Opentk shows out of memory exception - OPENGL
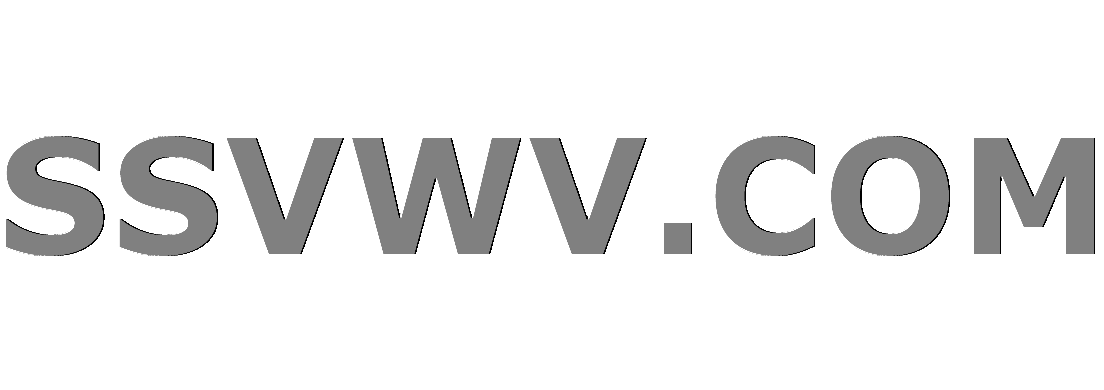
Multi tool use
up vote
1
down vote
favorite
I'm completely new to openGL.
I have to show two images side by side using shader code.
It shows properly using below code. But throws an
out of memory
exception and the entire system gets hangs.
Can you please check my code to see what might be the cause?
I think the problem is that I'm not properly deallocating memory from openTK textures after I don't need them, so I'm generating massive amounts of images, but I don't know how to fix this.
public partial class Form7ImageOnly : Form
string file = "opentksquare.png";
string file1 = "img_smile.png";
int program;
int vertShader;
int fragShader;
int buffer;
int positionLocation;
int positionLocation1;
int positionLocation2;
int texture;
int texture1;
int ScreenWidth;
int ScreenHeight;
float vertices =
// Left bottom triangle
-1f, -1f, 0f,
1f, -1f, 0f,
1f, 1f, 0f,
// Right top triangle
1f, 1f, 0f,
-1f, 1f, 0f,
-1f, -1f, 0f
;
public Form7ImageOnly()
InitializeComponent();
private void Form7ImageOnly_Load(object sender, EventArgs e)
glControl.Resize += new EventHandler(glControl_Resize);
glControl.Paint += new PaintEventHandler(glControl_Paint);
GL.Enable(EnableCap.DepthTest);
Application.Idle += Application_Idle;
glControl_Resize(glControl, EventArgs.Empty);
void Application_Idle(object sender, EventArgs e)
while (glControl.IsIdle)
Render();
private void Render()
ClearBufferMask.DepthBufferBit);
DrawImage(texture,texture1);
private void glControl_Paint(object sender, PaintEventArgs e)
Render();
private void glControl_Resize(object sender, EventArgs e)
Init();
private void Init()
CreateShaders();
CreateProgram();
InitBuffers();
public void DrawImage(int image,int image1)
GL.Viewport(new Rectangle(0, 0, ScreenWidth, ScreenHeight));
GL.MatrixMode(MatrixMode.Projection);
GL.PushMatrix();
GL.LoadIdentity();
GL.MatrixMode(MatrixMode.Modelview);
GL.PushMatrix();
GL.LoadIdentity();
GL.Disable(EnableCap.Lighting);
GL.Enable(EnableCap.Texture2D);
GL.ActiveTexture(TextureUnit.Texture0);
GL.BindTexture(TextureTarget.Texture2D, image);
GL.Uniform1(positionLocation1, 0);
GL.ActiveTexture(TextureUnit.Texture1);
GL.BindTexture(TextureTarget.Texture2D, image1);
GL.Uniform1(positionLocation2, 1);
RunShaders();
//GL.Begin(PrimitiveType.Quads);
//GL.TexCoord2(0, 1);
//GL.Vertex3(0, 0, 0);
//GL.TexCoord2(1, 1);
//GL.Vertex3(1920, 0, 0);
//GL.TexCoord2(1, 0);
//GL.Vertex3(1920, 1080, 0);
//GL.TexCoord2(0, 0);
//GL.Vertex3(0, 1080, 0);
//GL.End();
GL.Disable(EnableCap.Texture2D);
GL.PopMatrix();
GL.MatrixMode(MatrixMode.Projection);
GL.PopMatrix();
GL.MatrixMode(MatrixMode.Modelview);
ErrorCode ec = GL.GetError();
if (ec != 0)
System.Console.WriteLine(ec.ToString());
Console.Read();
glControl.SwapBuffers();
private void RunShaders()
GL.ClearColor(Color.Violet);
GL.UseProgram(program);
GL.DrawArrays(PrimitiveType.Triangles, 0, vertices.Length / 3);
ErrorCode ec = GL.GetError();
if (ec != 0)
System.Console.WriteLine(ec.ToString());
Console.Read();
private void CreateProgram()
program = GL.CreateProgram();
GL.AttachShader(program, vertShader);
GL.AttachShader(program, fragShader);
GL.LinkProgram(program);
private void CreateShaders()
/***********Vert Shader********************/
vertShader = GL.CreateShader(ShaderType.VertexShader);
GL.ShaderSource(vertShader, @"attribute vec3 a_position;
varying vec2 vTexCoord;
void main()
vTexCoord = (a_position.xy + 1) / 2;
gl_Position = vec4(a_position, 1);
");
GL.CompileShader(vertShader);
/***********Frag Shader ****************/
fragShader = GL.CreateShader(ShaderType.FragmentShader);
GL.ShaderSource(fragShader, @"precision highp float;
uniform sampler2D sTexture;uniform sampler2D sTexture1;
uniform int screenwidth;
varying vec2 vTexCoord;
void main ()
if ( vTexCoord.x<0.5 )
gl_FragColor = texture2D (sTexture, vec2(vTexCoord.x*2.0, vTexCoord.y));
else
gl_FragColor = texture2D (sTexture1, vec2(1.0- (vTexCoord.x*2.0-1.0), vTexCoord.y));
");
GL.CompileShader(fragShader);
private void InitBuffers()
buffer = GL.GenBuffer();
positionLocation = GL.GetAttribLocation(program, "a_position");
positionLocation1 = GL.GetUniformLocation(program, "sTexture");
positionLocation2 = GL.GetUniformLocation(program, "sTexture1");
GL.EnableVertexAttribArray(positionLocation);
GL.BindBuffer(BufferTarget.ArrayBuffer, buffer);
GL.BufferData(BufferTarget.ArrayBuffer, (IntPtr)(vertices.Length * sizeof(float)), vertices, BufferUsageHint.StaticDraw);
GL.VertexAttribPointer(positionLocation, 3, VertexAttribPointerType.Float, false, 0,0);
public int LoadTexture(string file)
Bitmap bitmap = new Bitmap(file);
int tex = -1;
if (bitmap != null)
GL.DeleteTextures(1, ref tex);
GL.Hint(HintTarget.PerspectiveCorrectionHint, HintMode.Nicest);
GL.GenTextures(1, out tex);
GL.BindTexture(TextureTarget.Texture2D, tex);
bitmap.RotateFlip(RotateFlipType.RotateNoneFlipY);
BitmapData data = bitmap.LockBits(new System.Drawing.Rectangle(0, 0, bitmap.Width, bitmap.Height),
ImageLockMode.ReadOnly, System.Drawing.Imaging.PixelFormat.Format32bppArgb);
GL.TexImage2D(TextureTarget.Texture2D, 0, PixelInternalFormat.Rgba,data.Width,data.Height, 0,
OpenTK.Graphics.OpenGL.PixelFormat.Bgra, PixelType.UnsignedByte, data.Scan0);
bitmap.UnlockBits(data);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureMinFilter, (int)TextureMinFilter.Linear);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureMagFilter, (int)TextureMagFilter.Linear);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureWrapS, (int)TextureWrapMode.ClampToEdge);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureWrapT, (int)TextureWrapMode.ClampToEdge);
return tex;
c# opengl out-of-memory glsl opentk
|
show 4 more comments
up vote
1
down vote
favorite
I'm completely new to openGL.
I have to show two images side by side using shader code.
It shows properly using below code. But throws an
out of memory
exception and the entire system gets hangs.
Can you please check my code to see what might be the cause?
I think the problem is that I'm not properly deallocating memory from openTK textures after I don't need them, so I'm generating massive amounts of images, but I don't know how to fix this.
public partial class Form7ImageOnly : Form
string file = "opentksquare.png";
string file1 = "img_smile.png";
int program;
int vertShader;
int fragShader;
int buffer;
int positionLocation;
int positionLocation1;
int positionLocation2;
int texture;
int texture1;
int ScreenWidth;
int ScreenHeight;
float vertices =
// Left bottom triangle
-1f, -1f, 0f,
1f, -1f, 0f,
1f, 1f, 0f,
// Right top triangle
1f, 1f, 0f,
-1f, 1f, 0f,
-1f, -1f, 0f
;
public Form7ImageOnly()
InitializeComponent();
private void Form7ImageOnly_Load(object sender, EventArgs e)
glControl.Resize += new EventHandler(glControl_Resize);
glControl.Paint += new PaintEventHandler(glControl_Paint);
GL.Enable(EnableCap.DepthTest);
Application.Idle += Application_Idle;
glControl_Resize(glControl, EventArgs.Empty);
void Application_Idle(object sender, EventArgs e)
while (glControl.IsIdle)
Render();
private void Render()
ClearBufferMask.DepthBufferBit);
DrawImage(texture,texture1);
private void glControl_Paint(object sender, PaintEventArgs e)
Render();
private void glControl_Resize(object sender, EventArgs e)
Init();
private void Init()
CreateShaders();
CreateProgram();
InitBuffers();
public void DrawImage(int image,int image1)
GL.Viewport(new Rectangle(0, 0, ScreenWidth, ScreenHeight));
GL.MatrixMode(MatrixMode.Projection);
GL.PushMatrix();
GL.LoadIdentity();
GL.MatrixMode(MatrixMode.Modelview);
GL.PushMatrix();
GL.LoadIdentity();
GL.Disable(EnableCap.Lighting);
GL.Enable(EnableCap.Texture2D);
GL.ActiveTexture(TextureUnit.Texture0);
GL.BindTexture(TextureTarget.Texture2D, image);
GL.Uniform1(positionLocation1, 0);
GL.ActiveTexture(TextureUnit.Texture1);
GL.BindTexture(TextureTarget.Texture2D, image1);
GL.Uniform1(positionLocation2, 1);
RunShaders();
//GL.Begin(PrimitiveType.Quads);
//GL.TexCoord2(0, 1);
//GL.Vertex3(0, 0, 0);
//GL.TexCoord2(1, 1);
//GL.Vertex3(1920, 0, 0);
//GL.TexCoord2(1, 0);
//GL.Vertex3(1920, 1080, 0);
//GL.TexCoord2(0, 0);
//GL.Vertex3(0, 1080, 0);
//GL.End();
GL.Disable(EnableCap.Texture2D);
GL.PopMatrix();
GL.MatrixMode(MatrixMode.Projection);
GL.PopMatrix();
GL.MatrixMode(MatrixMode.Modelview);
ErrorCode ec = GL.GetError();
if (ec != 0)
System.Console.WriteLine(ec.ToString());
Console.Read();
glControl.SwapBuffers();
private void RunShaders()
GL.ClearColor(Color.Violet);
GL.UseProgram(program);
GL.DrawArrays(PrimitiveType.Triangles, 0, vertices.Length / 3);
ErrorCode ec = GL.GetError();
if (ec != 0)
System.Console.WriteLine(ec.ToString());
Console.Read();
private void CreateProgram()
program = GL.CreateProgram();
GL.AttachShader(program, vertShader);
GL.AttachShader(program, fragShader);
GL.LinkProgram(program);
private void CreateShaders()
/***********Vert Shader********************/
vertShader = GL.CreateShader(ShaderType.VertexShader);
GL.ShaderSource(vertShader, @"attribute vec3 a_position;
varying vec2 vTexCoord;
void main()
vTexCoord = (a_position.xy + 1) / 2;
gl_Position = vec4(a_position, 1);
");
GL.CompileShader(vertShader);
/***********Frag Shader ****************/
fragShader = GL.CreateShader(ShaderType.FragmentShader);
GL.ShaderSource(fragShader, @"precision highp float;
uniform sampler2D sTexture;uniform sampler2D sTexture1;
uniform int screenwidth;
varying vec2 vTexCoord;
void main ()
if ( vTexCoord.x<0.5 )
gl_FragColor = texture2D (sTexture, vec2(vTexCoord.x*2.0, vTexCoord.y));
else
gl_FragColor = texture2D (sTexture1, vec2(1.0- (vTexCoord.x*2.0-1.0), vTexCoord.y));
");
GL.CompileShader(fragShader);
private void InitBuffers()
buffer = GL.GenBuffer();
positionLocation = GL.GetAttribLocation(program, "a_position");
positionLocation1 = GL.GetUniformLocation(program, "sTexture");
positionLocation2 = GL.GetUniformLocation(program, "sTexture1");
GL.EnableVertexAttribArray(positionLocation);
GL.BindBuffer(BufferTarget.ArrayBuffer, buffer);
GL.BufferData(BufferTarget.ArrayBuffer, (IntPtr)(vertices.Length * sizeof(float)), vertices, BufferUsageHint.StaticDraw);
GL.VertexAttribPointer(positionLocation, 3, VertexAttribPointerType.Float, false, 0,0);
public int LoadTexture(string file)
Bitmap bitmap = new Bitmap(file);
int tex = -1;
if (bitmap != null)
GL.DeleteTextures(1, ref tex);
GL.Hint(HintTarget.PerspectiveCorrectionHint, HintMode.Nicest);
GL.GenTextures(1, out tex);
GL.BindTexture(TextureTarget.Texture2D, tex);
bitmap.RotateFlip(RotateFlipType.RotateNoneFlipY);
BitmapData data = bitmap.LockBits(new System.Drawing.Rectangle(0, 0, bitmap.Width, bitmap.Height),
ImageLockMode.ReadOnly, System.Drawing.Imaging.PixelFormat.Format32bppArgb);
GL.TexImage2D(TextureTarget.Texture2D, 0, PixelInternalFormat.Rgba,data.Width,data.Height, 0,
OpenTK.Graphics.OpenGL.PixelFormat.Bgra, PixelType.UnsignedByte, data.Scan0);
bitmap.UnlockBits(data);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureMinFilter, (int)TextureMinFilter.Linear);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureMagFilter, (int)TextureMagFilter.Linear);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureWrapS, (int)TextureWrapMode.ClampToEdge);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureWrapT, (int)TextureWrapMode.ClampToEdge);
return tex;
c# opengl out-of-memory glsl opentk
Do youLoadTexture
in every frame?
– Rabbid76
Nov 9 at 6:41
3
You create a new texture object on the GPU in every frameGL.GenTextures(1, out tex);
and you never delete it. You spam the GPU with equal texture objects. NoteGL.DeleteTextures(1, ref tex);
deletes always -1, so it does nothing.
– Rabbid76
Nov 9 at 6:57
1
If you load the textures inInit()
, then you don't need it at the moment.
– Rabbid76
Nov 9 at 7:11
1
To confirm what you said : If I call it in Render() the code should be like : GL.DeleteTextures(1, ref texture); texture = LoadTexture(file); GL.DeleteTextures(1, ref texture1) ; texture1 = LoadTexture(file1); And no need of line GL.DeleteTextures(1, ref tex); in LoadTexture(). Is'nt it?
– nsds
Nov 9 at 7:23
1
Yes it is, this should work
– Rabbid76
Nov 9 at 7:24
|
show 4 more comments
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm completely new to openGL.
I have to show two images side by side using shader code.
It shows properly using below code. But throws an
out of memory
exception and the entire system gets hangs.
Can you please check my code to see what might be the cause?
I think the problem is that I'm not properly deallocating memory from openTK textures after I don't need them, so I'm generating massive amounts of images, but I don't know how to fix this.
public partial class Form7ImageOnly : Form
string file = "opentksquare.png";
string file1 = "img_smile.png";
int program;
int vertShader;
int fragShader;
int buffer;
int positionLocation;
int positionLocation1;
int positionLocation2;
int texture;
int texture1;
int ScreenWidth;
int ScreenHeight;
float vertices =
// Left bottom triangle
-1f, -1f, 0f,
1f, -1f, 0f,
1f, 1f, 0f,
// Right top triangle
1f, 1f, 0f,
-1f, 1f, 0f,
-1f, -1f, 0f
;
public Form7ImageOnly()
InitializeComponent();
private void Form7ImageOnly_Load(object sender, EventArgs e)
glControl.Resize += new EventHandler(glControl_Resize);
glControl.Paint += new PaintEventHandler(glControl_Paint);
GL.Enable(EnableCap.DepthTest);
Application.Idle += Application_Idle;
glControl_Resize(glControl, EventArgs.Empty);
void Application_Idle(object sender, EventArgs e)
while (glControl.IsIdle)
Render();
private void Render()
ClearBufferMask.DepthBufferBit);
DrawImage(texture,texture1);
private void glControl_Paint(object sender, PaintEventArgs e)
Render();
private void glControl_Resize(object sender, EventArgs e)
Init();
private void Init()
CreateShaders();
CreateProgram();
InitBuffers();
public void DrawImage(int image,int image1)
GL.Viewport(new Rectangle(0, 0, ScreenWidth, ScreenHeight));
GL.MatrixMode(MatrixMode.Projection);
GL.PushMatrix();
GL.LoadIdentity();
GL.MatrixMode(MatrixMode.Modelview);
GL.PushMatrix();
GL.LoadIdentity();
GL.Disable(EnableCap.Lighting);
GL.Enable(EnableCap.Texture2D);
GL.ActiveTexture(TextureUnit.Texture0);
GL.BindTexture(TextureTarget.Texture2D, image);
GL.Uniform1(positionLocation1, 0);
GL.ActiveTexture(TextureUnit.Texture1);
GL.BindTexture(TextureTarget.Texture2D, image1);
GL.Uniform1(positionLocation2, 1);
RunShaders();
//GL.Begin(PrimitiveType.Quads);
//GL.TexCoord2(0, 1);
//GL.Vertex3(0, 0, 0);
//GL.TexCoord2(1, 1);
//GL.Vertex3(1920, 0, 0);
//GL.TexCoord2(1, 0);
//GL.Vertex3(1920, 1080, 0);
//GL.TexCoord2(0, 0);
//GL.Vertex3(0, 1080, 0);
//GL.End();
GL.Disable(EnableCap.Texture2D);
GL.PopMatrix();
GL.MatrixMode(MatrixMode.Projection);
GL.PopMatrix();
GL.MatrixMode(MatrixMode.Modelview);
ErrorCode ec = GL.GetError();
if (ec != 0)
System.Console.WriteLine(ec.ToString());
Console.Read();
glControl.SwapBuffers();
private void RunShaders()
GL.ClearColor(Color.Violet);
GL.UseProgram(program);
GL.DrawArrays(PrimitiveType.Triangles, 0, vertices.Length / 3);
ErrorCode ec = GL.GetError();
if (ec != 0)
System.Console.WriteLine(ec.ToString());
Console.Read();
private void CreateProgram()
program = GL.CreateProgram();
GL.AttachShader(program, vertShader);
GL.AttachShader(program, fragShader);
GL.LinkProgram(program);
private void CreateShaders()
/***********Vert Shader********************/
vertShader = GL.CreateShader(ShaderType.VertexShader);
GL.ShaderSource(vertShader, @"attribute vec3 a_position;
varying vec2 vTexCoord;
void main()
vTexCoord = (a_position.xy + 1) / 2;
gl_Position = vec4(a_position, 1);
");
GL.CompileShader(vertShader);
/***********Frag Shader ****************/
fragShader = GL.CreateShader(ShaderType.FragmentShader);
GL.ShaderSource(fragShader, @"precision highp float;
uniform sampler2D sTexture;uniform sampler2D sTexture1;
uniform int screenwidth;
varying vec2 vTexCoord;
void main ()
if ( vTexCoord.x<0.5 )
gl_FragColor = texture2D (sTexture, vec2(vTexCoord.x*2.0, vTexCoord.y));
else
gl_FragColor = texture2D (sTexture1, vec2(1.0- (vTexCoord.x*2.0-1.0), vTexCoord.y));
");
GL.CompileShader(fragShader);
private void InitBuffers()
buffer = GL.GenBuffer();
positionLocation = GL.GetAttribLocation(program, "a_position");
positionLocation1 = GL.GetUniformLocation(program, "sTexture");
positionLocation2 = GL.GetUniformLocation(program, "sTexture1");
GL.EnableVertexAttribArray(positionLocation);
GL.BindBuffer(BufferTarget.ArrayBuffer, buffer);
GL.BufferData(BufferTarget.ArrayBuffer, (IntPtr)(vertices.Length * sizeof(float)), vertices, BufferUsageHint.StaticDraw);
GL.VertexAttribPointer(positionLocation, 3, VertexAttribPointerType.Float, false, 0,0);
public int LoadTexture(string file)
Bitmap bitmap = new Bitmap(file);
int tex = -1;
if (bitmap != null)
GL.DeleteTextures(1, ref tex);
GL.Hint(HintTarget.PerspectiveCorrectionHint, HintMode.Nicest);
GL.GenTextures(1, out tex);
GL.BindTexture(TextureTarget.Texture2D, tex);
bitmap.RotateFlip(RotateFlipType.RotateNoneFlipY);
BitmapData data = bitmap.LockBits(new System.Drawing.Rectangle(0, 0, bitmap.Width, bitmap.Height),
ImageLockMode.ReadOnly, System.Drawing.Imaging.PixelFormat.Format32bppArgb);
GL.TexImage2D(TextureTarget.Texture2D, 0, PixelInternalFormat.Rgba,data.Width,data.Height, 0,
OpenTK.Graphics.OpenGL.PixelFormat.Bgra, PixelType.UnsignedByte, data.Scan0);
bitmap.UnlockBits(data);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureMinFilter, (int)TextureMinFilter.Linear);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureMagFilter, (int)TextureMagFilter.Linear);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureWrapS, (int)TextureWrapMode.ClampToEdge);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureWrapT, (int)TextureWrapMode.ClampToEdge);
return tex;
c# opengl out-of-memory glsl opentk
I'm completely new to openGL.
I have to show two images side by side using shader code.
It shows properly using below code. But throws an
out of memory
exception and the entire system gets hangs.
Can you please check my code to see what might be the cause?
I think the problem is that I'm not properly deallocating memory from openTK textures after I don't need them, so I'm generating massive amounts of images, but I don't know how to fix this.
public partial class Form7ImageOnly : Form
string file = "opentksquare.png";
string file1 = "img_smile.png";
int program;
int vertShader;
int fragShader;
int buffer;
int positionLocation;
int positionLocation1;
int positionLocation2;
int texture;
int texture1;
int ScreenWidth;
int ScreenHeight;
float vertices =
// Left bottom triangle
-1f, -1f, 0f,
1f, -1f, 0f,
1f, 1f, 0f,
// Right top triangle
1f, 1f, 0f,
-1f, 1f, 0f,
-1f, -1f, 0f
;
public Form7ImageOnly()
InitializeComponent();
private void Form7ImageOnly_Load(object sender, EventArgs e)
glControl.Resize += new EventHandler(glControl_Resize);
glControl.Paint += new PaintEventHandler(glControl_Paint);
GL.Enable(EnableCap.DepthTest);
Application.Idle += Application_Idle;
glControl_Resize(glControl, EventArgs.Empty);
void Application_Idle(object sender, EventArgs e)
while (glControl.IsIdle)
Render();
private void Render()
ClearBufferMask.DepthBufferBit);
DrawImage(texture,texture1);
private void glControl_Paint(object sender, PaintEventArgs e)
Render();
private void glControl_Resize(object sender, EventArgs e)
Init();
private void Init()
CreateShaders();
CreateProgram();
InitBuffers();
public void DrawImage(int image,int image1)
GL.Viewport(new Rectangle(0, 0, ScreenWidth, ScreenHeight));
GL.MatrixMode(MatrixMode.Projection);
GL.PushMatrix();
GL.LoadIdentity();
GL.MatrixMode(MatrixMode.Modelview);
GL.PushMatrix();
GL.LoadIdentity();
GL.Disable(EnableCap.Lighting);
GL.Enable(EnableCap.Texture2D);
GL.ActiveTexture(TextureUnit.Texture0);
GL.BindTexture(TextureTarget.Texture2D, image);
GL.Uniform1(positionLocation1, 0);
GL.ActiveTexture(TextureUnit.Texture1);
GL.BindTexture(TextureTarget.Texture2D, image1);
GL.Uniform1(positionLocation2, 1);
RunShaders();
//GL.Begin(PrimitiveType.Quads);
//GL.TexCoord2(0, 1);
//GL.Vertex3(0, 0, 0);
//GL.TexCoord2(1, 1);
//GL.Vertex3(1920, 0, 0);
//GL.TexCoord2(1, 0);
//GL.Vertex3(1920, 1080, 0);
//GL.TexCoord2(0, 0);
//GL.Vertex3(0, 1080, 0);
//GL.End();
GL.Disable(EnableCap.Texture2D);
GL.PopMatrix();
GL.MatrixMode(MatrixMode.Projection);
GL.PopMatrix();
GL.MatrixMode(MatrixMode.Modelview);
ErrorCode ec = GL.GetError();
if (ec != 0)
System.Console.WriteLine(ec.ToString());
Console.Read();
glControl.SwapBuffers();
private void RunShaders()
GL.ClearColor(Color.Violet);
GL.UseProgram(program);
GL.DrawArrays(PrimitiveType.Triangles, 0, vertices.Length / 3);
ErrorCode ec = GL.GetError();
if (ec != 0)
System.Console.WriteLine(ec.ToString());
Console.Read();
private void CreateProgram()
program = GL.CreateProgram();
GL.AttachShader(program, vertShader);
GL.AttachShader(program, fragShader);
GL.LinkProgram(program);
private void CreateShaders()
/***********Vert Shader********************/
vertShader = GL.CreateShader(ShaderType.VertexShader);
GL.ShaderSource(vertShader, @"attribute vec3 a_position;
varying vec2 vTexCoord;
void main()
vTexCoord = (a_position.xy + 1) / 2;
gl_Position = vec4(a_position, 1);
");
GL.CompileShader(vertShader);
/***********Frag Shader ****************/
fragShader = GL.CreateShader(ShaderType.FragmentShader);
GL.ShaderSource(fragShader, @"precision highp float;
uniform sampler2D sTexture;uniform sampler2D sTexture1;
uniform int screenwidth;
varying vec2 vTexCoord;
void main ()
if ( vTexCoord.x<0.5 )
gl_FragColor = texture2D (sTexture, vec2(vTexCoord.x*2.0, vTexCoord.y));
else
gl_FragColor = texture2D (sTexture1, vec2(1.0- (vTexCoord.x*2.0-1.0), vTexCoord.y));
");
GL.CompileShader(fragShader);
private void InitBuffers()
buffer = GL.GenBuffer();
positionLocation = GL.GetAttribLocation(program, "a_position");
positionLocation1 = GL.GetUniformLocation(program, "sTexture");
positionLocation2 = GL.GetUniformLocation(program, "sTexture1");
GL.EnableVertexAttribArray(positionLocation);
GL.BindBuffer(BufferTarget.ArrayBuffer, buffer);
GL.BufferData(BufferTarget.ArrayBuffer, (IntPtr)(vertices.Length * sizeof(float)), vertices, BufferUsageHint.StaticDraw);
GL.VertexAttribPointer(positionLocation, 3, VertexAttribPointerType.Float, false, 0,0);
public int LoadTexture(string file)
Bitmap bitmap = new Bitmap(file);
int tex = -1;
if (bitmap != null)
GL.DeleteTextures(1, ref tex);
GL.Hint(HintTarget.PerspectiveCorrectionHint, HintMode.Nicest);
GL.GenTextures(1, out tex);
GL.BindTexture(TextureTarget.Texture2D, tex);
bitmap.RotateFlip(RotateFlipType.RotateNoneFlipY);
BitmapData data = bitmap.LockBits(new System.Drawing.Rectangle(0, 0, bitmap.Width, bitmap.Height),
ImageLockMode.ReadOnly, System.Drawing.Imaging.PixelFormat.Format32bppArgb);
GL.TexImage2D(TextureTarget.Texture2D, 0, PixelInternalFormat.Rgba,data.Width,data.Height, 0,
OpenTK.Graphics.OpenGL.PixelFormat.Bgra, PixelType.UnsignedByte, data.Scan0);
bitmap.UnlockBits(data);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureMinFilter, (int)TextureMinFilter.Linear);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureMagFilter, (int)TextureMagFilter.Linear);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureWrapS, (int)TextureWrapMode.ClampToEdge);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureWrapT, (int)TextureWrapMode.ClampToEdge);
return tex;
c# opengl out-of-memory glsl opentk
c# opengl out-of-memory glsl opentk
edited Nov 9 at 6:53
asked Nov 9 at 4:56
nsds
2652620
2652620
Do youLoadTexture
in every frame?
– Rabbid76
Nov 9 at 6:41
3
You create a new texture object on the GPU in every frameGL.GenTextures(1, out tex);
and you never delete it. You spam the GPU with equal texture objects. NoteGL.DeleteTextures(1, ref tex);
deletes always -1, so it does nothing.
– Rabbid76
Nov 9 at 6:57
1
If you load the textures inInit()
, then you don't need it at the moment.
– Rabbid76
Nov 9 at 7:11
1
To confirm what you said : If I call it in Render() the code should be like : GL.DeleteTextures(1, ref texture); texture = LoadTexture(file); GL.DeleteTextures(1, ref texture1) ; texture1 = LoadTexture(file1); And no need of line GL.DeleteTextures(1, ref tex); in LoadTexture(). Is'nt it?
– nsds
Nov 9 at 7:23
1
Yes it is, this should work
– Rabbid76
Nov 9 at 7:24
|
show 4 more comments
Do youLoadTexture
in every frame?
– Rabbid76
Nov 9 at 6:41
3
You create a new texture object on the GPU in every frameGL.GenTextures(1, out tex);
and you never delete it. You spam the GPU with equal texture objects. NoteGL.DeleteTextures(1, ref tex);
deletes always -1, so it does nothing.
– Rabbid76
Nov 9 at 6:57
1
If you load the textures inInit()
, then you don't need it at the moment.
– Rabbid76
Nov 9 at 7:11
1
To confirm what you said : If I call it in Render() the code should be like : GL.DeleteTextures(1, ref texture); texture = LoadTexture(file); GL.DeleteTextures(1, ref texture1) ; texture1 = LoadTexture(file1); And no need of line GL.DeleteTextures(1, ref tex); in LoadTexture(). Is'nt it?
– nsds
Nov 9 at 7:23
1
Yes it is, this should work
– Rabbid76
Nov 9 at 7:24
Do you
LoadTexture
in every frame?– Rabbid76
Nov 9 at 6:41
Do you
LoadTexture
in every frame?– Rabbid76
Nov 9 at 6:41
3
3
You create a new texture object on the GPU in every frame
GL.GenTextures(1, out tex);
and you never delete it. You spam the GPU with equal texture objects. Note GL.DeleteTextures(1, ref tex);
deletes always -1, so it does nothing.– Rabbid76
Nov 9 at 6:57
You create a new texture object on the GPU in every frame
GL.GenTextures(1, out tex);
and you never delete it. You spam the GPU with equal texture objects. Note GL.DeleteTextures(1, ref tex);
deletes always -1, so it does nothing.– Rabbid76
Nov 9 at 6:57
1
1
If you load the textures in
Init()
, then you don't need it at the moment.– Rabbid76
Nov 9 at 7:11
If you load the textures in
Init()
, then you don't need it at the moment.– Rabbid76
Nov 9 at 7:11
1
1
To confirm what you said : If I call it in Render() the code should be like : GL.DeleteTextures(1, ref texture); texture = LoadTexture(file); GL.DeleteTextures(1, ref texture1) ; texture1 = LoadTexture(file1); And no need of line GL.DeleteTextures(1, ref tex); in LoadTexture(). Is'nt it?
– nsds
Nov 9 at 7:23
To confirm what you said : If I call it in Render() the code should be like : GL.DeleteTextures(1, ref texture); texture = LoadTexture(file); GL.DeleteTextures(1, ref texture1) ; texture1 = LoadTexture(file1); And no need of line GL.DeleteTextures(1, ref tex); in LoadTexture(). Is'nt it?
– nsds
Nov 9 at 7:23
1
1
Yes it is, this should work
– Rabbid76
Nov 9 at 7:24
Yes it is, this should work
– Rabbid76
Nov 9 at 7:24
|
show 4 more comments
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53220090%2fopentk-shows-out-of-memory-exception-opengl%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
tns,1,zWTbvAwt HVPn5L1 JO LAzPBDwF6mNJ0GySBwu5SWoGEXc0J m1C XjzxYcdddEyZ24OI21La3C v2
Do you
LoadTexture
in every frame?– Rabbid76
Nov 9 at 6:41
3
You create a new texture object on the GPU in every frame
GL.GenTextures(1, out tex);
and you never delete it. You spam the GPU with equal texture objects. NoteGL.DeleteTextures(1, ref tex);
deletes always -1, so it does nothing.– Rabbid76
Nov 9 at 6:57
1
If you load the textures in
Init()
, then you don't need it at the moment.– Rabbid76
Nov 9 at 7:11
1
To confirm what you said : If I call it in Render() the code should be like : GL.DeleteTextures(1, ref texture); texture = LoadTexture(file); GL.DeleteTextures(1, ref texture1) ; texture1 = LoadTexture(file1); And no need of line GL.DeleteTextures(1, ref tex); in LoadTexture(). Is'nt it?
– nsds
Nov 9 at 7:23
1
Yes it is, this should work
– Rabbid76
Nov 9 at 7:24