Haskell code prints out a list for ints but not for chars
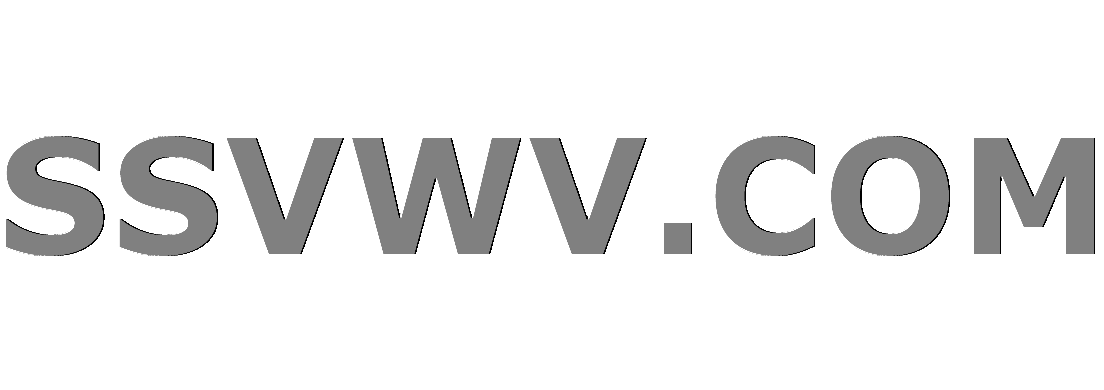
Multi tool use
My code currently looks like this. It is supposed to show the possible first symbols in the regular expression definition given to us beforehand. I am supposed to print these out as a list. For example, if the answer is supposed to be [1,2], it will come out [1,2] but when the answer is supposed to be ['1','2'] it will come out "12" or when it is supposed to be ['a', 'b'] it will come out "ab". What am I doing wrong?
data RE a -- regular expressions over an alphabet defined by 'a'
= Empty -- empty regular expression
| Sym a -- match the given symbol
| RE a :+: RE a -- concatenation of two regular expressions
| RE a :|: RE a -- choice between two regular expressions
| Rep (RE a) -- zero or more repetitions of a regular expression
| Rep1 (RE a) -- one or more repetitions of a regular expression
deriving (Show)
firstMatches :: RE a -> [a]
firstMatches Empty =
firstMatches (Sym a)= a:list
firstMatches(Rep(a))=firstMatches a
firstMatches(Rep1(a))=firstMatches a
firstMatches (Empty :+: b)= firstMatches b
firstMatches (a :+: _) = firstMatches a
firstMatches (a :|: b)= firstMatches a ++ firstMatches b
haskell
add a comment |
My code currently looks like this. It is supposed to show the possible first symbols in the regular expression definition given to us beforehand. I am supposed to print these out as a list. For example, if the answer is supposed to be [1,2], it will come out [1,2] but when the answer is supposed to be ['1','2'] it will come out "12" or when it is supposed to be ['a', 'b'] it will come out "ab". What am I doing wrong?
data RE a -- regular expressions over an alphabet defined by 'a'
= Empty -- empty regular expression
| Sym a -- match the given symbol
| RE a :+: RE a -- concatenation of two regular expressions
| RE a :|: RE a -- choice between two regular expressions
| Rep (RE a) -- zero or more repetitions of a regular expression
| Rep1 (RE a) -- one or more repetitions of a regular expression
deriving (Show)
firstMatches :: RE a -> [a]
firstMatches Empty =
firstMatches (Sym a)= a:list
firstMatches(Rep(a))=firstMatches a
firstMatches(Rep1(a))=firstMatches a
firstMatches (Empty :+: b)= firstMatches b
firstMatches (a :+: _) = firstMatches a
firstMatches (a :|: b)= firstMatches a ++ firstMatches b
haskell
Are you supposed to print it in a list format, or just return a list? What are the requirements specifically?
– DarthFennec
Nov 10 at 0:30
That's what's theShow
instance for lists does. If you want some other behavior, don't useshow
.
– Carl
Nov 10 at 0:41
add a comment |
My code currently looks like this. It is supposed to show the possible first symbols in the regular expression definition given to us beforehand. I am supposed to print these out as a list. For example, if the answer is supposed to be [1,2], it will come out [1,2] but when the answer is supposed to be ['1','2'] it will come out "12" or when it is supposed to be ['a', 'b'] it will come out "ab". What am I doing wrong?
data RE a -- regular expressions over an alphabet defined by 'a'
= Empty -- empty regular expression
| Sym a -- match the given symbol
| RE a :+: RE a -- concatenation of two regular expressions
| RE a :|: RE a -- choice between two regular expressions
| Rep (RE a) -- zero or more repetitions of a regular expression
| Rep1 (RE a) -- one or more repetitions of a regular expression
deriving (Show)
firstMatches :: RE a -> [a]
firstMatches Empty =
firstMatches (Sym a)= a:list
firstMatches(Rep(a))=firstMatches a
firstMatches(Rep1(a))=firstMatches a
firstMatches (Empty :+: b)= firstMatches b
firstMatches (a :+: _) = firstMatches a
firstMatches (a :|: b)= firstMatches a ++ firstMatches b
haskell
My code currently looks like this. It is supposed to show the possible first symbols in the regular expression definition given to us beforehand. I am supposed to print these out as a list. For example, if the answer is supposed to be [1,2], it will come out [1,2] but when the answer is supposed to be ['1','2'] it will come out "12" or when it is supposed to be ['a', 'b'] it will come out "ab". What am I doing wrong?
data RE a -- regular expressions over an alphabet defined by 'a'
= Empty -- empty regular expression
| Sym a -- match the given symbol
| RE a :+: RE a -- concatenation of two regular expressions
| RE a :|: RE a -- choice between two regular expressions
| Rep (RE a) -- zero or more repetitions of a regular expression
| Rep1 (RE a) -- one or more repetitions of a regular expression
deriving (Show)
firstMatches :: RE a -> [a]
firstMatches Empty =
firstMatches (Sym a)= a:list
firstMatches(Rep(a))=firstMatches a
firstMatches(Rep1(a))=firstMatches a
firstMatches (Empty :+: b)= firstMatches b
firstMatches (a :+: _) = firstMatches a
firstMatches (a :|: b)= firstMatches a ++ firstMatches b
haskell
haskell
asked Nov 10 at 0:20
Aditya Sirohi
61
61
Are you supposed to print it in a list format, or just return a list? What are the requirements specifically?
– DarthFennec
Nov 10 at 0:30
That's what's theShow
instance for lists does. If you want some other behavior, don't useshow
.
– Carl
Nov 10 at 0:41
add a comment |
Are you supposed to print it in a list format, or just return a list? What are the requirements specifically?
– DarthFennec
Nov 10 at 0:30
That's what's theShow
instance for lists does. If you want some other behavior, don't useshow
.
– Carl
Nov 10 at 0:41
Are you supposed to print it in a list format, or just return a list? What are the requirements specifically?
– DarthFennec
Nov 10 at 0:30
Are you supposed to print it in a list format, or just return a list? What are the requirements specifically?
– DarthFennec
Nov 10 at 0:30
That's what's the
Show
instance for lists does. If you want some other behavior, don't use show
.– Carl
Nov 10 at 0:41
That's what's the
Show
instance for lists does. If you want some other behavior, don't use show
.– Carl
Nov 10 at 0:41
add a comment |
1 Answer
1
active
oldest
votes
You're not doing anything wrong.
String
is a type synonym for [Char]
, so if you try to print a [Char]
it will print as a String
. This is somewhat of a special case, and it can be a little weird.
Show
is the typeclass used to print things as a string. The definition of Show
is something like this:
class Show a where
showsPrec :: Int -> a -> ShowS
show :: a -> String
showList :: [a] -> ShowS
The showList
function is optional. The documentation states:
The method
showList
is provided to allow the programmer to give a specialised way of showing lists of values. For example, this is used by the predefinedShow
instance of theChar
type, where values of typeString
should be shown in double quotes, rather than between square brackets.
So if you define a new type and instantiate Show
, you can optionally define a special way to show
a list of your type, separate from the way it's normally shown and separate from the way lists are normally shown. Char
takes advantage of this, in that a [Char]
(or equivalently, a String
), is shown with double-quotes instead of as a list of Char
values.
I can't think of a way to get it to use the default show
for a [Char]
. I don't think there is one. A workaround might be to create a newtype
wrapping Char
with its own Show
that uses the default showList
implementation, but that doesn't seem appropriate here.
If this is homework, I'd expect the grader to know about this already, and I seriously doubt you'd get marked down for it, especially since the problem doesn't appear to be about show
at all.
okay. so it turns out the teacher doesn't care if it shows up as a string because as you said, a string is just a list of chars
– Aditya Sirohi
Nov 10 at 1:46
@AdityaSirohi: Just for your own reference, thenewtype
solution DarthFennec mentions is:newtype C = C Char; instance Show C where show (C c) = show c
. Thenshow (map C "abc")
will produce the string"['a', 'b', 'c']"
as expected. This is also a good opportunity to useData.Coerce.coerce
, which converts safely between a type and anewtype
wrapper, with no runtime cost, e.g.coerce "abc" :: [C]
.
– Jon Purdy
Nov 10 at 7:15
Slight quibble:show
doesn't print anything; it returns a string.
– chepner
Nov 10 at 14:31
@chepner,show
doesn't "return" anything, it evaluates to a string. >:)
– luqui
Nov 11 at 20:24
That's an even bigger quibble than my original quibble :)
– chepner
Nov 11 at 20:31
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53234906%2fhaskell-code-prints-out-a-list-for-ints-but-not-for-chars%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You're not doing anything wrong.
String
is a type synonym for [Char]
, so if you try to print a [Char]
it will print as a String
. This is somewhat of a special case, and it can be a little weird.
Show
is the typeclass used to print things as a string. The definition of Show
is something like this:
class Show a where
showsPrec :: Int -> a -> ShowS
show :: a -> String
showList :: [a] -> ShowS
The showList
function is optional. The documentation states:
The method
showList
is provided to allow the programmer to give a specialised way of showing lists of values. For example, this is used by the predefinedShow
instance of theChar
type, where values of typeString
should be shown in double quotes, rather than between square brackets.
So if you define a new type and instantiate Show
, you can optionally define a special way to show
a list of your type, separate from the way it's normally shown and separate from the way lists are normally shown. Char
takes advantage of this, in that a [Char]
(or equivalently, a String
), is shown with double-quotes instead of as a list of Char
values.
I can't think of a way to get it to use the default show
for a [Char]
. I don't think there is one. A workaround might be to create a newtype
wrapping Char
with its own Show
that uses the default showList
implementation, but that doesn't seem appropriate here.
If this is homework, I'd expect the grader to know about this already, and I seriously doubt you'd get marked down for it, especially since the problem doesn't appear to be about show
at all.
okay. so it turns out the teacher doesn't care if it shows up as a string because as you said, a string is just a list of chars
– Aditya Sirohi
Nov 10 at 1:46
@AdityaSirohi: Just for your own reference, thenewtype
solution DarthFennec mentions is:newtype C = C Char; instance Show C where show (C c) = show c
. Thenshow (map C "abc")
will produce the string"['a', 'b', 'c']"
as expected. This is also a good opportunity to useData.Coerce.coerce
, which converts safely between a type and anewtype
wrapper, with no runtime cost, e.g.coerce "abc" :: [C]
.
– Jon Purdy
Nov 10 at 7:15
Slight quibble:show
doesn't print anything; it returns a string.
– chepner
Nov 10 at 14:31
@chepner,show
doesn't "return" anything, it evaluates to a string. >:)
– luqui
Nov 11 at 20:24
That's an even bigger quibble than my original quibble :)
– chepner
Nov 11 at 20:31
|
show 1 more comment
You're not doing anything wrong.
String
is a type synonym for [Char]
, so if you try to print a [Char]
it will print as a String
. This is somewhat of a special case, and it can be a little weird.
Show
is the typeclass used to print things as a string. The definition of Show
is something like this:
class Show a where
showsPrec :: Int -> a -> ShowS
show :: a -> String
showList :: [a] -> ShowS
The showList
function is optional. The documentation states:
The method
showList
is provided to allow the programmer to give a specialised way of showing lists of values. For example, this is used by the predefinedShow
instance of theChar
type, where values of typeString
should be shown in double quotes, rather than between square brackets.
So if you define a new type and instantiate Show
, you can optionally define a special way to show
a list of your type, separate from the way it's normally shown and separate from the way lists are normally shown. Char
takes advantage of this, in that a [Char]
(or equivalently, a String
), is shown with double-quotes instead of as a list of Char
values.
I can't think of a way to get it to use the default show
for a [Char]
. I don't think there is one. A workaround might be to create a newtype
wrapping Char
with its own Show
that uses the default showList
implementation, but that doesn't seem appropriate here.
If this is homework, I'd expect the grader to know about this already, and I seriously doubt you'd get marked down for it, especially since the problem doesn't appear to be about show
at all.
okay. so it turns out the teacher doesn't care if it shows up as a string because as you said, a string is just a list of chars
– Aditya Sirohi
Nov 10 at 1:46
@AdityaSirohi: Just for your own reference, thenewtype
solution DarthFennec mentions is:newtype C = C Char; instance Show C where show (C c) = show c
. Thenshow (map C "abc")
will produce the string"['a', 'b', 'c']"
as expected. This is also a good opportunity to useData.Coerce.coerce
, which converts safely between a type and anewtype
wrapper, with no runtime cost, e.g.coerce "abc" :: [C]
.
– Jon Purdy
Nov 10 at 7:15
Slight quibble:show
doesn't print anything; it returns a string.
– chepner
Nov 10 at 14:31
@chepner,show
doesn't "return" anything, it evaluates to a string. >:)
– luqui
Nov 11 at 20:24
That's an even bigger quibble than my original quibble :)
– chepner
Nov 11 at 20:31
|
show 1 more comment
You're not doing anything wrong.
String
is a type synonym for [Char]
, so if you try to print a [Char]
it will print as a String
. This is somewhat of a special case, and it can be a little weird.
Show
is the typeclass used to print things as a string. The definition of Show
is something like this:
class Show a where
showsPrec :: Int -> a -> ShowS
show :: a -> String
showList :: [a] -> ShowS
The showList
function is optional. The documentation states:
The method
showList
is provided to allow the programmer to give a specialised way of showing lists of values. For example, this is used by the predefinedShow
instance of theChar
type, where values of typeString
should be shown in double quotes, rather than between square brackets.
So if you define a new type and instantiate Show
, you can optionally define a special way to show
a list of your type, separate from the way it's normally shown and separate from the way lists are normally shown. Char
takes advantage of this, in that a [Char]
(or equivalently, a String
), is shown with double-quotes instead of as a list of Char
values.
I can't think of a way to get it to use the default show
for a [Char]
. I don't think there is one. A workaround might be to create a newtype
wrapping Char
with its own Show
that uses the default showList
implementation, but that doesn't seem appropriate here.
If this is homework, I'd expect the grader to know about this already, and I seriously doubt you'd get marked down for it, especially since the problem doesn't appear to be about show
at all.
You're not doing anything wrong.
String
is a type synonym for [Char]
, so if you try to print a [Char]
it will print as a String
. This is somewhat of a special case, and it can be a little weird.
Show
is the typeclass used to print things as a string. The definition of Show
is something like this:
class Show a where
showsPrec :: Int -> a -> ShowS
show :: a -> String
showList :: [a] -> ShowS
The showList
function is optional. The documentation states:
The method
showList
is provided to allow the programmer to give a specialised way of showing lists of values. For example, this is used by the predefinedShow
instance of theChar
type, where values of typeString
should be shown in double quotes, rather than between square brackets.
So if you define a new type and instantiate Show
, you can optionally define a special way to show
a list of your type, separate from the way it's normally shown and separate from the way lists are normally shown. Char
takes advantage of this, in that a [Char]
(or equivalently, a String
), is shown with double-quotes instead of as a list of Char
values.
I can't think of a way to get it to use the default show
for a [Char]
. I don't think there is one. A workaround might be to create a newtype
wrapping Char
with its own Show
that uses the default showList
implementation, but that doesn't seem appropriate here.
If this is homework, I'd expect the grader to know about this already, and I seriously doubt you'd get marked down for it, especially since the problem doesn't appear to be about show
at all.
answered Nov 10 at 0:47


DarthFennec
1,291711
1,291711
okay. so it turns out the teacher doesn't care if it shows up as a string because as you said, a string is just a list of chars
– Aditya Sirohi
Nov 10 at 1:46
@AdityaSirohi: Just for your own reference, thenewtype
solution DarthFennec mentions is:newtype C = C Char; instance Show C where show (C c) = show c
. Thenshow (map C "abc")
will produce the string"['a', 'b', 'c']"
as expected. This is also a good opportunity to useData.Coerce.coerce
, which converts safely between a type and anewtype
wrapper, with no runtime cost, e.g.coerce "abc" :: [C]
.
– Jon Purdy
Nov 10 at 7:15
Slight quibble:show
doesn't print anything; it returns a string.
– chepner
Nov 10 at 14:31
@chepner,show
doesn't "return" anything, it evaluates to a string. >:)
– luqui
Nov 11 at 20:24
That's an even bigger quibble than my original quibble :)
– chepner
Nov 11 at 20:31
|
show 1 more comment
okay. so it turns out the teacher doesn't care if it shows up as a string because as you said, a string is just a list of chars
– Aditya Sirohi
Nov 10 at 1:46
@AdityaSirohi: Just for your own reference, thenewtype
solution DarthFennec mentions is:newtype C = C Char; instance Show C where show (C c) = show c
. Thenshow (map C "abc")
will produce the string"['a', 'b', 'c']"
as expected. This is also a good opportunity to useData.Coerce.coerce
, which converts safely between a type and anewtype
wrapper, with no runtime cost, e.g.coerce "abc" :: [C]
.
– Jon Purdy
Nov 10 at 7:15
Slight quibble:show
doesn't print anything; it returns a string.
– chepner
Nov 10 at 14:31
@chepner,show
doesn't "return" anything, it evaluates to a string. >:)
– luqui
Nov 11 at 20:24
That's an even bigger quibble than my original quibble :)
– chepner
Nov 11 at 20:31
okay. so it turns out the teacher doesn't care if it shows up as a string because as you said, a string is just a list of chars
– Aditya Sirohi
Nov 10 at 1:46
okay. so it turns out the teacher doesn't care if it shows up as a string because as you said, a string is just a list of chars
– Aditya Sirohi
Nov 10 at 1:46
@AdityaSirohi: Just for your own reference, the
newtype
solution DarthFennec mentions is: newtype C = C Char; instance Show C where show (C c) = show c
. Then show (map C "abc")
will produce the string "['a', 'b', 'c']"
as expected. This is also a good opportunity to use Data.Coerce.coerce
, which converts safely between a type and a newtype
wrapper, with no runtime cost, e.g. coerce "abc" :: [C]
.– Jon Purdy
Nov 10 at 7:15
@AdityaSirohi: Just for your own reference, the
newtype
solution DarthFennec mentions is: newtype C = C Char; instance Show C where show (C c) = show c
. Then show (map C "abc")
will produce the string "['a', 'b', 'c']"
as expected. This is also a good opportunity to use Data.Coerce.coerce
, which converts safely between a type and a newtype
wrapper, with no runtime cost, e.g. coerce "abc" :: [C]
.– Jon Purdy
Nov 10 at 7:15
Slight quibble:
show
doesn't print anything; it returns a string.– chepner
Nov 10 at 14:31
Slight quibble:
show
doesn't print anything; it returns a string.– chepner
Nov 10 at 14:31
@chepner,
show
doesn't "return" anything, it evaluates to a string. >:)– luqui
Nov 11 at 20:24
@chepner,
show
doesn't "return" anything, it evaluates to a string. >:)– luqui
Nov 11 at 20:24
That's an even bigger quibble than my original quibble :)
– chepner
Nov 11 at 20:31
That's an even bigger quibble than my original quibble :)
– chepner
Nov 11 at 20:31
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53234906%2fhaskell-code-prints-out-a-list-for-ints-but-not-for-chars%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
HIwH 8MMToTk3ngKyCFE9u7Qpf1AIN UbDkVbhqQyDDnWf5bB7ubaSI DrymYVbniJjcAc4NA,iV1
Are you supposed to print it in a list format, or just return a list? What are the requirements specifically?
– DarthFennec
Nov 10 at 0:30
That's what's the
Show
instance for lists does. If you want some other behavior, don't useshow
.– Carl
Nov 10 at 0:41