Fire Rate Issue in Java 2D Gaming
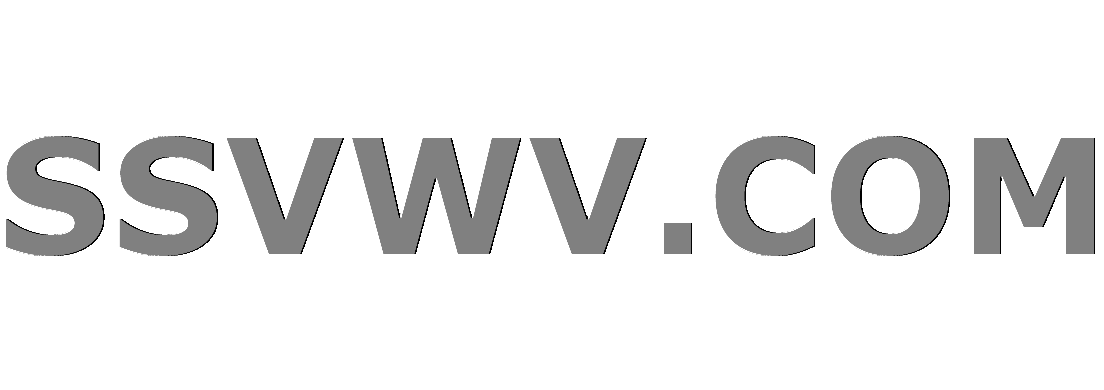
Multi tool use
I am creating a 2D tank game and I want my tank to be able to shoot immediately once the fire button is pressed and then again every half second, while the fire button is held. Currently In my game, my first bullet shoots immediately after the fire button is pressed, then there is a delay (I presume half second) until my tank starts shooting a stream of bullets. I'm wondering why the initial delay after the first bullet works, but the successive ones fail. Below I have included the method which creates bullets which is called every frame in my 144Hz main game loop:
public void addBullets(ArrayList<Animate> animates, ArrayList<Drawable> drawables)
if (this.ShootPressed)
if( firstShot
Here are the associated methods in my KeyListener class:
public void keyPressed(KeyEvent key) {
int keyPressed = key.getKeyCode();
if (keyPressed == up)
this.t1.toggleUpPressed();
if (keyPressed == down)
this.t1.toggleDownPressed();
if (keyPressed == left)
this.t1.toggleLeftPressed();
if (keyPressed == right)
this.t1.toggleRightPressed();
if(keyPressed == shoot)
this.t1.toggleShootPressed();
this.t1.setFirstShot(true);
I am including this for additional information even though the bug happens before the key is released:
public void keyReleased(KeyEvent ke)
int keyReleased = ke.getKeyCode();
if (keyReleased == up)
this.t1.unToggleUpPressed();
if (keyReleased == down)
this.t1.unToggleDownPressed();
if (keyReleased == left)
this.t1.unToggleLeftPressed();
if (keyReleased == right)
this.t1.unToggleRightPressed();
if (keyReleased == shoot)
this.t1.unToggleShootPressed();
java 2d-games
add a comment |
I am creating a 2D tank game and I want my tank to be able to shoot immediately once the fire button is pressed and then again every half second, while the fire button is held. Currently In my game, my first bullet shoots immediately after the fire button is pressed, then there is a delay (I presume half second) until my tank starts shooting a stream of bullets. I'm wondering why the initial delay after the first bullet works, but the successive ones fail. Below I have included the method which creates bullets which is called every frame in my 144Hz main game loop:
public void addBullets(ArrayList<Animate> animates, ArrayList<Drawable> drawables)
if (this.ShootPressed)
if( firstShot
Here are the associated methods in my KeyListener class:
public void keyPressed(KeyEvent key) {
int keyPressed = key.getKeyCode();
if (keyPressed == up)
this.t1.toggleUpPressed();
if (keyPressed == down)
this.t1.toggleDownPressed();
if (keyPressed == left)
this.t1.toggleLeftPressed();
if (keyPressed == right)
this.t1.toggleRightPressed();
if(keyPressed == shoot)
this.t1.toggleShootPressed();
this.t1.setFirstShot(true);
I am including this for additional information even though the bug happens before the key is released:
public void keyReleased(KeyEvent ke)
int keyReleased = ke.getKeyCode();
if (keyReleased == up)
this.t1.unToggleUpPressed();
if (keyReleased == down)
this.t1.unToggleDownPressed();
if (keyReleased == left)
this.t1.unToggleLeftPressed();
if (keyReleased == right)
this.t1.unToggleRightPressed();
if (keyReleased == shoot)
this.t1.unToggleShootPressed();
java 2d-games
add a comment |
I am creating a 2D tank game and I want my tank to be able to shoot immediately once the fire button is pressed and then again every half second, while the fire button is held. Currently In my game, my first bullet shoots immediately after the fire button is pressed, then there is a delay (I presume half second) until my tank starts shooting a stream of bullets. I'm wondering why the initial delay after the first bullet works, but the successive ones fail. Below I have included the method which creates bullets which is called every frame in my 144Hz main game loop:
public void addBullets(ArrayList<Animate> animates, ArrayList<Drawable> drawables)
if (this.ShootPressed)
if( firstShot
Here are the associated methods in my KeyListener class:
public void keyPressed(KeyEvent key) {
int keyPressed = key.getKeyCode();
if (keyPressed == up)
this.t1.toggleUpPressed();
if (keyPressed == down)
this.t1.toggleDownPressed();
if (keyPressed == left)
this.t1.toggleLeftPressed();
if (keyPressed == right)
this.t1.toggleRightPressed();
if(keyPressed == shoot)
this.t1.toggleShootPressed();
this.t1.setFirstShot(true);
I am including this for additional information even though the bug happens before the key is released:
public void keyReleased(KeyEvent ke)
int keyReleased = ke.getKeyCode();
if (keyReleased == up)
this.t1.unToggleUpPressed();
if (keyReleased == down)
this.t1.unToggleDownPressed();
if (keyReleased == left)
this.t1.unToggleLeftPressed();
if (keyReleased == right)
this.t1.unToggleRightPressed();
if (keyReleased == shoot)
this.t1.unToggleShootPressed();
java 2d-games
I am creating a 2D tank game and I want my tank to be able to shoot immediately once the fire button is pressed and then again every half second, while the fire button is held. Currently In my game, my first bullet shoots immediately after the fire button is pressed, then there is a delay (I presume half second) until my tank starts shooting a stream of bullets. I'm wondering why the initial delay after the first bullet works, but the successive ones fail. Below I have included the method which creates bullets which is called every frame in my 144Hz main game loop:
public void addBullets(ArrayList<Animate> animates, ArrayList<Drawable> drawables)
if (this.ShootPressed)
if( firstShot
Here are the associated methods in my KeyListener class:
public void keyPressed(KeyEvent key) {
int keyPressed = key.getKeyCode();
if (keyPressed == up)
this.t1.toggleUpPressed();
if (keyPressed == down)
this.t1.toggleDownPressed();
if (keyPressed == left)
this.t1.toggleLeftPressed();
if (keyPressed == right)
this.t1.toggleRightPressed();
if(keyPressed == shoot)
this.t1.toggleShootPressed();
this.t1.setFirstShot(true);
I am including this for additional information even though the bug happens before the key is released:
public void keyReleased(KeyEvent ke)
int keyReleased = ke.getKeyCode();
if (keyReleased == up)
this.t1.unToggleUpPressed();
if (keyReleased == down)
this.t1.unToggleDownPressed();
if (keyReleased == left)
this.t1.unToggleLeftPressed();
if (keyReleased == right)
this.t1.unToggleRightPressed();
if (keyReleased == shoot)
this.t1.unToggleShootPressed();
java 2d-games
java 2d-games
asked Nov 10 '18 at 9:57
yungvinsantos
82
82
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I suspect it's an issue with key-repeats which will repeatedly call KeyPressed while the key is held. It is usually setup to behave as you describe. Consequently, "firstShot" will be repeatedly set to true and a shot will be fired.
I advise restricting your event code, mainly as it's continuous in nature, to only toggling actions, rather than performing any logic. You can determine whether a shot is first or not in your game loop, with the help of some messages from your events.
However, the firstShot variable is not really necessary at all as the time delta will account for it. Removing it would also prevent firing faster than every 500ms by pressing the fire key rapidly, which you may or may not want.
yeah, I wasn't sure if the keypressed routine was being continuously called while holding down the key, but I learned that it was. the firstShot variable was as you said. It was not necessary at all and in fact, it was the root of my bug! thank you for the advice.
– yungvinsantos
Nov 13 '18 at 22:54
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53237825%2ffire-rate-issue-in-java-2d-gaming%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I suspect it's an issue with key-repeats which will repeatedly call KeyPressed while the key is held. It is usually setup to behave as you describe. Consequently, "firstShot" will be repeatedly set to true and a shot will be fired.
I advise restricting your event code, mainly as it's continuous in nature, to only toggling actions, rather than performing any logic. You can determine whether a shot is first or not in your game loop, with the help of some messages from your events.
However, the firstShot variable is not really necessary at all as the time delta will account for it. Removing it would also prevent firing faster than every 500ms by pressing the fire key rapidly, which you may or may not want.
yeah, I wasn't sure if the keypressed routine was being continuously called while holding down the key, but I learned that it was. the firstShot variable was as you said. It was not necessary at all and in fact, it was the root of my bug! thank you for the advice.
– yungvinsantos
Nov 13 '18 at 22:54
add a comment |
I suspect it's an issue with key-repeats which will repeatedly call KeyPressed while the key is held. It is usually setup to behave as you describe. Consequently, "firstShot" will be repeatedly set to true and a shot will be fired.
I advise restricting your event code, mainly as it's continuous in nature, to only toggling actions, rather than performing any logic. You can determine whether a shot is first or not in your game loop, with the help of some messages from your events.
However, the firstShot variable is not really necessary at all as the time delta will account for it. Removing it would also prevent firing faster than every 500ms by pressing the fire key rapidly, which you may or may not want.
yeah, I wasn't sure if the keypressed routine was being continuously called while holding down the key, but I learned that it was. the firstShot variable was as you said. It was not necessary at all and in fact, it was the root of my bug! thank you for the advice.
– yungvinsantos
Nov 13 '18 at 22:54
add a comment |
I suspect it's an issue with key-repeats which will repeatedly call KeyPressed while the key is held. It is usually setup to behave as you describe. Consequently, "firstShot" will be repeatedly set to true and a shot will be fired.
I advise restricting your event code, mainly as it's continuous in nature, to only toggling actions, rather than performing any logic. You can determine whether a shot is first or not in your game loop, with the help of some messages from your events.
However, the firstShot variable is not really necessary at all as the time delta will account for it. Removing it would also prevent firing faster than every 500ms by pressing the fire key rapidly, which you may or may not want.
I suspect it's an issue with key-repeats which will repeatedly call KeyPressed while the key is held. It is usually setup to behave as you describe. Consequently, "firstShot" will be repeatedly set to true and a shot will be fired.
I advise restricting your event code, mainly as it's continuous in nature, to only toggling actions, rather than performing any logic. You can determine whether a shot is first or not in your game loop, with the help of some messages from your events.
However, the firstShot variable is not really necessary at all as the time delta will account for it. Removing it would also prevent firing faster than every 500ms by pressing the fire key rapidly, which you may or may not want.
edited Nov 10 '18 at 13:14
answered Nov 10 '18 at 13:02
Hayden Ness
161
161
yeah, I wasn't sure if the keypressed routine was being continuously called while holding down the key, but I learned that it was. the firstShot variable was as you said. It was not necessary at all and in fact, it was the root of my bug! thank you for the advice.
– yungvinsantos
Nov 13 '18 at 22:54
add a comment |
yeah, I wasn't sure if the keypressed routine was being continuously called while holding down the key, but I learned that it was. the firstShot variable was as you said. It was not necessary at all and in fact, it was the root of my bug! thank you for the advice.
– yungvinsantos
Nov 13 '18 at 22:54
yeah, I wasn't sure if the keypressed routine was being continuously called while holding down the key, but I learned that it was. the firstShot variable was as you said. It was not necessary at all and in fact, it was the root of my bug! thank you for the advice.
– yungvinsantos
Nov 13 '18 at 22:54
yeah, I wasn't sure if the keypressed routine was being continuously called while holding down the key, but I learned that it was. the firstShot variable was as you said. It was not necessary at all and in fact, it was the root of my bug! thank you for the advice.
– yungvinsantos
Nov 13 '18 at 22:54
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53237825%2ffire-rate-issue-in-java-2d-gaming%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ReygvlK1AahbLiTYLeu,Xvb,8WAJ3iO9a9ts3MlsK g9 0cWsAtrh3717aVXmW7Gi2hci9xzCkDXe Q