Restructure a JSON and output to another file
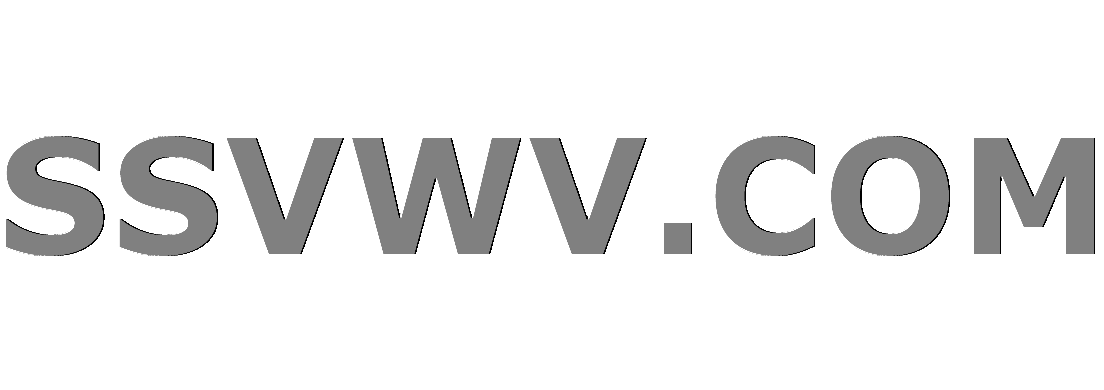
Multi tool use
Restructure a JSON and output to another file
I'm getting a very long list of one level json data that look something like this:
json-old.json
[
"stock": "abc", "volume": "45434", "price": "31", "date": "10/12/12",
"stock": "abc", "volume": "45435", "price": "30", "date": "10/13/12",
"stock": "xyz", "volume": "34465", "price": "14", "date": "10/12/12",
"stock": "xyz", "volume": "34434", "price": "14", "date": "10/13/12",
"stock": "zzz", "volume": "76755", "price": "65", "date": "10/12/12",
"stock": "zzz", "volume": "85646", "price": "67", "date": "10/13/12"
]
how can I take that above file and output it to a new file:
json-new.json
[
"abc":
"10/12/12": "volume": "45434", "price": "31" ,
"10/13/12": "volume": "45435", "price": "30"
,
"xyz":
"10/12/12": "volume": "34465", "price": "14" ,
"10/13/12": "volume": "34434", "price": "14"
,
"zzz":
"10/12/12": "volume": "76755", "price": "65" ,
"10/13/12": "volume": "85646", "price": "67"
]
Basically I want to organize and nest the data under the 'stock' property, and then nest the remaining data under 'date' property.
Is there a library I can use to automate this process in javascript or nodejs? I have a lot of this files inside a folder and want to output it to single file with the desired output (so that I can upload to a database like firebase).
Hi @FarooqKhan, to be perfectly honest, I don't even know where to start. I'm only a beginner in javascript and suddenly I get this task. I just want someone to point me in the right direction, and look and try to solve from there.
– Gab Shirohige
Sep 16 '18 at 12:57
Ok as a push, you should be grouping your data based on
stock
and date
to get your desired result.– Farooq Khan
Sep 16 '18 at 13:00
stock
date
lodash provides some useful functions, please read its docs.
– Farooq Khan
Sep 16 '18 at 13:01
3 Answers
3
You can use Array.reduce
const s = [
"stock": "abc", "volume": "45434", "price": "31", "date": "10/12/12" ,
"stock": "abc", "volume": "45435", "price": "30", "date": "10/13/12" ,
"stock": "xyz", "volume": "34465", "price": "14", "date": "10/12/12" ,
"stock": "xyz", "volume": "34434", "price": "14", "date": "10/13/12" ,
"stock": "zzz", "volume": "76755", "price": "65", "date": "10/12/12" ,
"stock": "zzz", "volume": "85646", "price": "67", "date": "10/13/12"
]
const g = s.reduce((ret, o) => , )
console.log([g])
Thank you. Clean and concise solution! Now I'll look for a way to accept the initial data from multiple files and output it to one file. Thanks!
– Gab Shirohige
Sep 16 '18 at 15:40
you can use for loop as follow.
var arr = [
"stock": "abc", "volume": "45434", "price": "31", "date": "10/12/12" ,
"stock": "abc", "volume": "45435", "price": "30", "date": "10/13/12" ,
"stock": "xyz", "volume": "34465", "price": "14", "date": "10/12/12" ,
"stock": "xyz", "volume": "34434", "price": "14", "date": "10/13/12" ,
"stock": "zzz", "volume": "76755", "price": "65", "date": "10/12/12" ,
"stock": "zzz", "volume": "85646", "price": "67", "date": "10/13/12"
];
var group = ;
var json =
for (var item in arr)
;
stock[itm.date] = date;
group[itm.stock] = stock;
for (i in group)
var obj =
obj[i] = group[i];
json.push(obj)
console.log(json);
// console.log(JSON.stringify(json));
and you use json as new format
This should do it:
const fs = require('fs')
const groupBy = (items, key) => items.reduce(
(result, item) => ( ),
item,
],
),
,
);
const inFileName = process.argv[2]
const outFileName = process.argv[3]
const json = JSON.parse(fs.readFileSync(inFileName, 'utf8'));
const groupedStock = groupBy(json,'stock')
const groupedDate =
for(s in groupedStock)
groupedDate[s] = groupBy(groupedStock[s],'date')
const filtered =
for(s in groupedDate)
filtered[s] =
for (d in groupedDate[s])
filtered[s][d] =
price: groupedDate[s][d][0].price,
volume: groupedDate[s][d][0].volume
const res =
for(s in filtered)
res.push(
[s]: filtered[s]
)
fs.writeFileSync(outFileName, JSON.stringify(res,null,2));
Run the program using node convert.js json-old.json json-new.json
node convert.js json-old.json json-new.json
Hey thanks man. This achieved what I was looking for. I couldn't ask you for more, but I still need to update this code to accept multiple files from a folder then output it to one file. I'll study more about the node file system module that you used here. Thanks!
– Gab Shirohige
Sep 16 '18 at 15:32
Thanks for contributing an answer to Stack Overflow!
But avoid …
To learn more, see our tips on writing great answers.
Required, but never shown
Required, but never shown
By clicking "Post Your Answer", you agree to our terms of service, privacy policy and cookie policy
Hi Gab, please show what you have tried so far.
– Farooq Khan
Sep 16 '18 at 12:48