How to build a simple JavaScript to-do list with localStorage
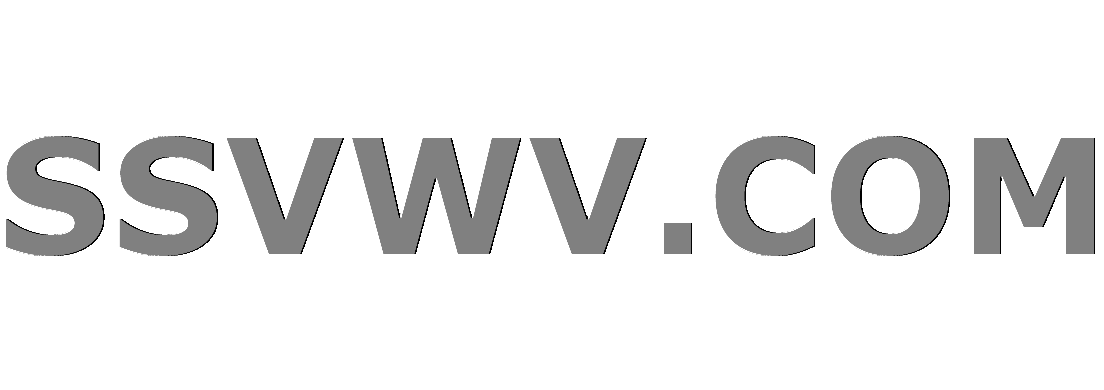
Multi tool use
I am building a simple JavaScript to-do list with DOM methods and am attempting to implement localStorage
for the list items. localStorage
appears to function properly when items are both added and removed. However, the word undefined
is thrown to the screen before the list items are rendered. Any idea why this is happening? Much appreciated!
JS:
var ul = document.getElementById("myUl");
function add()
var item = document.getElementById("newItem").value;
var itemTxt = document.createTextNode(item);
var li = document.createElement("li");
var btn = document.createElement("button");
var btnx = document.createTextNode("x");
btn.setAttribute("onclick", "remove()");
btn.appendChild(btnx);
li.appendChild(itemTxt);
li.appendChild(btn);
ul.appendChild(li);
function remove()
var task = this.event.currentTarget.parentNode;
ul.removeChild(task);
ul.innerHTML = localStorage["list"];
setInterval(function()
localStorage["list"] = ul.innerHTML;
, 1000);
HTML:
<input id="newItem" />
<button onclick="add()">add</button>
<ul id="myUl">New List</ul>
javascript
add a comment |
I am building a simple JavaScript to-do list with DOM methods and am attempting to implement localStorage
for the list items. localStorage
appears to function properly when items are both added and removed. However, the word undefined
is thrown to the screen before the list items are rendered. Any idea why this is happening? Much appreciated!
JS:
var ul = document.getElementById("myUl");
function add()
var item = document.getElementById("newItem").value;
var itemTxt = document.createTextNode(item);
var li = document.createElement("li");
var btn = document.createElement("button");
var btnx = document.createTextNode("x");
btn.setAttribute("onclick", "remove()");
btn.appendChild(btnx);
li.appendChild(itemTxt);
li.appendChild(btn);
ul.appendChild(li);
function remove()
var task = this.event.currentTarget.parentNode;
ul.removeChild(task);
ul.innerHTML = localStorage["list"];
setInterval(function()
localStorage["list"] = ul.innerHTML;
, 1000);
HTML:
<input id="newItem" />
<button onclick="add()">add</button>
<ul id="myUl">New List</ul>
javascript
3
You're usingul.innerHTML = localStorage["list"];
beforelocalStorage.list
has been populated
– CertainPerformance
Nov 12 '18 at 0:13
add a comment |
I am building a simple JavaScript to-do list with DOM methods and am attempting to implement localStorage
for the list items. localStorage
appears to function properly when items are both added and removed. However, the word undefined
is thrown to the screen before the list items are rendered. Any idea why this is happening? Much appreciated!
JS:
var ul = document.getElementById("myUl");
function add()
var item = document.getElementById("newItem").value;
var itemTxt = document.createTextNode(item);
var li = document.createElement("li");
var btn = document.createElement("button");
var btnx = document.createTextNode("x");
btn.setAttribute("onclick", "remove()");
btn.appendChild(btnx);
li.appendChild(itemTxt);
li.appendChild(btn);
ul.appendChild(li);
function remove()
var task = this.event.currentTarget.parentNode;
ul.removeChild(task);
ul.innerHTML = localStorage["list"];
setInterval(function()
localStorage["list"] = ul.innerHTML;
, 1000);
HTML:
<input id="newItem" />
<button onclick="add()">add</button>
<ul id="myUl">New List</ul>
javascript
I am building a simple JavaScript to-do list with DOM methods and am attempting to implement localStorage
for the list items. localStorage
appears to function properly when items are both added and removed. However, the word undefined
is thrown to the screen before the list items are rendered. Any idea why this is happening? Much appreciated!
JS:
var ul = document.getElementById("myUl");
function add()
var item = document.getElementById("newItem").value;
var itemTxt = document.createTextNode(item);
var li = document.createElement("li");
var btn = document.createElement("button");
var btnx = document.createTextNode("x");
btn.setAttribute("onclick", "remove()");
btn.appendChild(btnx);
li.appendChild(itemTxt);
li.appendChild(btn);
ul.appendChild(li);
function remove()
var task = this.event.currentTarget.parentNode;
ul.removeChild(task);
ul.innerHTML = localStorage["list"];
setInterval(function()
localStorage["list"] = ul.innerHTML;
, 1000);
HTML:
<input id="newItem" />
<button onclick="add()">add</button>
<ul id="myUl">New List</ul>
javascript
javascript
edited Nov 12 '18 at 2:53


qiAlex
2,0261724
2,0261724
asked Nov 12 '18 at 0:11


JS_is_awesome18JS_is_awesome18
434
434
3
You're usingul.innerHTML = localStorage["list"];
beforelocalStorage.list
has been populated
– CertainPerformance
Nov 12 '18 at 0:13
add a comment |
3
You're usingul.innerHTML = localStorage["list"];
beforelocalStorage.list
has been populated
– CertainPerformance
Nov 12 '18 at 0:13
3
3
You're using
ul.innerHTML = localStorage["list"];
before localStorage.list
has been populated– CertainPerformance
Nov 12 '18 at 0:13
You're using
ul.innerHTML = localStorage["list"];
before localStorage.list
has been populated– CertainPerformance
Nov 12 '18 at 0:13
add a comment |
1 Answer
1
active
oldest
votes
I updated code with comments:
var ul = document.getElementById("myUl");
function add()
var item = document.getElementById("newItem").value;
var itemTxt = document.createTextNode(item);
var li = document.createElement("li");
var btn = document.createElement("button");
var btnx = document.createTextNode("x");
btn.setAttribute("onclick", "remove()");
btn.appendChild(btnx);
li.appendChild(itemTxt);
li.appendChild(btn);
ul.appendChild(li);
localStorage["list"] = ul.innerHTML // updating localstorage
function remove()
var task = this.event.currentTarget.parentNode;
ul.removeChild(task);
localStorage["list"] = ul.innerHTML // updating localstorage
if (localStorage["list"]) // checking, if there is something in localstorage
ul.innerHTML = localStorage["list"];
Demo: https://codepen.io/anon/pen/dQpwpz
NB: It is not a best practice to put html
into localStorage
, but I believe you know it.
Thanks for responding. I checked the demo, but it looks like "undefined" still shows up. Any idea how to set this code up to remove that?
– JS_is_awesome18
Nov 12 '18 at 0:56
I did post answer, but then find an error in demo. Most likelyundefined
was saved intolocalStarage
before the code was fixed. try to cleanlocalStorage
in browser and refresh a page (or use incognito mode in Chrome).
– qiAlex
Nov 12 '18 at 1:02
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53254522%2fhow-to-build-a-simple-javascript-to-do-list-with-localstorage%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I updated code with comments:
var ul = document.getElementById("myUl");
function add()
var item = document.getElementById("newItem").value;
var itemTxt = document.createTextNode(item);
var li = document.createElement("li");
var btn = document.createElement("button");
var btnx = document.createTextNode("x");
btn.setAttribute("onclick", "remove()");
btn.appendChild(btnx);
li.appendChild(itemTxt);
li.appendChild(btn);
ul.appendChild(li);
localStorage["list"] = ul.innerHTML // updating localstorage
function remove()
var task = this.event.currentTarget.parentNode;
ul.removeChild(task);
localStorage["list"] = ul.innerHTML // updating localstorage
if (localStorage["list"]) // checking, if there is something in localstorage
ul.innerHTML = localStorage["list"];
Demo: https://codepen.io/anon/pen/dQpwpz
NB: It is not a best practice to put html
into localStorage
, but I believe you know it.
Thanks for responding. I checked the demo, but it looks like "undefined" still shows up. Any idea how to set this code up to remove that?
– JS_is_awesome18
Nov 12 '18 at 0:56
I did post answer, but then find an error in demo. Most likelyundefined
was saved intolocalStarage
before the code was fixed. try to cleanlocalStorage
in browser and refresh a page (or use incognito mode in Chrome).
– qiAlex
Nov 12 '18 at 1:02
add a comment |
I updated code with comments:
var ul = document.getElementById("myUl");
function add()
var item = document.getElementById("newItem").value;
var itemTxt = document.createTextNode(item);
var li = document.createElement("li");
var btn = document.createElement("button");
var btnx = document.createTextNode("x");
btn.setAttribute("onclick", "remove()");
btn.appendChild(btnx);
li.appendChild(itemTxt);
li.appendChild(btn);
ul.appendChild(li);
localStorage["list"] = ul.innerHTML // updating localstorage
function remove()
var task = this.event.currentTarget.parentNode;
ul.removeChild(task);
localStorage["list"] = ul.innerHTML // updating localstorage
if (localStorage["list"]) // checking, if there is something in localstorage
ul.innerHTML = localStorage["list"];
Demo: https://codepen.io/anon/pen/dQpwpz
NB: It is not a best practice to put html
into localStorage
, but I believe you know it.
Thanks for responding. I checked the demo, but it looks like "undefined" still shows up. Any idea how to set this code up to remove that?
– JS_is_awesome18
Nov 12 '18 at 0:56
I did post answer, but then find an error in demo. Most likelyundefined
was saved intolocalStarage
before the code was fixed. try to cleanlocalStorage
in browser and refresh a page (or use incognito mode in Chrome).
– qiAlex
Nov 12 '18 at 1:02
add a comment |
I updated code with comments:
var ul = document.getElementById("myUl");
function add()
var item = document.getElementById("newItem").value;
var itemTxt = document.createTextNode(item);
var li = document.createElement("li");
var btn = document.createElement("button");
var btnx = document.createTextNode("x");
btn.setAttribute("onclick", "remove()");
btn.appendChild(btnx);
li.appendChild(itemTxt);
li.appendChild(btn);
ul.appendChild(li);
localStorage["list"] = ul.innerHTML // updating localstorage
function remove()
var task = this.event.currentTarget.parentNode;
ul.removeChild(task);
localStorage["list"] = ul.innerHTML // updating localstorage
if (localStorage["list"]) // checking, if there is something in localstorage
ul.innerHTML = localStorage["list"];
Demo: https://codepen.io/anon/pen/dQpwpz
NB: It is not a best practice to put html
into localStorage
, but I believe you know it.
I updated code with comments:
var ul = document.getElementById("myUl");
function add()
var item = document.getElementById("newItem").value;
var itemTxt = document.createTextNode(item);
var li = document.createElement("li");
var btn = document.createElement("button");
var btnx = document.createTextNode("x");
btn.setAttribute("onclick", "remove()");
btn.appendChild(btnx);
li.appendChild(itemTxt);
li.appendChild(btn);
ul.appendChild(li);
localStorage["list"] = ul.innerHTML // updating localstorage
function remove()
var task = this.event.currentTarget.parentNode;
ul.removeChild(task);
localStorage["list"] = ul.innerHTML // updating localstorage
if (localStorage["list"]) // checking, if there is something in localstorage
ul.innerHTML = localStorage["list"];
Demo: https://codepen.io/anon/pen/dQpwpz
NB: It is not a best practice to put html
into localStorage
, but I believe you know it.
edited Nov 12 '18 at 1:02
answered Nov 12 '18 at 0:50


qiAlexqiAlex
2,0261724
2,0261724
Thanks for responding. I checked the demo, but it looks like "undefined" still shows up. Any idea how to set this code up to remove that?
– JS_is_awesome18
Nov 12 '18 at 0:56
I did post answer, but then find an error in demo. Most likelyundefined
was saved intolocalStarage
before the code was fixed. try to cleanlocalStorage
in browser and refresh a page (or use incognito mode in Chrome).
– qiAlex
Nov 12 '18 at 1:02
add a comment |
Thanks for responding. I checked the demo, but it looks like "undefined" still shows up. Any idea how to set this code up to remove that?
– JS_is_awesome18
Nov 12 '18 at 0:56
I did post answer, but then find an error in demo. Most likelyundefined
was saved intolocalStarage
before the code was fixed. try to cleanlocalStorage
in browser and refresh a page (or use incognito mode in Chrome).
– qiAlex
Nov 12 '18 at 1:02
Thanks for responding. I checked the demo, but it looks like "undefined" still shows up. Any idea how to set this code up to remove that?
– JS_is_awesome18
Nov 12 '18 at 0:56
Thanks for responding. I checked the demo, but it looks like "undefined" still shows up. Any idea how to set this code up to remove that?
– JS_is_awesome18
Nov 12 '18 at 0:56
I did post answer, but then find an error in demo. Most likely
undefined
was saved into localStarage
before the code was fixed. try to clean localStorage
in browser and refresh a page (or use incognito mode in Chrome).– qiAlex
Nov 12 '18 at 1:02
I did post answer, but then find an error in demo. Most likely
undefined
was saved into localStarage
before the code was fixed. try to clean localStorage
in browser and refresh a page (or use incognito mode in Chrome).– qiAlex
Nov 12 '18 at 1:02
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53254522%2fhow-to-build-a-simple-javascript-to-do-list-with-localstorage%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
deyKlNtzN XvRqTkIEnFFZPtg,IJv52Lr,IL09,Jy4kM7 aVZQ zwiH,ms
3
You're using
ul.innerHTML = localStorage["list"];
beforelocalStorage.list
has been populated– CertainPerformance
Nov 12 '18 at 0:13