Incorrect if-else statements
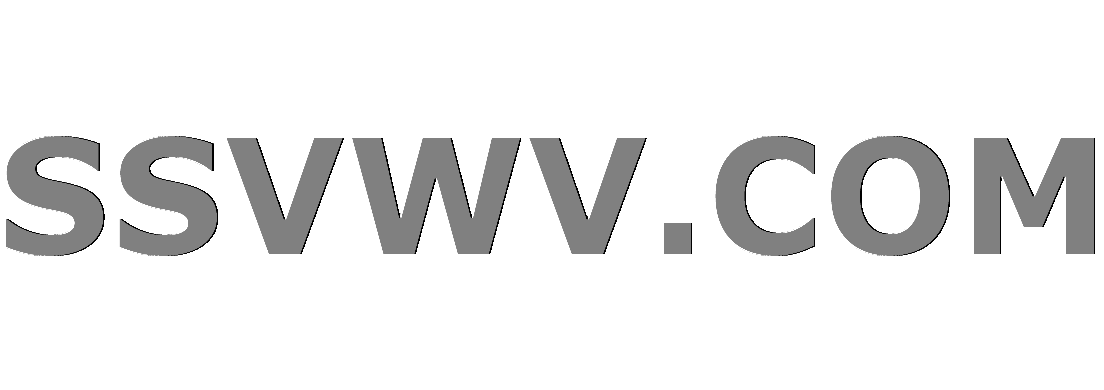
Multi tool use
Incorrect if-else statements
I've been trying to write a function that will ask for a number between 1-11 and will randomly choose a number and will compare between them.
I can't figure out why no matter what number I type in (equals or smaller or bigger) it will always type the "Your number is less" message.
def loto():
_number = int(input("Enter any number between 1-10: "))
import random
for x in range(1):
print ("Python chose: " + str(random.randint(1,11)))
if ("_number" == "random"):
print ("You Won! :)")
if ("_number" < "random"):
print ("Your number is less")
if ("_number" > "random"):
print ("Your number is more")
else:
print ("You Lost :(")
loto()
I'm using Python 3.
Thanks:)
You also need to use
elif
, not separate if
statements. Otherwise, the You Lost
message gets printed whenever _number > random
is not true, instead of only whenever all of the three comparisons are not true.– abarnert
Sep 2 at 17:52
elif
if
You Lost
_number > random
but if I take "" off it will say: TypeError: '<' not supported between instances of 'int' and 'module'
– marinaaa
Sep 2 at 17:53
While we're at it: why are you using
_number
instead of number
, why do you have parentheses around your conditions, and why are you doing for x in range(1):
which just loops once, setting x
to 0, and never using x
? You're making your code more complicated and harder to understand to no benefit.– abarnert
Sep 2 at 17:53
_number
number
for x in range(1):
x
x
That's because your code makes no sense. What is
_number == random
supposed to do? _number
is a number, but random
is a module.– melpomene
Sep 2 at 17:54
_number == random
_number
random
2 Answers
2
Your first problem is that you're comparing the strings "_number"
and "random"
. ASCIIbetically (or, rather, Unicoderifically), "_number" < "random"
, because the _
character is #95 and the r
character is #114.
"_number"
"random"
"_number" < "random"
_
r
If you want to compare two variables, you just refer to the variables, not strings that happen to be the same as the names of those variables.
Your second problem is that random
isn't your random number, it's the module you used to create that number. And, more seriously, you aren't storing that number anywhere—you're just converting it to a string to print it out and then throwing it away.
random
Your third problem is that you need to change those if
s to elif
s. Otherwise, the You Lost
message gets printed whenever _number > random
is not true, instead of only whenever all of the three comparisons are not true.
if
elif
You Lost
_number > random
Putting that all together:
choice = random.randint(1,11)
for x in range(1):
print ("Python chose: " + str(choice))
if (_number == choice):
print ("You Won! :)")
elif (_number < choice):
print ("Your number is less")
elif (_number > choice):
print ("Your number is more")
else:
print ("You Lost :(")
Of course there's no way to actually lose your game—one of the three conditions is always going to be true. (If you were using complex numbers, or floats including NaN, you could input a number that wasn't comparable in any way to the selected one, but you're not.)
While we're at it:
_number
number
for x in range(1):
x
print
So:
import random
def loto():
number = int(input("Enter any number between 1-10: "))
choice = random.randint(1, 11)
print("Python chose:", choice)
if number == choice:
print("You Won! :)")
elif number < choice:
print("Your number is less")
elif number > choice:
print("Your number is more")
else:
print("You Lost :(")
loto()
@melpomene Yes, of course it is. But it's also not relevant to the problem. There are also a number of other things that can be improved that aren't relevant to the problem. That's why I first show the minimal changes to fix this, and then, separately, explain all of those improvements.
– abarnert
Sep 2 at 18:02
you awesome dude!
– marinaaa
Sep 2 at 18:11
You were comparing strings not variables, you need to remove quotes, and you not saved random number into a variable. The for loop is making one repetition only, you can remove it.
Update your cod like this:
import random
def loto():
_number = int(input("Enter any number between 1-10: "))
rand_number = random.randint(1,11) # can't had the same name as random
if (_number == rand_number):
print ("You Won! :)")
elif (_number < rand_number):
print ("Your number is less")
elif (_number > rand_number):
print ("Your number is more")
else:
print ("You Lost :(")
loto()
Thanks for contributing an answer to Stack Overflow!
But avoid …
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
But avoid …
To learn more, see our tips on writing great answers.
Required, but never shown
Required, but never shown
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
You are comparing strings, not variables.
– Daniel
Sep 2 at 17:50