Index out of range while accessing index of the next iteration in loop in python
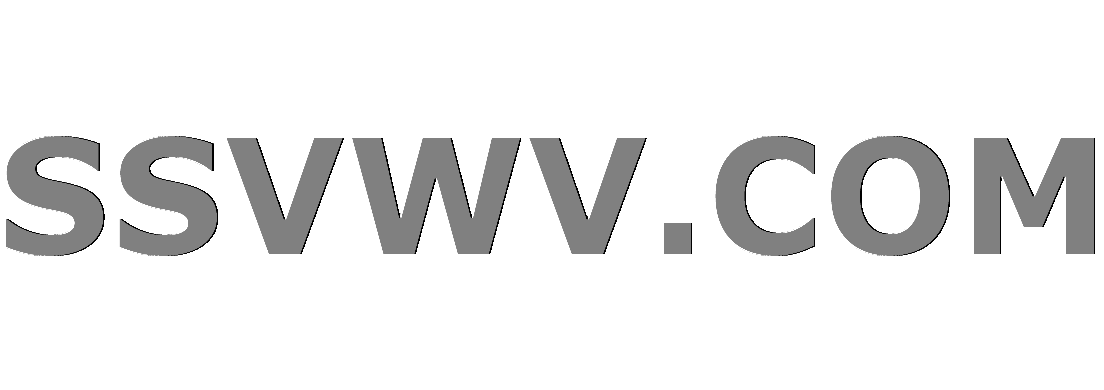
Multi tool use
Index out of range while accessing index of the next iteration in loop in python
There are several image files in a directory on which I need to iterate through, where need to access the next iteration value in the current iteration.
img_files_list = os.listdir(/path/to/directory)
new_list =
for index, img in enumerate(img_files_list):
if img in new_list:
continue
new_list.extend([img, img_files_list[index + 1], img_files_list[index + 2]])
print(img)
print(img_files_list[index + 1])
print(img_files_list[index + 2])
I need to iterate over all the items of the img_files_list but when reached at the end of the loop need to properly come out of loop without index out of range exception. Anyone, please point out where do I missed the logic.
index + 1
index + 2
img_files_list[10]
img_files_list[11]
Why do you need index+2? Because cause of error is it. For ex., if you have 2 element in list, index values will be 0, 1. And for first element, index+1 is 1. But index+2 is 2 and it is out of range.
– kur ag
Sep 16 '18 at 12:25
You will add only one if line after for line. if index < (len(img_files_list) - 2) :
– kur ag
Sep 16 '18 at 12:54
2 Answers
2
Seems more like Code Review than Stack Overflow.
When you reach the last item in your for image, img in enumerate(img_files_list)
line, there is no item after the last one. This is what causes an IndexOutOfRangeException
.
for image, img in enumerate(img_files_list)
IndexOutOfRangeException
There are a few ways to approach this:
As Sruthi V. wrote, include a condition:
print(img_files_list[i])
if i + 1 < len(img_files_list):
print(img_files_list[i + 1])
if i + 2 < len(img_files_list):
print(img_files_list[i + 2])
Include a try... except
:
try... except
try:
print(img_files_list[i])
print(img_files_list[i + 1])
print(img_files_list[i + 2])
except IndexOutOfRangeException:
pass
Limit the range of your loop:
i_img = list(enumerate(['a', 'b', 'c', 'd', 'e']))
for i, img in i_img [:len(i_img ) - 2]:
print(img)
print(i_img[i + 1])
print(i_img[i + 2])
Note that you have no reason to use enumerate
— you're just using it to get indices. You also don't need your if img in new_list
— you're just using it to skip the next two. You can make this more elegant and solve your problem using range (a modified solution 3).
enumerate
if img in new_list
imgs = os.listdir('/path/to/directory')
triplets =
for i in range(0, len(imgs) - 2, 3):
triplet = [imgs[i], imgs[i + 1], imgs[i + 2]]
triplets.extend(triplet)
print('n'.join(triplet))
N.B. I'm not sure what you're even trying to do. Even your if img in new_list
might be a mistake since it just skips the last 1-2 final items. And if that were gone, all you would be doing is making a copy of this list and printing its contents. In which case I would suggest:
if img in new_list
imgs = os.listdir('/path/to/directory')
print('n'.join(imgs))
But if you clarify what you're trying to do, I can edit this answer.
From my comment to your question:
index + 1
and index + 2
cause a problem for last 2 items. For
example, if your list's size is 10, then indexes will be 0,2,...,9.
When the index is 8 and 9, you are trying to execute
img_files_list[10]
and img_files_list[11]
. So the index is out of
range.
index + 1
index + 2
img_files_list[10]
img_files_list[11]
I assume that you want to access images as 3-item list, then I suggest you a little bit different way. I will use an integer array instead of images for a better understanding.
img_files_list = [0,1,2,3,4,5,6,7,8,9]
new_list =
n = 3
for i in range(0, len(img_files_list), n): # Respectively, i is 0,3,6,9,...
temp = img_files_list[i:i+n]
print(temp)
new_list.extend(temp)
Output:
[0, 1, 2]
[3, 4, 5]
[6, 7, 8]
[9]
Thanks for contributing an answer to Stack Overflow!
But avoid …
To learn more, see our tips on writing great answers.
Required, but never shown
Required, but never shown
By clicking "Post Your Answer", you agree to our terms of service, privacy policy and cookie policy
index + 1
andindex + 2
cause a problem for last 2 items. For example, if your list's size is 10, then indexes will be 0,2,...,9. When the index is 8 and 9, you are trying to executeimg_files_list[10]
andimg_files_list[11]
. So the index is out of range.– Alperen
Sep 16 '18 at 12:24