How to sum two lists of tuples
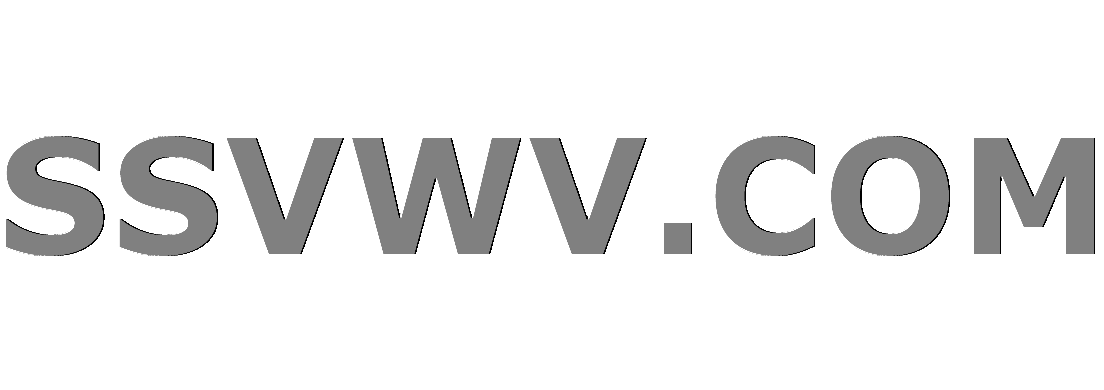
Multi tool use
How to sum two lists of tuples
list1 = [(1533945600000, 140), (1534032000000, 412), (1534118400000, 364), (1534204800000, 488), (1534291200000, 272), (1534377600000, 350), (1534464000000, 301), (1534550400000, 159), (1534636800000, 224), (1534723200000, 241), (1534809600000, 223), (1534896000000, 175)]
list2 = [(1533945600000, 1516), (1534032000000, 2176), (1534118400000, 2046), (1534204800000, 2400), (1534291200000, 8370), (1534377600000, 2112), (1534464000000, 1441), (1534550400000, 784), (1534636800000, 1391), (1534723200000, 1178), (1534809600000, 1020), (1534896000000, 795)]
How could you combine them into a single list of tuples? considering the first value of the key appears in both lists.
Just to clarify, you want to add the second items in each tuple but leave the first the same? Your first item being (1533945600000, 1656)? Have you done any work with tuples before?
– Lio Elbammalf
Aug 23 at 10:41
@LioElbammalf yes, it would be to sum the second value leaving the first value the same
– lapinkoira
Aug 23 at 10:42
Can you post what the output should look like? I.E what would you see after
print(output)
– jambrothers
Aug 23 at 10:43
print(output)
@lapinkoira does the order of 1st value of tuples can be different in list1 and list2?
– Sandeep Kadapa
Aug 23 at 10:47
4 Answers
4
use zip
on list1,list2 and then add second value of each tuple:
zip
lst =
for tup1,tup2 in zip(list1,list2):
sum_ = tup1[1]+tup2[1]
lst.append((tup1[0],sum_))
lst
[(1533945600000, 1656),
(1534032000000, 2588),
(1534118400000, 2410),
(1534204800000, 2888),
(1534291200000, 8642),
(1534377600000, 2462),
(1534464000000, 1742),
(1534550400000, 943),
(1534636800000, 1615),
(1534723200000, 1419),
(1534809600000, 1243),
(1534896000000, 970)]
This answer makes several assumptions that might be inappropriate: 1. The lists have the same length. 2. The tuples have the same keys in both lists. 3. The tuples are in the same order in both lists.
– Agost Biro
Aug 23 at 10:45
@AgostBiro My assumptions are correct as per OP's comment.
– Sandeep Kadapa
Aug 23 at 10:52
Since these assumptions aren't clear from the question, my comment was meant as warning for other people trying to solve the same problem.
– Agost Biro
Aug 23 at 10:55
@AgostBiro I appreciate your comment. It is just that my assumptions met expectations of OP. Thank you.
– Sandeep Kadapa
Aug 23 at 10:58
Using List comprehension
with zip
:
List comprehension
zip
>>> [(x[0], x[1]+y[1]) for x,y in zip(list1, list2)]
#driver values :
IN : list1 = [(1533945600000, 140), (1534032000000, 412), (1534118400000, 364), (1534204800000, 488), (1534291200000, 272), (1534377600000, 350), (1534464000000, 301), (1534550400000, 159), (1534636800000, 224), (1534723200000, 241), (1534809600000, 223), (1534896000000, 175)]
list2 = [(1533945600000, 1516), (1534032000000, 2176), (1534118400000, 2046), (1534204800000, 2400), (1534291200000, 8370), (1534377600000, 2112), (1534464000000, 1441), (1534550400000, 784), (1534636800000, 1391), (1534723200000, 1178), (1534809600000, 1020), (1534896000000, 795)]
OUT : [(1533945600000, 1656), (1534032000000, 2588), (1534118400000, 2410), (1534204800000, 2888), (1534291200000, 8642), (1534377600000, 2462), (1534464000000, 1742), (1534550400000, 943), (1534636800000, 1615), (1534723200000, 1419), (1534809600000, 1243), (1534896000000, 970)]
combined_list=
for i,j in zip(list1,list2):
combined_list.append((i[0]+j[0],i[1]+j[1]))
You can use the following snippet if the lists are not guaranteed to have the same length or the keys are not guaranteed to be present in both lists or they are not in the same order:
from collections import defaultdict
list1 = [(1533945600000, 140), (1534032000000, 412), (1534118400000, 364), (1534204800000, 488), (1534291200000, 272), (1534377600000, 350), (1534464000000, 301), (1534550400000, 159), (1534636800000, 224), (1534723200000, 241), (1534809600000, 223), (1534896000000, 175)]
list2 = [(1533945600000, 1516), (1534032000000, 2176), (1534118400000, 2046), (1534204800000, 2400), (1534291200000, 8370), (1534377600000, 2112), (1534464000000, 1441), (1534550400000, 784), (1534636800000, 1391), (1534723200000, 1178), (1534809600000, 1020), (1534896000000, 795)]
d = defaultdict(int, list1)
for key, n in list2:
d[key] += n
res = list(map(tuple, d.items()))
res:
[(1533945600000, 1656),
(1534032000000, 2588),
(1534118400000, 2410),
(1534204800000, 2888),
(1534291200000, 8642),
(1534377600000, 2462),
(1534464000000, 1742),
(1534550400000, 943),
(1534636800000, 1615),
(1534723200000, 1419),
(1534809600000, 1243),
(1534896000000, 970)]
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Can you explain more? Not exactly sure if you just want to combine the two lists or something more.
– akash12300
Aug 23 at 10:40