Form Validation- html and javascript
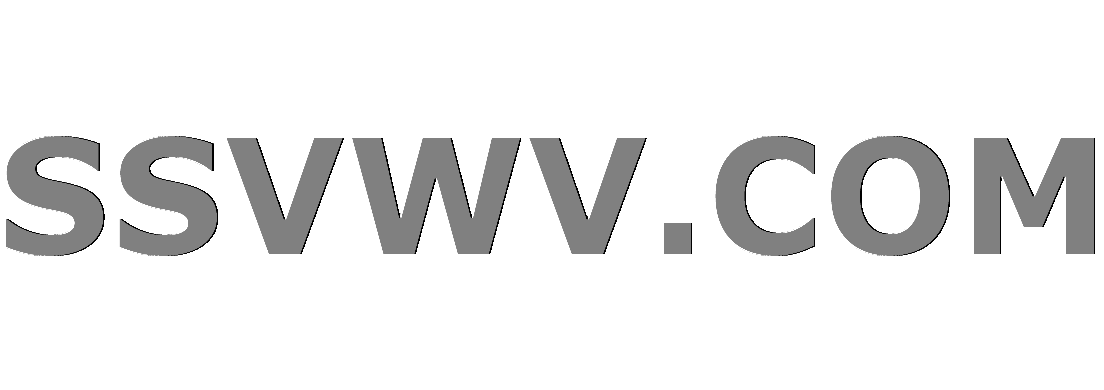
Multi tool use
Form Validation- html and javascript
I'm relatively new to coding- working on form validation and can't get the page to actually return false (not submit the form if the user inputs the incorrect isbn length) with my formValidation function. Wondering what I'm missing here.
The alert pops up and form is submitted no matter what the isbn.length is- however, if it's the correct length, it does get added to the database and is rerouted to the home page. If it's not the right length, it's routed to
"This page isn’t working
localhost didn’t send any data.
ERR_EMPTY_RESPONSE"
Javascript:
//function to validate the form's ISBN number input and ensure it's between 10-14 digits.
function formValidation()
var isbn = document.forms["sellForm"]["isbn"];
if (isbn.length >= 10 && isbn.length <= 14)
return true;
else
alert("Please input a 10-14 digit ISBN number");
isbn.focus();
return false;
</script>
corresponding HTML:
<form name="sellForm" method="POST" action="/create" role="form" onsubmit="formValidation()">
<div class="form-group">
<label for="role" >ISBN</label>
<input type="number" size=14 class="form-control" name="isbn" id="role" required
placeholder="input the 10-14 digit ISBN number"/>
</div>
<div class="form-group">
<label for="age">Condition</label>
<br>
<input type="radio" name="book_condition" value="Very Used"> Very Used<br>
<input type="radio" name="book_condition" value="Lightly Used"> Lightly Used<br>
<input type="radio" name="book_condition" value="Like New"> Like New
</div>
</div>
<div class="form-group">
<label>Price</label>
<input type="number" class="form-control" name="price" required
placeholder="input whole dollar price">
</div>
<button type="submit" class="btn btn-primary btn-md" id="add-btn">
<span class="fa fa-fire"></span> Sell</button>
</form>
What does "can't get the page to actual return false" mean?
– Andy Ray
Sep 5 '18 at 0:08
What does
console.log(isbn)
tell you when placed right after you set that variable? Is it defined? Also, "10-12 digits", or "10-14"? Alert msg says one, code & placeholder text say the other.– mc01
Sep 5 '18 at 0:11
console.log(isbn)
it should be 10-14. checking console.log(isbn)
– Kelly Hook
Sep 5 '18 at 0:14
a. you should change
var isbn = document.forms["sellForm"]["isbn"];
to var isbn = document.forms["sellForm"]["isbn"].value;
b. and, you should debug the isbn
value. in web browser, hit f12 to go to "Console tab", type like console.log(isbn)
. c. onsubmit="return formValidation()"
.– WhitehorseJJ
Sep 5 '18 at 0:21
var isbn = document.forms["sellForm"]["isbn"];
var isbn = document.forms["sellForm"]["isbn"].value;
isbn
console.log(isbn)
onsubmit="return formValidation()"
3 Answers
3
You have other issues (see Andre's answer) but you need to add return
as part of the onsubmit
attribute
return
onsubmit
<form name="sellForm" method="POST" action="/create" role="form" onsubmit="return formValidation();">
The Full Version
function formValidation()
/*This is the form element*/
var isbnEl = document.forms["sellForm"]["isbn"];
var isbn = isbnEl.value;
/*The following 2 lines are for demonstration only and can be removed*/
console.log(isbnEl);
console.log(isbn);
if (isbn.length >= 10 && isbn.length <= 14)
return true;
else
alert("Please input a 10-12 digit ISBN number");
isbnEl.focus();
return false;
<form name="sellForm" method="POST" action="/create" role="form" onsubmit="return formValidation()">
<div class="form-group">
<label for="role">ISBN</label>
<input type="number" size=14 class="form-control" name="isbn" id="role" required placeholder="input the 10-14 digit ISBN number" />
</div>
<div class="form-group">
<label for="age">Condition</label>
<br>
<input type="radio" name="book_condition" value="Very Used"> Very Used<br>
<input type="radio" name="book_condition" value="Lightly Used"> Lightly Used<br>
<input type="radio" name="book_condition" value="Like New"> Like New
</div>
<!--</div> <---- This is an orphan tag, remove it -->
<div class="form-group">
<label>Price</label>
<input type="number" class="form-control" name="price" required placeholder="input whole dollar price">
</div>
<button type="submit" class="btn btn-primary btn-md" id="add-btn">
<span class="fa fa-fire"></span> Sell</button>
</form>
awesome- thank you!
– Kelly Hook
Sep 5 '18 at 2:24
You might need to check isbn.value like this:
function formValidation()
var isbn = document.forms["sellForm"]["isbn"];
if (isbn.value >= 10 && isbn.value <= 14)
return true;
else
alert("Please input a 10-12 digit ISBN number");
isbn.focus();
return false;
Then add the logic as desired.
I think he is not checking the value but the length if the length is between 10 and 14.
– null
Sep 5 '18 at 0:20
The html control is a numeric control, base on the isbn number, I think is not the length what was trying to check.
– Andre Gamboa
Sep 5 '18 at 0:23
isbn.value doesn't work- also checking length
– Kelly Hook
Sep 5 '18 at 0:24
It works for me, could you explain more what you are trying to accomplish in order to send you a full example.
– Andre Gamboa
Sep 5 '18 at 0:27
@AndreGamboa Your answer seems to be incorrect. You must check the value's length like this:
isbn.value.length
. See my answer it contains a runnable snippet.– ths
Sep 5 '18 at 0:39
isbn.value.length
You need to check for the isbn
input field value's length, and in the form tag you need to get the formValidation
function's return in the onsubmit
handler like this: onsubmit="return formValidation()"
.
isbn
formValidation
onsubmit
onsubmit="return formValidation()"
function formValidation()
var isbn = document.forms["sellForm"]["isbn"];
// check for the input field value's length
if (isbn.value.length >= 10 && isbn.value.length <= 14)
return true;
else
alert("Please input a 10-12 digit ISBN number");
isbn.focus();
return false;
<form name="sellForm" method="POST" action="/create" role="form" onsubmit="return formValidation()">
<div class="form-group">
<label for="role" >ISBN</label>
<input type="number" size=14 class="form-control" name="isbn" id="role" required
placeholder="input the 10-14 digit ISBN number"/>
</div>
<div class="form-group">
<label for="age">Condition</label>
<br>
<input type="radio" name="book_condition" value="Very Used"> Very Used<br>
<input type="radio" name="book_condition" value="Lightly Used"> Lightly Used<br>
<input type="radio" name="book_condition" value="Like New"> Like New
</div>
</div>
<div class="form-group">
<label>Price</label>
<input type="number" class="form-control" name="price" required
placeholder="input whole dollar price">
</div>
<button type="submit" class="btn btn-primary btn-md" id="add-btn">
<span class="fa fa-fire"></span> Sell</button>
</form>
That's same with me.
– WhitehorseJJ
Sep 5 '18 at 0:27
@WhitehorseJJ what ? I didn't get it ?!
– ths
Sep 5 '18 at 0:29
Don't worry. it doesn't mean. ths.
– WhitehorseJJ
Sep 5 '18 at 0:31
@WhitehorseJJ what are you trying to say ?
– ths
Sep 5 '18 at 0:32
That's nothing. you're very quick and intelligent.
– WhitehorseJJ
Sep 5 '18 at 0:34
Thanks for contributing an answer to Stack Overflow!
But avoid …
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
But avoid …
To learn more, see our tips on writing great answers.
Required, but never shown
Required, but never shown
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Do you hit the alert? Any console errors (hit F12 to bring up browser tools then go to the "Console" tab)?
– Jon P
Sep 5 '18 at 0:05