Why is loop not stopping at defined stop point?
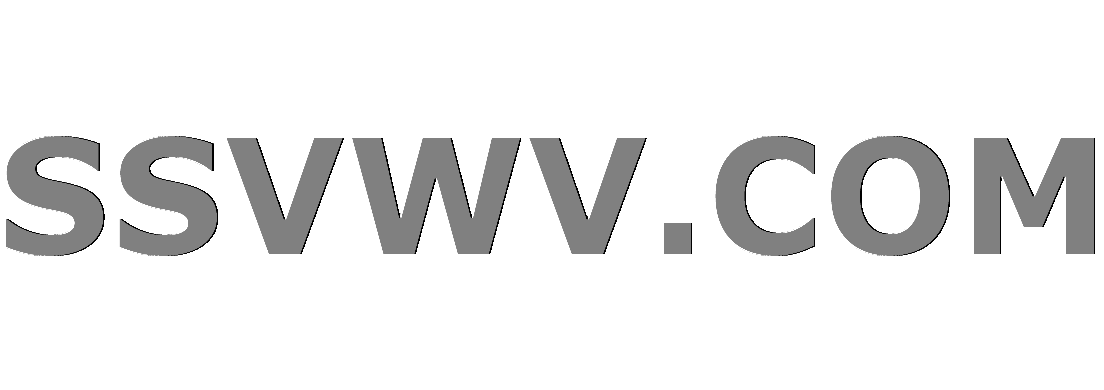
Multi tool use
up vote
0
down vote
favorite
I am working on a quiz for an online course, testing understanding of loops. The problem is:
"Write a function called laugh() that takes one parameter, num that represents the number of “ha”s to return. Such that console.log(laugh(3));
prints "hahaha!"
" I solved this using the following loop:
function laugh(num)
var string = ""
for (var i = 0; i < num; i++)
string = string + "ha";
return string + "!";
console.log(laugh(3));
But out of curiosity, I increased num
and had the loop return i
instead of the string:
function laugh(num)
var string = "";
for (var i = 0; i < num; i++)
string = string + "ha";
return i;
console.log(laugh(16))
This printed 16
. Now I'm confused - shouldn't i
only reach 15
since one of the conditions of the loop is that i < num
and num
is set to 16
?
javascript function loops for-loop
add a comment |
up vote
0
down vote
favorite
I am working on a quiz for an online course, testing understanding of loops. The problem is:
"Write a function called laugh() that takes one parameter, num that represents the number of “ha”s to return. Such that console.log(laugh(3));
prints "hahaha!"
" I solved this using the following loop:
function laugh(num)
var string = ""
for (var i = 0; i < num; i++)
string = string + "ha";
return string + "!";
console.log(laugh(3));
But out of curiosity, I increased num
and had the loop return i
instead of the string:
function laugh(num)
var string = "";
for (var i = 0; i < num; i++)
string = string + "ha";
return i;
console.log(laugh(16))
This printed 16
. Now I'm confused - shouldn't i
only reach 15
since one of the conditions of the loop is that i < num
and num
is set to 16
?
javascript function loops for-loop
1
The test occurs after the increment.
– CertainPerformance
Nov 9 at 3:37
no, @Plotisateur, post or pre increment makes absolutely no difference in this code
– Bravo
Nov 9 at 3:43
@Plotisateur Even if it were a pre-increment (++i
), the effect would be the same - the "test" part is checked after thefinal-expression
part is finished.
– CertainPerformance
Nov 9 at 3:44
Supossei
is equal to 15, then the condition15 < 16
will be true and for sure you will still be in the loop. So there is no way the method can return 15. Wheni
reach the value16
then the loop is finished (because16 < 16
isfalse
) and that value is returned.
– D. Smania
Nov 9 at 3:46
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am working on a quiz for an online course, testing understanding of loops. The problem is:
"Write a function called laugh() that takes one parameter, num that represents the number of “ha”s to return. Such that console.log(laugh(3));
prints "hahaha!"
" I solved this using the following loop:
function laugh(num)
var string = ""
for (var i = 0; i < num; i++)
string = string + "ha";
return string + "!";
console.log(laugh(3));
But out of curiosity, I increased num
and had the loop return i
instead of the string:
function laugh(num)
var string = "";
for (var i = 0; i < num; i++)
string = string + "ha";
return i;
console.log(laugh(16))
This printed 16
. Now I'm confused - shouldn't i
only reach 15
since one of the conditions of the loop is that i < num
and num
is set to 16
?
javascript function loops for-loop
I am working on a quiz for an online course, testing understanding of loops. The problem is:
"Write a function called laugh() that takes one parameter, num that represents the number of “ha”s to return. Such that console.log(laugh(3));
prints "hahaha!"
" I solved this using the following loop:
function laugh(num)
var string = ""
for (var i = 0; i < num; i++)
string = string + "ha";
return string + "!";
console.log(laugh(3));
But out of curiosity, I increased num
and had the loop return i
instead of the string:
function laugh(num)
var string = "";
for (var i = 0; i < num; i++)
string = string + "ha";
return i;
console.log(laugh(16))
This printed 16
. Now I'm confused - shouldn't i
only reach 15
since one of the conditions of the loop is that i < num
and num
is set to 16
?
javascript function loops for-loop
javascript function loops for-loop
asked Nov 9 at 3:36


Carlo Tapia
212
212
1
The test occurs after the increment.
– CertainPerformance
Nov 9 at 3:37
no, @Plotisateur, post or pre increment makes absolutely no difference in this code
– Bravo
Nov 9 at 3:43
@Plotisateur Even if it were a pre-increment (++i
), the effect would be the same - the "test" part is checked after thefinal-expression
part is finished.
– CertainPerformance
Nov 9 at 3:44
Supossei
is equal to 15, then the condition15 < 16
will be true and for sure you will still be in the loop. So there is no way the method can return 15. Wheni
reach the value16
then the loop is finished (because16 < 16
isfalse
) and that value is returned.
– D. Smania
Nov 9 at 3:46
add a comment |
1
The test occurs after the increment.
– CertainPerformance
Nov 9 at 3:37
no, @Plotisateur, post or pre increment makes absolutely no difference in this code
– Bravo
Nov 9 at 3:43
@Plotisateur Even if it were a pre-increment (++i
), the effect would be the same - the "test" part is checked after thefinal-expression
part is finished.
– CertainPerformance
Nov 9 at 3:44
Supossei
is equal to 15, then the condition15 < 16
will be true and for sure you will still be in the loop. So there is no way the method can return 15. Wheni
reach the value16
then the loop is finished (because16 < 16
isfalse
) and that value is returned.
– D. Smania
Nov 9 at 3:46
1
1
The test occurs after the increment.
– CertainPerformance
Nov 9 at 3:37
The test occurs after the increment.
– CertainPerformance
Nov 9 at 3:37
no, @Plotisateur, post or pre increment makes absolutely no difference in this code
– Bravo
Nov 9 at 3:43
no, @Plotisateur, post or pre increment makes absolutely no difference in this code
– Bravo
Nov 9 at 3:43
@Plotisateur Even if it were a pre-increment (
++i
), the effect would be the same - the "test" part is checked after the final-expression
part is finished.– CertainPerformance
Nov 9 at 3:44
@Plotisateur Even if it were a pre-increment (
++i
), the effect would be the same - the "test" part is checked after the final-expression
part is finished.– CertainPerformance
Nov 9 at 3:44
Suposse
i
is equal to 15, then the condition 15 < 16
will be true and for sure you will still be in the loop. So there is no way the method can return 15. When i
reach the value 16
then the loop is finished (because 16 < 16
is false
) and that value is returned.– D. Smania
Nov 9 at 3:46
Suposse
i
is equal to 15, then the condition 15 < 16
will be true and for sure you will still be in the loop. So there is no way the method can return 15. When i
reach the value 16
then the loop is finished (because 16 < 16
is false
) and that value is returned.– D. Smania
Nov 9 at 3:46
add a comment |
4 Answers
4
active
oldest
votes
up vote
0
down vote
In for loop first is increment done after that condition is check. So when "i" incremented to 15 then it checks 15<16, true so continue with rest of the code.
After that, "i" incremented to 16 and checks 16<16, false so came out from the loop.
So, If you print "i", then it will print current value of "i" which is 16
Thank you. I understand now!
– Carlo Tapia
Nov 9 at 18:34
add a comment |
up vote
0
down vote
The value of i
starts at 0, then it is checked to see if it is less than 16, and lastly, it is increased by 1. On the last iteration, i
is 15, which satisfies the condition of being less than 15, and then it is incremented. Now, i
is 16, which is not less than 16, so the for loop finishes with i
equal to 16.
add a comment |
up vote
0
down vote
See, you start i at 0. Walk through the loop step by step. It will execute for i = 0,1,2,3...15. But now you see, the loop executes for 0. So for any loop of the form (int i = 0; i < num; i++), i reaches num. Convention is to start counting at 0 because that's convention.
add a comment |
up vote
0
down vote
The loop exit condition is i < num
, so when i >= 16 code string = string + "ha";
is not executing
add a comment |
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
In for loop first is increment done after that condition is check. So when "i" incremented to 15 then it checks 15<16, true so continue with rest of the code.
After that, "i" incremented to 16 and checks 16<16, false so came out from the loop.
So, If you print "i", then it will print current value of "i" which is 16
Thank you. I understand now!
– Carlo Tapia
Nov 9 at 18:34
add a comment |
up vote
0
down vote
In for loop first is increment done after that condition is check. So when "i" incremented to 15 then it checks 15<16, true so continue with rest of the code.
After that, "i" incremented to 16 and checks 16<16, false so came out from the loop.
So, If you print "i", then it will print current value of "i" which is 16
Thank you. I understand now!
– Carlo Tapia
Nov 9 at 18:34
add a comment |
up vote
0
down vote
up vote
0
down vote
In for loop first is increment done after that condition is check. So when "i" incremented to 15 then it checks 15<16, true so continue with rest of the code.
After that, "i" incremented to 16 and checks 16<16, false so came out from the loop.
So, If you print "i", then it will print current value of "i" which is 16
In for loop first is increment done after that condition is check. So when "i" incremented to 15 then it checks 15<16, true so continue with rest of the code.
After that, "i" incremented to 16 and checks 16<16, false so came out from the loop.
So, If you print "i", then it will print current value of "i" which is 16
answered Nov 9 at 3:45
Biplab Malakar
39837
39837
Thank you. I understand now!
– Carlo Tapia
Nov 9 at 18:34
add a comment |
Thank you. I understand now!
– Carlo Tapia
Nov 9 at 18:34
Thank you. I understand now!
– Carlo Tapia
Nov 9 at 18:34
Thank you. I understand now!
– Carlo Tapia
Nov 9 at 18:34
add a comment |
up vote
0
down vote
The value of i
starts at 0, then it is checked to see if it is less than 16, and lastly, it is increased by 1. On the last iteration, i
is 15, which satisfies the condition of being less than 15, and then it is incremented. Now, i
is 16, which is not less than 16, so the for loop finishes with i
equal to 16.
add a comment |
up vote
0
down vote
The value of i
starts at 0, then it is checked to see if it is less than 16, and lastly, it is increased by 1. On the last iteration, i
is 15, which satisfies the condition of being less than 15, and then it is incremented. Now, i
is 16, which is not less than 16, so the for loop finishes with i
equal to 16.
add a comment |
up vote
0
down vote
up vote
0
down vote
The value of i
starts at 0, then it is checked to see if it is less than 16, and lastly, it is increased by 1. On the last iteration, i
is 15, which satisfies the condition of being less than 15, and then it is incremented. Now, i
is 16, which is not less than 16, so the for loop finishes with i
equal to 16.
The value of i
starts at 0, then it is checked to see if it is less than 16, and lastly, it is increased by 1. On the last iteration, i
is 15, which satisfies the condition of being less than 15, and then it is incremented. Now, i
is 16, which is not less than 16, so the for loop finishes with i
equal to 16.
edited Nov 9 at 3:59
answered Nov 9 at 3:53
hev1
5,4653527
5,4653527
add a comment |
add a comment |
up vote
0
down vote
See, you start i at 0. Walk through the loop step by step. It will execute for i = 0,1,2,3...15. But now you see, the loop executes for 0. So for any loop of the form (int i = 0; i < num; i++), i reaches num. Convention is to start counting at 0 because that's convention.
add a comment |
up vote
0
down vote
See, you start i at 0. Walk through the loop step by step. It will execute for i = 0,1,2,3...15. But now you see, the loop executes for 0. So for any loop of the form (int i = 0; i < num; i++), i reaches num. Convention is to start counting at 0 because that's convention.
add a comment |
up vote
0
down vote
up vote
0
down vote
See, you start i at 0. Walk through the loop step by step. It will execute for i = 0,1,2,3...15. But now you see, the loop executes for 0. So for any loop of the form (int i = 0; i < num; i++), i reaches num. Convention is to start counting at 0 because that's convention.
See, you start i at 0. Walk through the loop step by step. It will execute for i = 0,1,2,3...15. But now you see, the loop executes for 0. So for any loop of the form (int i = 0; i < num; i++), i reaches num. Convention is to start counting at 0 because that's convention.
answered Nov 9 at 4:00


RDJ
11
11
add a comment |
add a comment |
up vote
0
down vote
The loop exit condition is i < num
, so when i >= 16 code string = string + "ha";
is not executing
add a comment |
up vote
0
down vote
The loop exit condition is i < num
, so when i >= 16 code string = string + "ha";
is not executing
add a comment |
up vote
0
down vote
up vote
0
down vote
The loop exit condition is i < num
, so when i >= 16 code string = string + "ha";
is not executing
The loop exit condition is i < num
, so when i >= 16 code string = string + "ha";
is not executing
edited Nov 9 at 9:45
answered Nov 9 at 3:43


Evgeny A. Mamonov
39636
39636
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53219490%2fwhy-is-loop-not-stopping-at-defined-stop-point%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
PrYax0DFV9cFoXRpR GG6RVgxSVG OP8P 2pQRr,FsjJlYy,va6w5kzT2GXNoRL1QWu
1
The test occurs after the increment.
– CertainPerformance
Nov 9 at 3:37
no, @Plotisateur, post or pre increment makes absolutely no difference in this code
– Bravo
Nov 9 at 3:43
@Plotisateur Even if it were a pre-increment (
++i
), the effect would be the same - the "test" part is checked after thefinal-expression
part is finished.– CertainPerformance
Nov 9 at 3:44
Suposse
i
is equal to 15, then the condition15 < 16
will be true and for sure you will still be in the loop. So there is no way the method can return 15. Wheni
reach the value16
then the loop is finished (because16 < 16
isfalse
) and that value is returned.– D. Smania
Nov 9 at 3:46