Unity3d Smoothly Rotate an object based on a list of Pitch, Roll and Yaw angles
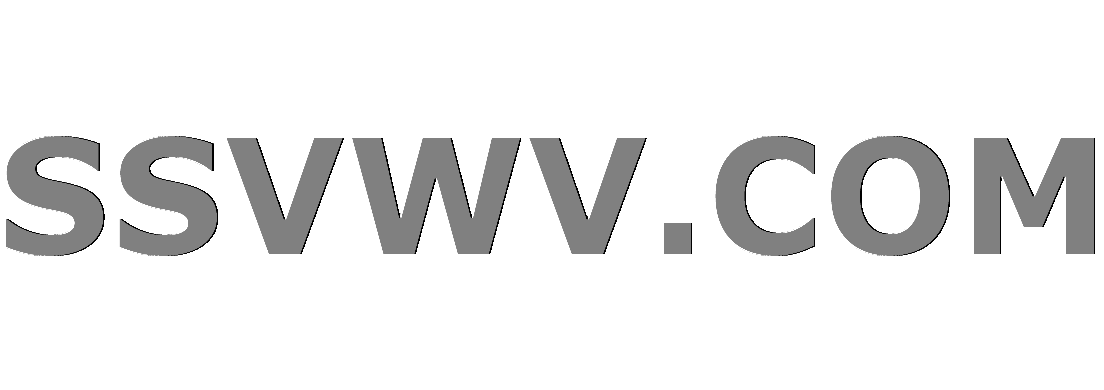
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty height:90px;width:728px;box-sizing:border-box;
I want to smoothly rotate an object(a plane) based on a list of angles (Pitch, Roll and Yaw) I get from an API call. The reponse object is the Rootresponse below
public class ResponseData
public List<int> x; //roll
public List<int> y; //yaw
public List<int> z; //pitch
public class RootResponse
public ResponseData data;
public string status; //status of the api call
I've tried to loop through the values each frame in the FixedUpdate method using a while loop using the below piece of code. This throws an "ArgumentOutOfRange" exception.
If I use transform.Roatate or Quarternion angle as per the documentation I'm only able to get the final position.
What is the best approach I can opt for in this case?
void FixedUpdate()
if(shouldUpdate) //set to true for a success response from the api call
while(ind < dataLen)
transform.position = Vector3.MoveTowards(new Vector3(batData.x[ind], batData.y[ind], batData.z[ind]), new Vector3(batData.x[ind+1], batData.y[ind + 1], batData.z[ind + 1]), speed * Time.deltaTime);
ind++; //to increment the index every frame
c# unity3d
add a comment |
I want to smoothly rotate an object(a plane) based on a list of angles (Pitch, Roll and Yaw) I get from an API call. The reponse object is the Rootresponse below
public class ResponseData
public List<int> x; //roll
public List<int> y; //yaw
public List<int> z; //pitch
public class RootResponse
public ResponseData data;
public string status; //status of the api call
I've tried to loop through the values each frame in the FixedUpdate method using a while loop using the below piece of code. This throws an "ArgumentOutOfRange" exception.
If I use transform.Roatate or Quarternion angle as per the documentation I'm only able to get the final position.
What is the best approach I can opt for in this case?
void FixedUpdate()
if(shouldUpdate) //set to true for a success response from the api call
while(ind < dataLen)
transform.position = Vector3.MoveTowards(new Vector3(batData.x[ind], batData.y[ind], batData.z[ind]), new Vector3(batData.x[ind+1], batData.y[ind + 1], batData.z[ind + 1]), speed * Time.deltaTime);
ind++; //to increment the index every frame
c# unity3d
add a comment |
I want to smoothly rotate an object(a plane) based on a list of angles (Pitch, Roll and Yaw) I get from an API call. The reponse object is the Rootresponse below
public class ResponseData
public List<int> x; //roll
public List<int> y; //yaw
public List<int> z; //pitch
public class RootResponse
public ResponseData data;
public string status; //status of the api call
I've tried to loop through the values each frame in the FixedUpdate method using a while loop using the below piece of code. This throws an "ArgumentOutOfRange" exception.
If I use transform.Roatate or Quarternion angle as per the documentation I'm only able to get the final position.
What is the best approach I can opt for in this case?
void FixedUpdate()
if(shouldUpdate) //set to true for a success response from the api call
while(ind < dataLen)
transform.position = Vector3.MoveTowards(new Vector3(batData.x[ind], batData.y[ind], batData.z[ind]), new Vector3(batData.x[ind+1], batData.y[ind + 1], batData.z[ind + 1]), speed * Time.deltaTime);
ind++; //to increment the index every frame
c# unity3d
I want to smoothly rotate an object(a plane) based on a list of angles (Pitch, Roll and Yaw) I get from an API call. The reponse object is the Rootresponse below
public class ResponseData
public List<int> x; //roll
public List<int> y; //yaw
public List<int> z; //pitch
public class RootResponse
public ResponseData data;
public string status; //status of the api call
I've tried to loop through the values each frame in the FixedUpdate method using a while loop using the below piece of code. This throws an "ArgumentOutOfRange" exception.
If I use transform.Roatate or Quarternion angle as per the documentation I'm only able to get the final position.
What is the best approach I can opt for in this case?
void FixedUpdate()
if(shouldUpdate) //set to true for a success response from the api call
while(ind < dataLen)
transform.position = Vector3.MoveTowards(new Vector3(batData.x[ind], batData.y[ind], batData.z[ind]), new Vector3(batData.x[ind+1], batData.y[ind + 1], batData.z[ind + 1]), speed * Time.deltaTime);
ind++; //to increment the index every frame
c# unity3d
c# unity3d
edited Nov 13 '18 at 23:21
Ruzihm
3,72611628
3,72611628
asked Nov 13 '18 at 22:35


Lohit PeesapatiLohit Peesapati
187
187
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You (probably) don't want to apply all of the rotations inside the span of one frame regardless of rotational speed.
Rather, you should keep track of how much you are rotating during the course of working through the queue that frame, and get out of the while loop if you meet that.
public float maxAngleRotatePerFrame = 5f;
void FixedUpdate()
if(shouldUpdate) //set to true for a success response from the api call
// Keep track of how far we've traveled in this frame.
float angleTraveledThisFrame = 0f;
// Rotate until we've rotated as much as we can in a single frame,
// or we run out of rotations to do.
while (angleTraveledThisFrame < maxAngleRotatePerFrame && ind < dataLen)
// Figure out how we want to rotate and how much that is
Quaternion curRot = transform.rotation;
Quaternion goalRot = Quaternion.Euler(
batData.x[ind],
batData.y[ind],
batData.z[ind]
);
float angleLeftInThisInd = Quaternion.Angle(curRot, goalRot);
// Rotate as much as we can toward that rotation this frame
float curAngleRotate = Mathf.Min(
angleLeftInThisInd,
maxAngleRotatePerFrame - angleTraveledThisFrame
);
transform.rotation = Quaternion.RotateTowards(curRot, goalRot, curAngleRotate);
// Update how much we've rotated already. This determines
// if we get to go through the while loop again.
angleTraveledThisFrame += curAngleRotate;
if (angleTraveledThisFrame < maxAngleRotatePerFrame )
// If we have more rotating to do this frame,
// increment the index.
ind++;
if (ind==dataLen)
// If you need to do anything when you run out of rotations,
// you can do it here.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53290539%2funity3d-smoothly-rotate-an-object-based-on-a-list-of-pitch-roll-and-yaw-angles%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You (probably) don't want to apply all of the rotations inside the span of one frame regardless of rotational speed.
Rather, you should keep track of how much you are rotating during the course of working through the queue that frame, and get out of the while loop if you meet that.
public float maxAngleRotatePerFrame = 5f;
void FixedUpdate()
if(shouldUpdate) //set to true for a success response from the api call
// Keep track of how far we've traveled in this frame.
float angleTraveledThisFrame = 0f;
// Rotate until we've rotated as much as we can in a single frame,
// or we run out of rotations to do.
while (angleTraveledThisFrame < maxAngleRotatePerFrame && ind < dataLen)
// Figure out how we want to rotate and how much that is
Quaternion curRot = transform.rotation;
Quaternion goalRot = Quaternion.Euler(
batData.x[ind],
batData.y[ind],
batData.z[ind]
);
float angleLeftInThisInd = Quaternion.Angle(curRot, goalRot);
// Rotate as much as we can toward that rotation this frame
float curAngleRotate = Mathf.Min(
angleLeftInThisInd,
maxAngleRotatePerFrame - angleTraveledThisFrame
);
transform.rotation = Quaternion.RotateTowards(curRot, goalRot, curAngleRotate);
// Update how much we've rotated already. This determines
// if we get to go through the while loop again.
angleTraveledThisFrame += curAngleRotate;
if (angleTraveledThisFrame < maxAngleRotatePerFrame )
// If we have more rotating to do this frame,
// increment the index.
ind++;
if (ind==dataLen)
// If you need to do anything when you run out of rotations,
// you can do it here.
add a comment |
You (probably) don't want to apply all of the rotations inside the span of one frame regardless of rotational speed.
Rather, you should keep track of how much you are rotating during the course of working through the queue that frame, and get out of the while loop if you meet that.
public float maxAngleRotatePerFrame = 5f;
void FixedUpdate()
if(shouldUpdate) //set to true for a success response from the api call
// Keep track of how far we've traveled in this frame.
float angleTraveledThisFrame = 0f;
// Rotate until we've rotated as much as we can in a single frame,
// or we run out of rotations to do.
while (angleTraveledThisFrame < maxAngleRotatePerFrame && ind < dataLen)
// Figure out how we want to rotate and how much that is
Quaternion curRot = transform.rotation;
Quaternion goalRot = Quaternion.Euler(
batData.x[ind],
batData.y[ind],
batData.z[ind]
);
float angleLeftInThisInd = Quaternion.Angle(curRot, goalRot);
// Rotate as much as we can toward that rotation this frame
float curAngleRotate = Mathf.Min(
angleLeftInThisInd,
maxAngleRotatePerFrame - angleTraveledThisFrame
);
transform.rotation = Quaternion.RotateTowards(curRot, goalRot, curAngleRotate);
// Update how much we've rotated already. This determines
// if we get to go through the while loop again.
angleTraveledThisFrame += curAngleRotate;
if (angleTraveledThisFrame < maxAngleRotatePerFrame )
// If we have more rotating to do this frame,
// increment the index.
ind++;
if (ind==dataLen)
// If you need to do anything when you run out of rotations,
// you can do it here.
add a comment |
You (probably) don't want to apply all of the rotations inside the span of one frame regardless of rotational speed.
Rather, you should keep track of how much you are rotating during the course of working through the queue that frame, and get out of the while loop if you meet that.
public float maxAngleRotatePerFrame = 5f;
void FixedUpdate()
if(shouldUpdate) //set to true for a success response from the api call
// Keep track of how far we've traveled in this frame.
float angleTraveledThisFrame = 0f;
// Rotate until we've rotated as much as we can in a single frame,
// or we run out of rotations to do.
while (angleTraveledThisFrame < maxAngleRotatePerFrame && ind < dataLen)
// Figure out how we want to rotate and how much that is
Quaternion curRot = transform.rotation;
Quaternion goalRot = Quaternion.Euler(
batData.x[ind],
batData.y[ind],
batData.z[ind]
);
float angleLeftInThisInd = Quaternion.Angle(curRot, goalRot);
// Rotate as much as we can toward that rotation this frame
float curAngleRotate = Mathf.Min(
angleLeftInThisInd,
maxAngleRotatePerFrame - angleTraveledThisFrame
);
transform.rotation = Quaternion.RotateTowards(curRot, goalRot, curAngleRotate);
// Update how much we've rotated already. This determines
// if we get to go through the while loop again.
angleTraveledThisFrame += curAngleRotate;
if (angleTraveledThisFrame < maxAngleRotatePerFrame )
// If we have more rotating to do this frame,
// increment the index.
ind++;
if (ind==dataLen)
// If you need to do anything when you run out of rotations,
// you can do it here.
You (probably) don't want to apply all of the rotations inside the span of one frame regardless of rotational speed.
Rather, you should keep track of how much you are rotating during the course of working through the queue that frame, and get out of the while loop if you meet that.
public float maxAngleRotatePerFrame = 5f;
void FixedUpdate()
if(shouldUpdate) //set to true for a success response from the api call
// Keep track of how far we've traveled in this frame.
float angleTraveledThisFrame = 0f;
// Rotate until we've rotated as much as we can in a single frame,
// or we run out of rotations to do.
while (angleTraveledThisFrame < maxAngleRotatePerFrame && ind < dataLen)
// Figure out how we want to rotate and how much that is
Quaternion curRot = transform.rotation;
Quaternion goalRot = Quaternion.Euler(
batData.x[ind],
batData.y[ind],
batData.z[ind]
);
float angleLeftInThisInd = Quaternion.Angle(curRot, goalRot);
// Rotate as much as we can toward that rotation this frame
float curAngleRotate = Mathf.Min(
angleLeftInThisInd,
maxAngleRotatePerFrame - angleTraveledThisFrame
);
transform.rotation = Quaternion.RotateTowards(curRot, goalRot, curAngleRotate);
// Update how much we've rotated already. This determines
// if we get to go through the while loop again.
angleTraveledThisFrame += curAngleRotate;
if (angleTraveledThisFrame < maxAngleRotatePerFrame )
// If we have more rotating to do this frame,
// increment the index.
ind++;
if (ind==dataLen)
// If you need to do anything when you run out of rotations,
// you can do it here.
edited Nov 13 '18 at 23:26
answered Nov 13 '18 at 23:20
RuzihmRuzihm
3,72611628
3,72611628
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53290539%2funity3d-smoothly-rotate-an-object-based-on-a-list-of-pitch-roll-and-yaw-angles%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hXD3Av a,t M,A kSOEpOM8K6k,qH,X F