Does csv.DicReader() return a dictionary object?
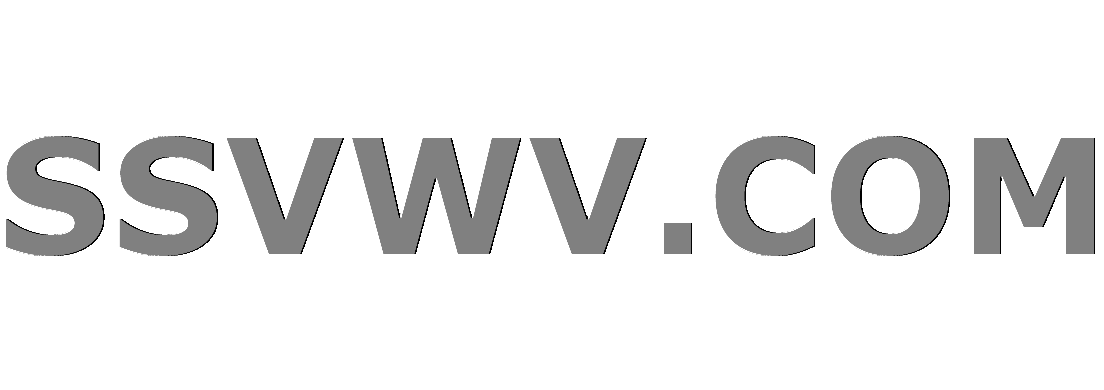
Multi tool use
Does csv.DicReader() return a dictionary object?
While I am trying to use csv.DictReader()
to transfer a CSV file to a dictionary, like this:
csv.DictReader()
csv_file = open(input_file, "r")
data = csv.DictReader(csv_file)
However, I cannot apply any dictionary methods into data
.
data
Thus, I print the type of data
.
data
print(type(data))
and find the type is:
<class 'csv.DictReader'>
So does csv.DictReader()
really return a dictionary object? How can I get a dictionary object from CSV?
csv.DictReader()
My CSV file looks like this:
[['play', 'weather', 'temperature'], ['yes', 'sunny', '77'], ['no', 'rainny', '60'], ['yes', 'windy', '70'],...]
Actually, I do not have a expected output. I just want to store these value for my further calculation.
Edit (as per comment): The output could be two parts. The first one is the headers of the csv file, such as ['play', 'weather', 'temperature']
. The second one can be this: [['yes', 'sunny', '77'], ['no', 'rainny', '60'], ['yes', 'windy', '70']...]
['play', 'weather', 'temperature']
[['yes', 'sunny', '77'], ['no', 'rainny', '60'], ['yes', 'windy', '70']...]
Given your example, what do you want your desired dictionary to look like?
– jpp
Sep 8 '18 at 23:23
I have added the file. But basically, I don't know which way is the best way to store it. @jpp
– sissi
Sep 8 '18 at 23:25
@Aran-FeyI have added the file. But basically, I don't know which way is the best way to store it.
– sissi
Sep 8 '18 at 23:26
If you look to store for calculations than will suggest to look for pandas dataframe instead of dict ...
– n1tk
Sep 8 '18 at 23:41
2 Answers
2
Given your desired output, you do not need to use dict
or, consequently, csv.DictReader
. Instead, just use csv.reader
, which returns an iterator. Then use next
and list
to extract headers and data respectively:
dict
csv.DictReader
csv.reader
next
list
from io import StringIO
import csv
x = StringIO("""play,weather,temperature
yes,sunny,77
no,rainy,60
yes,windy,70""")
# replace x with open('file.csv', 'r')
with x as fin:
reader = csv.reader(fin)
headers = next(reader) # get headers from first row
data = list(reader) # exhaust iterator from second row onwards
The result is a list of headers, and a list of lists for data:
print(headers)
['play', 'weather', 'temperature']
print(data)
[['yes', 'sunny', '77'],
['no', 'rainy', '60'],
['yes', 'windy', '70']]
If you are willing to use a 3rd party library, Pandas may be a better option as it handles type conversion and indexing more conveniently:
import pandas as pd
df = pd.read_csv('file.csv')
The result is a pd.DataFrame
object:
pd.DataFrame
print(df)
play weather temperature
0 yes sunny 77
1 no rainy 60
2 yes windy 70
print(type(df))
<class 'pandas.core.frame.DataFrame'>
Thanks a lot! It is exactly what I want.
– sissi
Sep 9 '18 at 0:02
DictReader
returns a file-like object. It still reads the data from the csv file in one row at a time, but the returned rows are ordered dictionaries instead of lists.
DictReader
If your files is:
play,weather,temperature
yes,sunny,77
no,rainny,60
yes,windy,70
Then you can use DictReader
in the following way:
DictReader
with open('path/to/file.csv') as fp:
header = fp.readline().strip().split(',')
dreader = DictReader(fp, header)
data = list(dreader)
In this case, data
will be a list of OrderedDict
objects with the mapping from the headers to each item in the row.
data
OrderedDict
data
#returns:
[OrderedDict([('play', 'yes'), ('weather', 'sunny'), ('temperature', '77')]),
OrderedDict([('play', 'no'), ('weather', 'rainny'), ('temperature', '60')]),
OrderedDict([('play', 'yes'), ('weather', 'windy'), ('temperature', '70')])]
Thanks for contributing an answer to Stack Overflow!
But avoid …
To learn more, see our tips on writing great answers.
Required, but never shown
Required, but never shown
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Could you maybe show us what your csv file looks like and what kind of dictionary you want to get as output?
– Aran-Fey
Sep 8 '18 at 23:10