How strings are compared to each other?
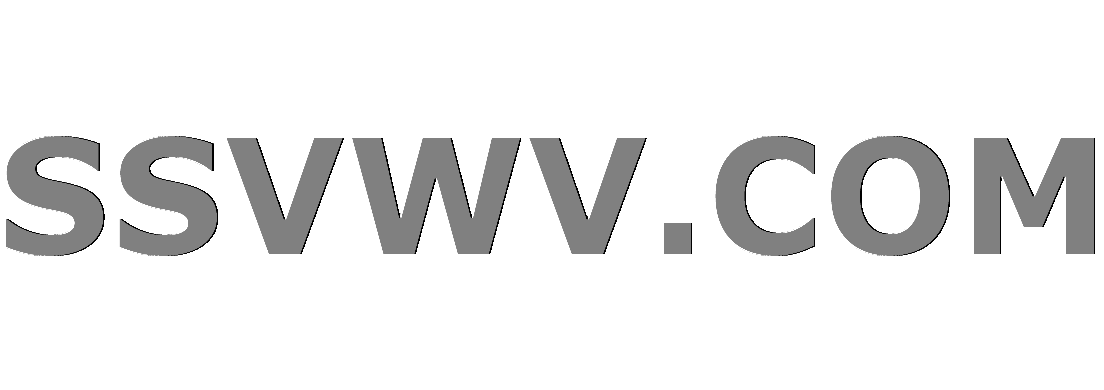
Multi tool use
How strings are compared to each other?
When comparing 2 strings, what does actually happen? How do we determine that content inside a String A is equal to String B? Do we look in unicode character table for element to element comparision of both Strings? if yes, then how does equals() know about element in a String like "ABC", how does equal() sperates A from BC to compare it to first element of other string ?
@JBNizet okay, but how does it do that? I looked at equals(), i dont actually understand it.
– calculusnoob
Sep 13 '18 at 22:25
Well, post the code you looked at (it varies depending on the Java version), and tell us what you don't understand in that code. But basically, it compares the length of both strings, and then compare the characters, one by one.
– JB Nizet
Sep 13 '18 at 22:27
FYI, link to source code of
String
in OpenJDK for Java 10.– Basil Bourque
Sep 13 '18 at 22:58
String
2 Answers
2
Strings are basically immutable wrappers around a char
, with a lot of useful utility methods.
char
So, to check if two strings are equal, you simply check if they both have char
arrays of equal size and with equal content.
char
Using the Arrays
class, which has useful methods for working with arrays, a simplified String
implementation might look like this:
Arrays
String
public final class String
private char value;
public String(char value)
this.value = value.clone();
@Override
public boolean equals(Object obj)
if (! (obj instanceof String))
return false;
String other = (String) obj;
return Arrays.equals(this.value, other.value);
@Override
public int hashCode()
return Arrays.hashCode(this.value);
public char toCharArray()
return this.value.clone();
public String substring(int beginIndex, int endIndex)
return new String(Arrays.copyOfRange(this.value, beginIndex, endIndex));
// more utility methods
The real String
class is more complicated, in different ways depending on the Java version.
String
E.g. in earlier versions, the char
could be shared by multiple String
objects, so e.g. substring()
wouldn't need to copy the array.
char
String
substring()
In later versions, optimizations have changed to store characters in byte
(LATIN1) arrays if possible, instead of char
(UTF-16) arrays, in order to save space.
byte
char
These various optimizations will of course complicate the internal code of the String
utility methods, which might be what is confusing you when you look at the JDK source code.
String
Somewhat unrelated note: the sharing of a single char was actually a premature optimization. They thought it would optimize some String operations to share the same array and specify a start and end point so string.substring wouldn't create an entirely new string but they didn't take into consideration the idea that web developers would download an entire web page and split/filter out a few words, expecting the original string to be collected. Removing the shared backing array was more of a de-optimization :)
– Bill K
Sep 13 '18 at 22:53
When in Doubt always check the JavaDocs:
Compares this string to the specified object. The result is true if
and only if the argument is not null and is a String object that
represents the same sequence of characters as this object.
When you use equals with Strings this is actually happens
public boolean equals(Object anObject)
if (this == anObject)
return true;
if (anObject instanceof String)
String anotherString = (String)anObject;
int n = value.length;
if (n == anotherString.value.length)
char v1 = value;
char v2 = anotherString.value;
int i = 0;
while (n-- != 0)
if (v1[i] != v2[i])
return false;
i++;
return true;
return false;
So it will return only if you are comparing other String if you have the same sequence because it is comparing Characters.
Thanks for contributing an answer to Stack Overflow!
But avoid …
To learn more, see our tips on writing great answers.
Required, but never shown
Required, but never shown
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
The compiler has no idea. The equals() method of the String class does that, at runtime. Not the compiler. The compiler transforms source code into bytecode. It doesn't execute the code.
– JB Nizet
Sep 13 '18 at 22:23