Generate list of items with 0 prefix using padStart
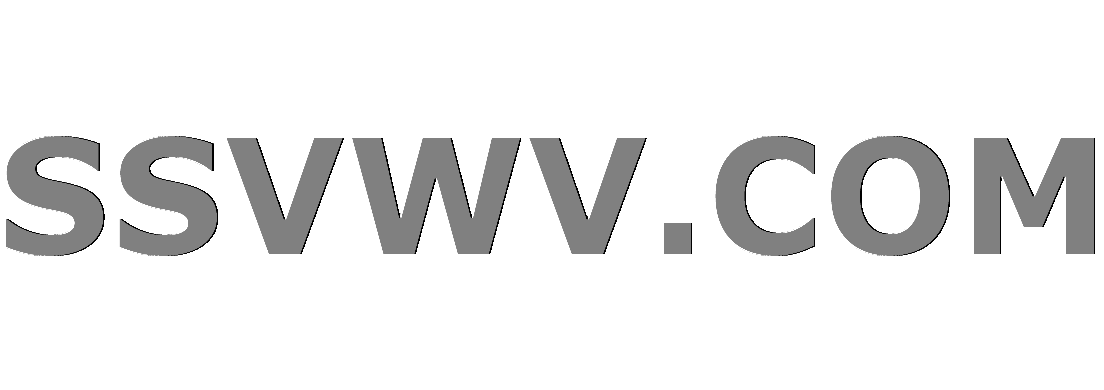
Multi tool use
Generate list of items with 0 prefix using padStart
I implemented the way to generate a list of items with iterable counts with prefix 0. What is the best way to generate such kind of list?
Current behaviour:
const generateList = (length, n, i) =>
let b = n+i
return b.toString().padStart(length.toString().length + n.toString.length, 0)
Array(10).fill(null).map((x, i) => generateList(10,2, i))
Output result:
["002", "003", "004", "005", "006", "007", "008", "009", "010", "011"]
Do u have any idea to make it another way?
You can use Math.floor((length+n)/)+2 , 0 inside padStart
– cemsina güzel
Sep 10 '18 at 13:35
I don't think you meant
n.toString.length
which is 1
for any number n
.– Bergi
Sep 10 '18 at 13:43
n.toString.length
1
n
Is the current output the expected output?
– Bergi
Sep 10 '18 at 13:43
stackoverflow.com/questions/1267283/… stackoverflow.com/questions/10073699/…
– epascarello
Sep 10 '18 at 13:46
3 Answers
3
You could determine the number of characters needed at the start and used the predetermined value to format the output for the array.
function createList(startValue, endValue)
let
// The minimum output length, for a single digit number, is 2 chars.
outputLength = 2,
testValue = 10,
// Create an empty array which has as many items as numbers we need to
// generate for the output. Add 1 to the end value as this is to be
// inclusive of the range to create. If the +1 is not done the resulting
// array is 1 item too small.
emptyArray = Array(endValue - startValue + 1);
// As long as test value is less than the end value, keep increasing the
// output size by 1 and continue to the next multiple of 10.
while (testValue <= endValue)
outputLength++;
testValue = testValue * 10;
// Create a new array, with the same length as the empty array created
// earlier. For each position place a padded number into the output array.
return Array.from(emptyArray, (currentValue, index) =>
// Pad the current value to the determined max length.
return (startValue + index).toString().padStart(outputLength, '0');
);
function createListWithLength(length, startValue = 0)
return createList(startValue, startValue + length);
console.log(createList(2,10));
console.log(createListWithLength(30));
console.log(createListWithLength(10, 995));
the parens are redundant here ->
(endValue + 1) - startValue
– marzelin
Sep 10 '18 at 14:28
(endValue + 1) - startValue
and use
Array.from(Array(length), fillerFn)
instead filling with null
s and then mapping over.– marzelin
Sep 10 '18 at 14:29
Array.from(Array(length), fillerFn)
null
Yeah, I have a things with adding parenthesis where not absolutely necessary. I guess I find it easier to read. I'd actually prefer a good old
for
-loop, have the feeling it would give better performance.– Thijs
Sep 10 '18 at 14:31
for
Didn't know about that second param for the
Array.from
method, thanks!– Thijs
Sep 10 '18 at 14:32
Array.from
I prefer
for..of
cause it can work with iterables (i.e. node lists)– marzelin
Sep 10 '18 at 14:32
for..of
Have a look at generators:
function* range(from, to)
for (var i=from; i<to; i++)
yield i;
function* paddedRange(from, to)
const length = (to-1).toString(10) + 1 /* at least one pad */;
for (const i of range(from, to))
yield i.padStart(length, '0');
console.log(Array.from(paddedRange(2, 12)));
You can also inline the loop from range
into paddedRange
, or you can make it return an array directly:
range
paddedRange
function paddedRange(from, to)
const length = (to-1).toString(10) + 1 /* at least one pad */;
return Array.from(range(from, to), i => i.padStart(length, '0'));
console.log(paddedRange(2, 12));
The main simplification is that you should compute the padding length only once and give it a denotative name, instead of computing it for every number again. Also ranges are usually given by their lower and upper end instead of their begin and a length, but you can easily switch back if you need the latter for some reason.
generators, nice. But I see also good old
var
still used ;)– marzelin
Sep 10 '18 at 14:39
var
@marzelin There's no advantage in using
let
here, as we don't need a block scope.– Bergi
Sep 10 '18 at 14:40
let
so you prefer
var
over let
, and use let
only when needed?– marzelin
Sep 10 '18 at 14:42
var
let
let
@marzelin I tend use
var
for all function-scoped variables, and let
when I need a block scope for a closure or don't want to use the variable after the loop. If it doesn't matter (like here) I'll flip a coin or check which side of the bed I got up today :-)– Bergi
Sep 10 '18 at 14:45
var
let
haha, I prefer being concise in this matter ;)
– marzelin
Sep 10 '18 at 14:48
Not sure, but maybe something like this
const generateList = length => Array(length).fill('0').map((item, index) => item + index);
console.log(generateList(20));
That only work for lists up to and including 9…
– deceze♦
Sep 10 '18 at 13:26
Thanks for contributing an answer to Stack Overflow!
But avoid …
To learn more, see our tips on writing great answers.
Required, but never shown
Required, but never shown
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
codereview.stackexchange.com?
– deceze♦
Sep 10 '18 at 13:14