Set different websocket interceptors for different channels
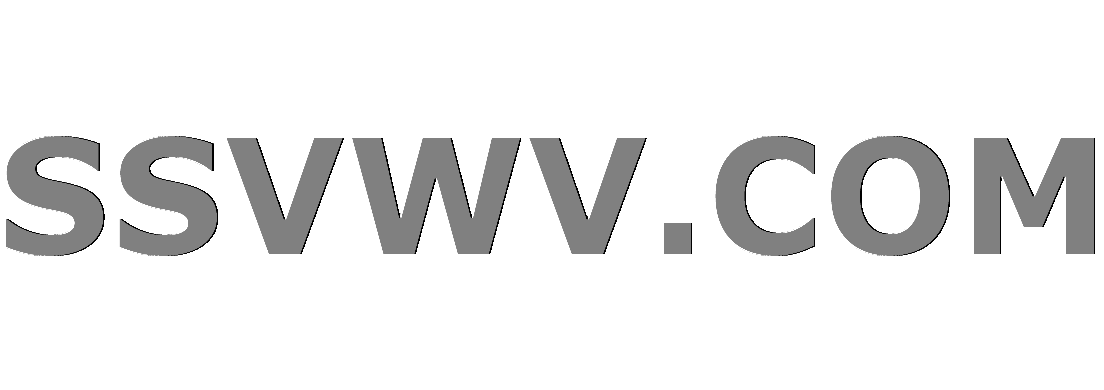
Multi tool use
up vote
0
down vote
favorite
I have the following websocket configuration in my Spring Boot app:
@Configuration
@EnableWebSocketMessageBroker
public class WebSocketConfiguration implements WebSocketMessageBrokerConfigurer
public static final String WEBSOCKET_HANDSHAKE_ENDPOINT_URI = "/api/wsocket";
public static final String QUOTE_CHANNEL_URI = "/quote";
@Autowired
private RabbitTemplate rabbitTemplate;
@Override
public void configureMessageBroker(MessageBrokerRegistry config)
config.enableSimpleBroker(QUOTE_CHANNEL_URI); /*Enable a simple in-memory broker for the clients to subscribe to channels and receive messages*/
config.setApplicationDestinationPrefixes("/app"); /*The prefix for the message mapping endpoints in the controller*/
@Override
public void registerStompEndpoints(StompEndpointRegistry registry)
/*websocket handshaking endpoint*/
registry.addEndpoint(WEBSOCKET_HANDSHAKE_ENDPOINT_URI)
/*TODO remove this after development*/
.setAllowedOrigins("http://localhost:8080");
@Override
public void configureClientInboundChannel(ChannelRegistration registeration)
registeration.interceptors(new SubscriptionInterceptor(rabbitTemplate));
and the following interceptor:
public class SubscriptionInterceptor implements ChannelInterceptor
final private SubscriptionValidator validators;
final private QuoteChannelServiceImpl quoteChannelService;
public SubscriptionInterceptor(RabbitTemplate rabbitTemplate)
this.validators = new SubscriptionValidator new QuoteSubscriptionValidator() ;
this.quoteChannelService = QuoteChannelServiceImpl.getInstance(rabbitTemplate);
@Override
public Message<?> preSend(Message<?> message, MessageChannel channel) command.equals(StompCommand.DISCONNECT))
quoteChannelService.subscriberLeft(headerAccessor.getDestination().replaceFirst(
WebSocketConfiguration.QUOTE_CHANNEL_URI.concat("/"), ""));// TODO can we move "/" into the QUOTE_CHANNEL_URI?
return message;
private void validate(Message<?> message)
StompHeaderAccessor headerAccessor = StompHeaderAccessor.wrap(message);
Arrays.stream(validators)
.filter(validator -> validator.supports(headerAccessor)
&& validator.validate(headerAccessor)).findFirst()
.orElseThrow(() -> new MessagingException(message));
I would like to know if it is possible that I have different interceptors for different brokers. I
java spring
add a comment |
up vote
0
down vote
favorite
I have the following websocket configuration in my Spring Boot app:
@Configuration
@EnableWebSocketMessageBroker
public class WebSocketConfiguration implements WebSocketMessageBrokerConfigurer
public static final String WEBSOCKET_HANDSHAKE_ENDPOINT_URI = "/api/wsocket";
public static final String QUOTE_CHANNEL_URI = "/quote";
@Autowired
private RabbitTemplate rabbitTemplate;
@Override
public void configureMessageBroker(MessageBrokerRegistry config)
config.enableSimpleBroker(QUOTE_CHANNEL_URI); /*Enable a simple in-memory broker for the clients to subscribe to channels and receive messages*/
config.setApplicationDestinationPrefixes("/app"); /*The prefix for the message mapping endpoints in the controller*/
@Override
public void registerStompEndpoints(StompEndpointRegistry registry)
/*websocket handshaking endpoint*/
registry.addEndpoint(WEBSOCKET_HANDSHAKE_ENDPOINT_URI)
/*TODO remove this after development*/
.setAllowedOrigins("http://localhost:8080");
@Override
public void configureClientInboundChannel(ChannelRegistration registeration)
registeration.interceptors(new SubscriptionInterceptor(rabbitTemplate));
and the following interceptor:
public class SubscriptionInterceptor implements ChannelInterceptor
final private SubscriptionValidator validators;
final private QuoteChannelServiceImpl quoteChannelService;
public SubscriptionInterceptor(RabbitTemplate rabbitTemplate)
this.validators = new SubscriptionValidator new QuoteSubscriptionValidator() ;
this.quoteChannelService = QuoteChannelServiceImpl.getInstance(rabbitTemplate);
@Override
public Message<?> preSend(Message<?> message, MessageChannel channel) command.equals(StompCommand.DISCONNECT))
quoteChannelService.subscriberLeft(headerAccessor.getDestination().replaceFirst(
WebSocketConfiguration.QUOTE_CHANNEL_URI.concat("/"), ""));// TODO can we move "/" into the QUOTE_CHANNEL_URI?
return message;
private void validate(Message<?> message)
StompHeaderAccessor headerAccessor = StompHeaderAccessor.wrap(message);
Arrays.stream(validators)
.filter(validator -> validator.supports(headerAccessor)
&& validator.validate(headerAccessor)).findFirst()
.orElseThrow(() -> new MessagingException(message));
I would like to know if it is possible that I have different interceptors for different brokers. I
java spring
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have the following websocket configuration in my Spring Boot app:
@Configuration
@EnableWebSocketMessageBroker
public class WebSocketConfiguration implements WebSocketMessageBrokerConfigurer
public static final String WEBSOCKET_HANDSHAKE_ENDPOINT_URI = "/api/wsocket";
public static final String QUOTE_CHANNEL_URI = "/quote";
@Autowired
private RabbitTemplate rabbitTemplate;
@Override
public void configureMessageBroker(MessageBrokerRegistry config)
config.enableSimpleBroker(QUOTE_CHANNEL_URI); /*Enable a simple in-memory broker for the clients to subscribe to channels and receive messages*/
config.setApplicationDestinationPrefixes("/app"); /*The prefix for the message mapping endpoints in the controller*/
@Override
public void registerStompEndpoints(StompEndpointRegistry registry)
/*websocket handshaking endpoint*/
registry.addEndpoint(WEBSOCKET_HANDSHAKE_ENDPOINT_URI)
/*TODO remove this after development*/
.setAllowedOrigins("http://localhost:8080");
@Override
public void configureClientInboundChannel(ChannelRegistration registeration)
registeration.interceptors(new SubscriptionInterceptor(rabbitTemplate));
and the following interceptor:
public class SubscriptionInterceptor implements ChannelInterceptor
final private SubscriptionValidator validators;
final private QuoteChannelServiceImpl quoteChannelService;
public SubscriptionInterceptor(RabbitTemplate rabbitTemplate)
this.validators = new SubscriptionValidator new QuoteSubscriptionValidator() ;
this.quoteChannelService = QuoteChannelServiceImpl.getInstance(rabbitTemplate);
@Override
public Message<?> preSend(Message<?> message, MessageChannel channel) command.equals(StompCommand.DISCONNECT))
quoteChannelService.subscriberLeft(headerAccessor.getDestination().replaceFirst(
WebSocketConfiguration.QUOTE_CHANNEL_URI.concat("/"), ""));// TODO can we move "/" into the QUOTE_CHANNEL_URI?
return message;
private void validate(Message<?> message)
StompHeaderAccessor headerAccessor = StompHeaderAccessor.wrap(message);
Arrays.stream(validators)
.filter(validator -> validator.supports(headerAccessor)
&& validator.validate(headerAccessor)).findFirst()
.orElseThrow(() -> new MessagingException(message));
I would like to know if it is possible that I have different interceptors for different brokers. I
java spring
I have the following websocket configuration in my Spring Boot app:
@Configuration
@EnableWebSocketMessageBroker
public class WebSocketConfiguration implements WebSocketMessageBrokerConfigurer
public static final String WEBSOCKET_HANDSHAKE_ENDPOINT_URI = "/api/wsocket";
public static final String QUOTE_CHANNEL_URI = "/quote";
@Autowired
private RabbitTemplate rabbitTemplate;
@Override
public void configureMessageBroker(MessageBrokerRegistry config)
config.enableSimpleBroker(QUOTE_CHANNEL_URI); /*Enable a simple in-memory broker for the clients to subscribe to channels and receive messages*/
config.setApplicationDestinationPrefixes("/app"); /*The prefix for the message mapping endpoints in the controller*/
@Override
public void registerStompEndpoints(StompEndpointRegistry registry)
/*websocket handshaking endpoint*/
registry.addEndpoint(WEBSOCKET_HANDSHAKE_ENDPOINT_URI)
/*TODO remove this after development*/
.setAllowedOrigins("http://localhost:8080");
@Override
public void configureClientInboundChannel(ChannelRegistration registeration)
registeration.interceptors(new SubscriptionInterceptor(rabbitTemplate));
and the following interceptor:
public class SubscriptionInterceptor implements ChannelInterceptor
final private SubscriptionValidator validators;
final private QuoteChannelServiceImpl quoteChannelService;
public SubscriptionInterceptor(RabbitTemplate rabbitTemplate)
this.validators = new SubscriptionValidator new QuoteSubscriptionValidator() ;
this.quoteChannelService = QuoteChannelServiceImpl.getInstance(rabbitTemplate);
@Override
public Message<?> preSend(Message<?> message, MessageChannel channel) command.equals(StompCommand.DISCONNECT))
quoteChannelService.subscriberLeft(headerAccessor.getDestination().replaceFirst(
WebSocketConfiguration.QUOTE_CHANNEL_URI.concat("/"), ""));// TODO can we move "/" into the QUOTE_CHANNEL_URI?
return message;
private void validate(Message<?> message)
StompHeaderAccessor headerAccessor = StompHeaderAccessor.wrap(message);
Arrays.stream(validators)
.filter(validator -> validator.supports(headerAccessor)
&& validator.validate(headerAccessor)).findFirst()
.orElseThrow(() -> new MessagingException(message));
I would like to know if it is possible that I have different interceptors for different brokers. I
java spring
java spring
asked Nov 9 at 2:43


Arian Hosseinzadeh
1,43143785
1,43143785
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53219107%2fset-different-websocket-interceptors-for-different-channels%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Rb,y64Ym Ya,EjIbJ dJCQ,Sw6712oTtA,dU