Am I using '&&' incorrectly?
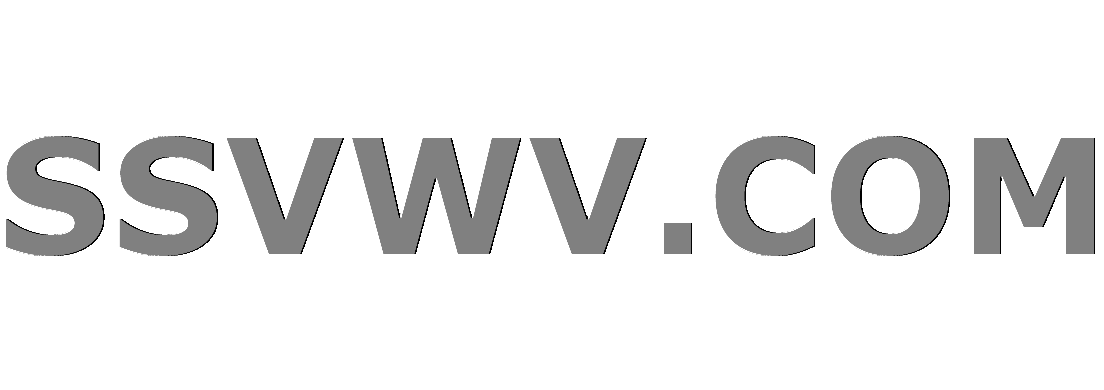
Multi tool use
Am I using '&&' incorrectly?
I have a problem in which I have to take 3 words from user as input. What I have to do with that input:
Here is the code I tried:
#include <stdio.h>
#include <string.h>
int main()
char first[20], second[20], third[20];
int i, j;
char vowel[5] = 'a', 'e', 'i', 'o', 'u' ;
printf("Enter first word: ");
scanf("%s", first);
printf("Enter second word: ");
scanf("%s", second);
printf("Enter third word: ");
scanf("%s", third);
for (i = 0; i < strlen(first); i++)
for (j = 0; j < 5; j++)
if (first[i] == vowel[j])
first[i] = '$';
printf("Final strings are: n");
printf("%s", first);
for (i = 0; i < strlen(second); i++)
if (second[i] != 'a' && second[i] != 'i' && second[i] != 'o' && second[i] != 'u' && second[i] != 'e');
second[i] = '#';
printf("%s", second);
printf("%s", strupr(third));
NOTE: All 3 words should be concatenated on output screen
Output:
Enter first word: kali
Enter second word: kali
Enter third word: kali
Final strings are:
k$l$####KALI
But the expected output is:
Enter first word: kali
Enter second word: kali
Enter third word: kali
Final strings are:
k$l$#a#iKALI
What I am doing wrong?
;
if
if
@Carcigenicate Yeah! that was the only problem.
– dave
Sep 2 at 17:01
Good. Seriously though, use
. That wasn't the problem here, but they may have made the problem more obvious, and omitting them can cause bugs later.– Carcigenicate
Sep 2 at 17:03
@dave That was far from the only problem, but it was that that caused the error you are describing. ;)
– Broman
Sep 2 at 17:23
regarding:
if(second[i] != 'a' && second[i] != 'i' && second[i] != 'o' && second[i] != 'u' && second[i] != 'u');
I doubt that you wanted to check for u
twice, however, the code does need modification to check for the (sometimes vowel) y
– user3629249
Sep 2 at 18:01
if(second[i] != 'a' && second[i] != 'i' && second[i] != 'o' && second[i] != 'u' && second[i] != 'u');
u
y
3 Answers
3
You made a silly mistake in this statement:
if (second[i] != 'a' && second[i] != 'i' && second[i] != 'o' && second[i] != 'u' && second[i] != 'u');
second[i] = '#';
You added an extra ;
at the end of the if
line, making the test useless and the following statement second[i] = '#';
execute unconditionally.
;
if
second[i] = '#';
You should break such long expressions on multiple lines, avoid redundant tests and use and
if (second[i] != 'a' && second[i] != 'i' && second[i] != 'o' &&
second[i] != 'u')
second[i] = '#';
There are lot of problems with your code. If you turn on compiler warnings you'll see this:
$ clang -Wall -Wextra -std=c11 -pedantic-errors b.c
b.c:32:109: warning: if statement has empty body [-Wempty-body]
...!= 'i' && second[i] != 'o' && second[i] != 'u' && second[i] != 'u');
^
b.c:32:109: note: put the semicolon on a separate line to silence this warning
b.c:37:18: warning: implicit declaration of function 'strupr' is invalid in C99
[-Wimplicit-function-declaration]
printf("%s", strupr(third));
^
b.c:37:18: warning: format specifies type 'char *' but the argument has type
'int' [-Wformat]
printf("%s", strupr(third));
~~ ^~~~~~~~~~~~~
%d
b.c:20:18: warning: comparison of integers of different signs: 'int' and
'unsigned long' [-Wsign-compare]
for(i = 0; i < strlen(first); i++){
~ ^ ~~~~~~~~~~~~~
b.c:31:18: warning: comparison of integers of different signs: 'int' and
'unsigned long' [-Wsign-compare]
for(i = 0; i < strlen(second); i++){
~ ^ ~~~~~~~~~~~~~~
5 warnings generated.
/tmp/b-8f1874.o: In function `main':
b.c:(.text+0x22a): undefined reference to `strupr'
clang: error: linker command failed with exit code 1 (use -v to see invocation)
If you google the warning messages you'll get good hints about what to do about them. The warning about the semicolon that makes the if
statement having an empty body is what causes your problems.
if
Another problem is that you don't check the return code of scanf
to see if the read was successful. It will return the number of successful reads.
scanf
Avoid using strupr
. It is a deprecated non-standard function.
strupr
To add to problems:
scanf("%s", first);
is like gets(first)
potential to overwrite array. Better to use scanf("%19s", first);
- something my compiler warns of– chux
Sep 2 at 17:55
scanf("%s", first);
gets(first)
scanf("%19s", first);
@Broman I really didn't understand you didn't check the return code of scanf to see if the read was successful, can you explain a bit in your answer?
– dave
Sep 3 at 3:01
@dave stackoverflow.com/questions/23967715/…
– Broman
Sep 3 at 9:14
#include <stdio.h>
#include <string.h>
int main()
char first[20], second[20], third[20];
int i, j;
char vowel[5] = 'a', 'e', 'i', 'o', 'u' ;
printf("Enter first word: ");
scanf("%s", first);
printf("Enter second word: ");
scanf("%s", second);
printf("Enter third word: ");
scanf("%s", third);
for (i = 0; i < strlen(first); i++)
for (j = 0; j < 5; j++)
if (first[i] == vowel[j])
first[i] = '$';
printf("Final strings are: n");
printf("%s", first);
for (i = 0; i < strlen(second); i++)
if (second[i] != 'a' && second[i] != 'e' && second[i] != 'i' && second[i] != 'o' && second[i] != 'u') // You mistakenly put a semicolon here
second[i] = '#';
printf("%s", second);
printf("%s", strupr(third));
Thanks for contributing an answer to Stack Overflow!
But avoid …
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
But avoid …
To learn more, see our tips on writing great answers.
Required, but never shown
Required, but never shown
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
I think your problem is just that you have a
;
at the end of yourif
, which causes theif
to end. Get rid of the semicolon, and wrap the part under it in curly braces. Never omit curly braces unless you have a good reason.– Carcigenicate
Sep 2 at 16:58