Conditionally call ViewComponent inside Razor Page
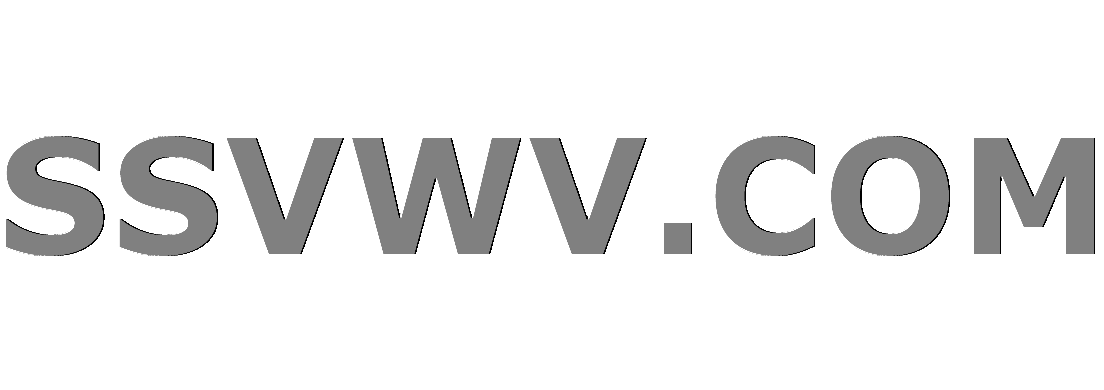
Multi tool use
I'm new to Razor Pages and View Components and I'm cleary failing to put my head around the concepts:
I have a Example page
, this page is rendered inside a _Layout
, like the following:
@page "value1?/value2?"
@model MyProject.Pages.ExampleModel
@
ViewData["Title"] = "Example";
Now I need to call a ViewComponent conditionally based on value1
and value2
:
public class ExampleModel : PageModel
public async Task<IActionResult> OnGetAsync(string value1, string value2)
// this contains a lot of logic, db access etc, and passing objects to the component, but I'm abbreviating
if (value1 == null && value2 == null)
return this.ViewComponent(nameof(SomeComponent));
else
return this.ViewComponent(nameof(SomeOtherComponent));
// return Page();
This is the extension I'm using to call the components:
public static class PageModelExtensions
public static ViewComponentResult ViewComponent(this PageModel pageModel, string componentName, object arguments)
return new ViewComponentResult
ViewComponentName = componentName,
Arguments = arguments,
ViewData = pageModel.ViewData,
TempData = pageModel.TempData
;
This is working, but because I'm not calling return Page()
, the component is being rendered without calling _Layout
. How should I approach this?
c# asp.net-core
add a comment |
I'm new to Razor Pages and View Components and I'm cleary failing to put my head around the concepts:
I have a Example page
, this page is rendered inside a _Layout
, like the following:
@page "value1?/value2?"
@model MyProject.Pages.ExampleModel
@
ViewData["Title"] = "Example";
Now I need to call a ViewComponent conditionally based on value1
and value2
:
public class ExampleModel : PageModel
public async Task<IActionResult> OnGetAsync(string value1, string value2)
// this contains a lot of logic, db access etc, and passing objects to the component, but I'm abbreviating
if (value1 == null && value2 == null)
return this.ViewComponent(nameof(SomeComponent));
else
return this.ViewComponent(nameof(SomeOtherComponent));
// return Page();
This is the extension I'm using to call the components:
public static class PageModelExtensions
public static ViewComponentResult ViewComponent(this PageModel pageModel, string componentName, object arguments)
return new ViewComponentResult
ViewComponentName = componentName,
Arguments = arguments,
ViewData = pageModel.ViewData,
TempData = pageModel.TempData
;
This is working, but because I'm not calling return Page()
, the component is being rendered without calling _Layout
. How should I approach this?
c# asp.net-core
add a comment |
I'm new to Razor Pages and View Components and I'm cleary failing to put my head around the concepts:
I have a Example page
, this page is rendered inside a _Layout
, like the following:
@page "value1?/value2?"
@model MyProject.Pages.ExampleModel
@
ViewData["Title"] = "Example";
Now I need to call a ViewComponent conditionally based on value1
and value2
:
public class ExampleModel : PageModel
public async Task<IActionResult> OnGetAsync(string value1, string value2)
// this contains a lot of logic, db access etc, and passing objects to the component, but I'm abbreviating
if (value1 == null && value2 == null)
return this.ViewComponent(nameof(SomeComponent));
else
return this.ViewComponent(nameof(SomeOtherComponent));
// return Page();
This is the extension I'm using to call the components:
public static class PageModelExtensions
public static ViewComponentResult ViewComponent(this PageModel pageModel, string componentName, object arguments)
return new ViewComponentResult
ViewComponentName = componentName,
Arguments = arguments,
ViewData = pageModel.ViewData,
TempData = pageModel.TempData
;
This is working, but because I'm not calling return Page()
, the component is being rendered without calling _Layout
. How should I approach this?
c# asp.net-core
I'm new to Razor Pages and View Components and I'm cleary failing to put my head around the concepts:
I have a Example page
, this page is rendered inside a _Layout
, like the following:
@page "value1?/value2?"
@model MyProject.Pages.ExampleModel
@
ViewData["Title"] = "Example";
Now I need to call a ViewComponent conditionally based on value1
and value2
:
public class ExampleModel : PageModel
public async Task<IActionResult> OnGetAsync(string value1, string value2)
// this contains a lot of logic, db access etc, and passing objects to the component, but I'm abbreviating
if (value1 == null && value2 == null)
return this.ViewComponent(nameof(SomeComponent));
else
return this.ViewComponent(nameof(SomeOtherComponent));
// return Page();
This is the extension I'm using to call the components:
public static class PageModelExtensions
public static ViewComponentResult ViewComponent(this PageModel pageModel, string componentName, object arguments)
return new ViewComponentResult
ViewComponentName = componentName,
Arguments = arguments,
ViewData = pageModel.ViewData,
TempData = pageModel.TempData
;
This is working, but because I'm not calling return Page()
, the component is being rendered without calling _Layout
. How should I approach this?
c# asp.net-core
c# asp.net-core
edited Nov 10 '18 at 16:22
Kirk Larkin
20k33857
20k33857
asked Nov 10 '18 at 16:17
lolollolol
2,57622643
2,57622643
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
If you want to render a View Component from within a Razor Page like this, one option is to specify the name of said View Component in your PageModel
class and then use Component.InvokeAsync
in the correpsonding .cshtml file. Here's what that might look like:
Example.cshtml.cs
public class ExampleModel : PageModel
public string ViewComponentName get; set;
public async Task<IActionResult> OnGetAsync(string value1, string value2)
...
if (value1 == null && value2 == null)
ViewComponentName = nameof(SomeComponent);
else
ViewComponentName = nameof(SomeOtherComponent);
return Page();
Example.cshtml
@page "value1?/value2?"
@model MyProject.Pages.ExampleModel
...
@await Component.InvokeAsync(Model.ViewComponentName)
In Example.cshtml.cs, ViewComponentName
is a new property that (unsurprisingly) represents the name of the View Component that should be rendered as part of the page, which is realised in Example.cshtml using Component.InvokeAsync
.
1
Oh, I see. I can see how to apply the same method using parametersComponent.InvokeAsync(Model.ViewComponentName, Model.SomeParameter)
. Is this right? Thx.
– lolol
Nov 10 '18 at 16:36
Yes, that looks right.
– Kirk Larkin
Nov 10 '18 at 16:37
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53240883%2fconditionally-call-viewcomponent-inside-razor-page%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you want to render a View Component from within a Razor Page like this, one option is to specify the name of said View Component in your PageModel
class and then use Component.InvokeAsync
in the correpsonding .cshtml file. Here's what that might look like:
Example.cshtml.cs
public class ExampleModel : PageModel
public string ViewComponentName get; set;
public async Task<IActionResult> OnGetAsync(string value1, string value2)
...
if (value1 == null && value2 == null)
ViewComponentName = nameof(SomeComponent);
else
ViewComponentName = nameof(SomeOtherComponent);
return Page();
Example.cshtml
@page "value1?/value2?"
@model MyProject.Pages.ExampleModel
...
@await Component.InvokeAsync(Model.ViewComponentName)
In Example.cshtml.cs, ViewComponentName
is a new property that (unsurprisingly) represents the name of the View Component that should be rendered as part of the page, which is realised in Example.cshtml using Component.InvokeAsync
.
1
Oh, I see. I can see how to apply the same method using parametersComponent.InvokeAsync(Model.ViewComponentName, Model.SomeParameter)
. Is this right? Thx.
– lolol
Nov 10 '18 at 16:36
Yes, that looks right.
– Kirk Larkin
Nov 10 '18 at 16:37
add a comment |
If you want to render a View Component from within a Razor Page like this, one option is to specify the name of said View Component in your PageModel
class and then use Component.InvokeAsync
in the correpsonding .cshtml file. Here's what that might look like:
Example.cshtml.cs
public class ExampleModel : PageModel
public string ViewComponentName get; set;
public async Task<IActionResult> OnGetAsync(string value1, string value2)
...
if (value1 == null && value2 == null)
ViewComponentName = nameof(SomeComponent);
else
ViewComponentName = nameof(SomeOtherComponent);
return Page();
Example.cshtml
@page "value1?/value2?"
@model MyProject.Pages.ExampleModel
...
@await Component.InvokeAsync(Model.ViewComponentName)
In Example.cshtml.cs, ViewComponentName
is a new property that (unsurprisingly) represents the name of the View Component that should be rendered as part of the page, which is realised in Example.cshtml using Component.InvokeAsync
.
1
Oh, I see. I can see how to apply the same method using parametersComponent.InvokeAsync(Model.ViewComponentName, Model.SomeParameter)
. Is this right? Thx.
– lolol
Nov 10 '18 at 16:36
Yes, that looks right.
– Kirk Larkin
Nov 10 '18 at 16:37
add a comment |
If you want to render a View Component from within a Razor Page like this, one option is to specify the name of said View Component in your PageModel
class and then use Component.InvokeAsync
in the correpsonding .cshtml file. Here's what that might look like:
Example.cshtml.cs
public class ExampleModel : PageModel
public string ViewComponentName get; set;
public async Task<IActionResult> OnGetAsync(string value1, string value2)
...
if (value1 == null && value2 == null)
ViewComponentName = nameof(SomeComponent);
else
ViewComponentName = nameof(SomeOtherComponent);
return Page();
Example.cshtml
@page "value1?/value2?"
@model MyProject.Pages.ExampleModel
...
@await Component.InvokeAsync(Model.ViewComponentName)
In Example.cshtml.cs, ViewComponentName
is a new property that (unsurprisingly) represents the name of the View Component that should be rendered as part of the page, which is realised in Example.cshtml using Component.InvokeAsync
.
If you want to render a View Component from within a Razor Page like this, one option is to specify the name of said View Component in your PageModel
class and then use Component.InvokeAsync
in the correpsonding .cshtml file. Here's what that might look like:
Example.cshtml.cs
public class ExampleModel : PageModel
public string ViewComponentName get; set;
public async Task<IActionResult> OnGetAsync(string value1, string value2)
...
if (value1 == null && value2 == null)
ViewComponentName = nameof(SomeComponent);
else
ViewComponentName = nameof(SomeOtherComponent);
return Page();
Example.cshtml
@page "value1?/value2?"
@model MyProject.Pages.ExampleModel
...
@await Component.InvokeAsync(Model.ViewComponentName)
In Example.cshtml.cs, ViewComponentName
is a new property that (unsurprisingly) represents the name of the View Component that should be rendered as part of the page, which is realised in Example.cshtml using Component.InvokeAsync
.
answered Nov 10 '18 at 16:32
Kirk LarkinKirk Larkin
20k33857
20k33857
1
Oh, I see. I can see how to apply the same method using parametersComponent.InvokeAsync(Model.ViewComponentName, Model.SomeParameter)
. Is this right? Thx.
– lolol
Nov 10 '18 at 16:36
Yes, that looks right.
– Kirk Larkin
Nov 10 '18 at 16:37
add a comment |
1
Oh, I see. I can see how to apply the same method using parametersComponent.InvokeAsync(Model.ViewComponentName, Model.SomeParameter)
. Is this right? Thx.
– lolol
Nov 10 '18 at 16:36
Yes, that looks right.
– Kirk Larkin
Nov 10 '18 at 16:37
1
1
Oh, I see. I can see how to apply the same method using parameters
Component.InvokeAsync(Model.ViewComponentName, Model.SomeParameter)
. Is this right? Thx.– lolol
Nov 10 '18 at 16:36
Oh, I see. I can see how to apply the same method using parameters
Component.InvokeAsync(Model.ViewComponentName, Model.SomeParameter)
. Is this right? Thx.– lolol
Nov 10 '18 at 16:36
Yes, that looks right.
– Kirk Larkin
Nov 10 '18 at 16:37
Yes, that looks right.
– Kirk Larkin
Nov 10 '18 at 16:37
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53240883%2fconditionally-call-viewcomponent-inside-razor-page%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
UVEF Oa7hfUhlM1M7hk,NRp,bN3 Hi,6Y NnFy7gWXSejJ,e,FPsHJMpLxgbZQ,dOFO BDJ3m8vpGTjOsNkkRwNPZuA9LOo,hlwTDd