j which should be 2 here
– GNDevs
Nov 13 '18 at 22:46
thanks I accept your answer but if you can please help me with how to get the number of lines which not start with letter j which should be 2 here
– GNDevs
Nov 13 '18 at 22:46
|
show 1 more comment
You can use the readline
build in core module and do something like this:
var rl = require('readline'), fs = require('fs')
var lineReader = rl.createInterface( input: fs.createReadStream('file.txt') )
lineReader.on('line', function (name)
if(name.startsWith('j'))
console.log(name)
)
See it working here
add a comment |
You can use the readline
build in core module and do something like this:
var rl = require('readline'), fs = require('fs')
var lineReader = rl.createInterface( input: fs.createReadStream('file.txt') )
lineReader.on('line', function (name)
if(name.startsWith('j'))
console.log(name)
)
See it working here
add a comment |
You can use the readline
build in core module and do something like this:
var rl = require('readline'), fs = require('fs')
var lineReader = rl.createInterface( input: fs.createReadStream('file.txt') )
lineReader.on('line', function (name)
if(name.startsWith('j'))
console.log(name)
)
See it working here
You can use the readline
build in core module and do something like this:
var rl = require('readline'), fs = require('fs')
var lineReader = rl.createInterface( input: fs.createReadStream('file.txt') )
lineReader.on('line', function (name)
if(name.startsWith('j'))
console.log(name)
)
See it working here
edited Nov 13 '18 at 21:35
answered Nov 13 '18 at 21:01


AkrionAkrion
9,58011224
9,58011224
add a comment |
add a comment |
I suggest using the regular expressions approach.
info
should be of type string
, therefore it is possible for you to use the String.prototype
function match()
, like so:
let matches = info.match(expression);
or in your case: let matches = info.match(/bjw+/g);
(example)
you can use regex to detect how many lines the text files has by detecting how many line breaks
you have in your raw data (if possible):
let lines = info.match(/n/g).length + 1;
(example)
add a comment |
I suggest using the regular expressions approach.
info
should be of type string
, therefore it is possible for you to use the String.prototype
function match()
, like so:
let matches = info.match(expression);
or in your case: let matches = info.match(/bjw+/g);
(example)
you can use regex to detect how many lines the text files has by detecting how many line breaks
you have in your raw data (if possible):
let lines = info.match(/n/g).length + 1;
(example)
add a comment |
I suggest using the regular expressions approach.
info
should be of type string
, therefore it is possible for you to use the String.prototype
function match()
, like so:
let matches = info.match(expression);
or in your case: let matches = info.match(/bjw+/g);
(example)
you can use regex to detect how many lines the text files has by detecting how many line breaks
you have in your raw data (if possible):
let lines = info.match(/n/g).length + 1;
(example)
I suggest using the regular expressions approach.
info
should be of type string
, therefore it is possible for you to use the String.prototype
function match()
, like so:
let matches = info.match(expression);
or in your case: let matches = info.match(/bjw+/g);
(example)
you can use regex to detect how many lines the text files has by detecting how many line breaks
you have in your raw data (if possible):
let lines = info.match(/n/g).length + 1;
(example)
answered Nov 13 '18 at 21:17


Omer ShukarOmer Shukar
420514
420514
add a comment |
add a comment |
All these answers are incredibly over-complicated.
Simply iterate through all words and check if it start with the letter:
for (const word of wordList.split("n"))
if (word.startsWith("j")) console.log(`$word start with the letter j`);
add a comment |
All these answers are incredibly over-complicated.
Simply iterate through all words and check if it start with the letter:
for (const word of wordList.split("n"))
if (word.startsWith("j")) console.log(`$word start with the letter j`);
add a comment |
All these answers are incredibly over-complicated.
Simply iterate through all words and check if it start with the letter:
for (const word of wordList.split("n"))
if (word.startsWith("j")) console.log(`$word start with the letter j`);
All these answers are incredibly over-complicated.
Simply iterate through all words and check if it start with the letter:
for (const word of wordList.split("n"))
if (word.startsWith("j")) console.log(`$word start with the letter j`);
answered Nov 14 '18 at 13:47
OliverOliver
160413
160413
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53289362%2ffind-words-that-start-with-specific-letter%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
eVjjaU sJwTLzRCgv,OSJ5rst7d1YcPDMIQP,1eyzP1O8P6paiApRGB