PHP Decrypt data (encrypted with mcrypt) using openssl
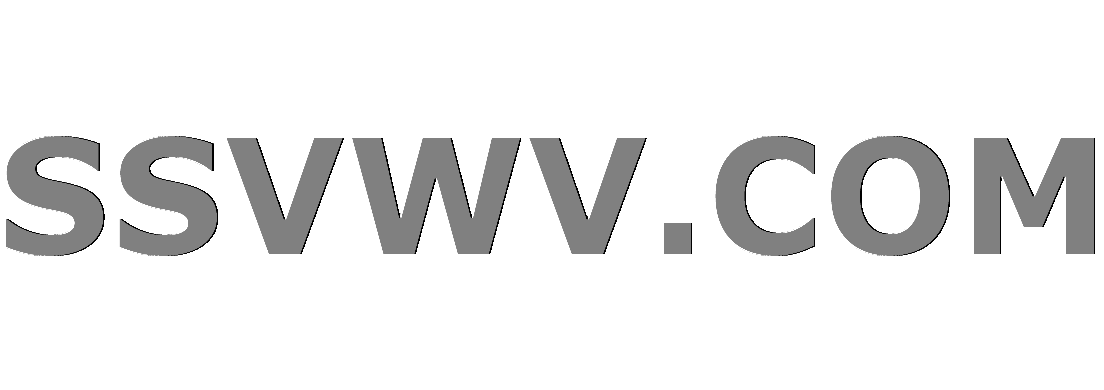
Multi tool use
up vote
-2
down vote
favorite
I have a database full of data encrypted in 3DES (ECB) that was encrypted with the php Mcrypt library. Since Mcrypt is depreacated, I need to switch to OpenSSL to decrypt it. All the data will be reencrypted using xchacha20-poly1305-ietf.
So I don't need comments about 3DES not being secure and ECB bad etc, we know, this is why we are trying to decrypt to have a better encryption algorithm.
I am providing below the code that was used to encrypt using mcrypt and the 1 line we are trying to use (openssl) to decrypt it. It always returns false and we are wondering why.
I am starting to suspect the problem is with the mcrypt library using an 8 bytes IV while open SSL saying it has to be 0 bytes.
Any help would be appreciated to find a way to decrypt the values using openssl.
Thanks in advance.
Here is the code:
$sEncryptionKey = 'aaaabbbbccccddddeeeeffff';
$sDataToEncrypt = 'Foo bar';
echo "Data to be Encrypted: $sDataToEncryptn";
$rMcrypt = mcrypt_module_open(MCRYPT_3DES, '', MCRYPT_MODE_ECB, '');
$iIvSize = mcrypt_enc_get_iv_size($rMcrypt); //This gives 8 bytes
$sInitializationVector = mcrypt_create_iv($iIvSize, MCRYPT_RAND);
$iKeySize = mcrypt_enc_get_key_size($rMcrypt);
if ($iKeySize != strlen($sEncryptionKey))
throw new Exception ('Invalid key length: '.$iKeySize);
mcrypt_generic_init($rMcrypt, $sEncryptionKey, $sInitializationVector);
$sEncryptedString = base64_encode(mcrypt_generic($rMcrypt, $sDataToEncrypt));
echo "Data Encrypted: $sEncryptedStringn";
$sDecryptedString = trim(mdecrypt_generic($rMcrypt, base64_decode($sEncryptedString)));
echo "Data Decrypted: $sDecryptedStringn";
mcrypt_generic_deinit($rMcrypt);
mcrypt_module_close($rMcrypt);
$sDecryptedString2 = openssl_decrypt(base64_decode($sEncryptedString), 'des-ede3', $sEncryptionKey, 0, ''); //this returns false.
echo "Data Decrypted (open SSL): $sDecryptedString2n";
$sDecryptedString2 = openssl_decrypt(base64_decode($sEncryptedString), 'des-ede3', $sEncryptionKey, 0, $sInitializationVector); //Warning: openssl_decrypt(): IV passed is 8 bytes long which is longer than the 0 expected by selected cipher, truncating
?>
The output of the program shows:
Data to be Encrypted: Foo bar
Data Encrypted: 5Mraf9swmaI=
Data Decrypted: Foo bar
Data Decrypted (open SSL):
Warning: openssl_decrypt(): IV passed is 8 bytes long which is longer than the 0 expected by selected cipher, truncating in /usr/local/www/appcluster01.ezmax.ca/pub/web/test/ian/test.cmd on line 31
php openssl mcrypt 3des ecb
add a comment |
up vote
-2
down vote
favorite
I have a database full of data encrypted in 3DES (ECB) that was encrypted with the php Mcrypt library. Since Mcrypt is depreacated, I need to switch to OpenSSL to decrypt it. All the data will be reencrypted using xchacha20-poly1305-ietf.
So I don't need comments about 3DES not being secure and ECB bad etc, we know, this is why we are trying to decrypt to have a better encryption algorithm.
I am providing below the code that was used to encrypt using mcrypt and the 1 line we are trying to use (openssl) to decrypt it. It always returns false and we are wondering why.
I am starting to suspect the problem is with the mcrypt library using an 8 bytes IV while open SSL saying it has to be 0 bytes.
Any help would be appreciated to find a way to decrypt the values using openssl.
Thanks in advance.
Here is the code:
$sEncryptionKey = 'aaaabbbbccccddddeeeeffff';
$sDataToEncrypt = 'Foo bar';
echo "Data to be Encrypted: $sDataToEncryptn";
$rMcrypt = mcrypt_module_open(MCRYPT_3DES, '', MCRYPT_MODE_ECB, '');
$iIvSize = mcrypt_enc_get_iv_size($rMcrypt); //This gives 8 bytes
$sInitializationVector = mcrypt_create_iv($iIvSize, MCRYPT_RAND);
$iKeySize = mcrypt_enc_get_key_size($rMcrypt);
if ($iKeySize != strlen($sEncryptionKey))
throw new Exception ('Invalid key length: '.$iKeySize);
mcrypt_generic_init($rMcrypt, $sEncryptionKey, $sInitializationVector);
$sEncryptedString = base64_encode(mcrypt_generic($rMcrypt, $sDataToEncrypt));
echo "Data Encrypted: $sEncryptedStringn";
$sDecryptedString = trim(mdecrypt_generic($rMcrypt, base64_decode($sEncryptedString)));
echo "Data Decrypted: $sDecryptedStringn";
mcrypt_generic_deinit($rMcrypt);
mcrypt_module_close($rMcrypt);
$sDecryptedString2 = openssl_decrypt(base64_decode($sEncryptedString), 'des-ede3', $sEncryptionKey, 0, ''); //this returns false.
echo "Data Decrypted (open SSL): $sDecryptedString2n";
$sDecryptedString2 = openssl_decrypt(base64_decode($sEncryptedString), 'des-ede3', $sEncryptionKey, 0, $sInitializationVector); //Warning: openssl_decrypt(): IV passed is 8 bytes long which is longer than the 0 expected by selected cipher, truncating
?>
The output of the program shows:
Data to be Encrypted: Foo bar
Data Encrypted: 5Mraf9swmaI=
Data Decrypted: Foo bar
Data Decrypted (open SSL):
Warning: openssl_decrypt(): IV passed is 8 bytes long which is longer than the 0 expected by selected cipher, truncating in /usr/local/www/appcluster01.ezmax.ca/pub/web/test/ian/test.cmd on line 31
php openssl mcrypt 3des ecb
Any 2 way symmetric encryption is inherently insecure on a webserver. Because the webserver would also possess the key to decrypt it. If you don't need the data decrypted (often) look at asymmetric encryption. But On to your problem, you have to use the same IV that was used to encrypt it to decrypt it. Beyond that I don't think the 2 are compatible.
– ArtisticPhoenix
Nov 9 at 19:29
Possible duplicate of Decrypt mcrypt with openssl
– ArtisticPhoenix
Nov 9 at 19:34
add a comment |
up vote
-2
down vote
favorite
up vote
-2
down vote
favorite
I have a database full of data encrypted in 3DES (ECB) that was encrypted with the php Mcrypt library. Since Mcrypt is depreacated, I need to switch to OpenSSL to decrypt it. All the data will be reencrypted using xchacha20-poly1305-ietf.
So I don't need comments about 3DES not being secure and ECB bad etc, we know, this is why we are trying to decrypt to have a better encryption algorithm.
I am providing below the code that was used to encrypt using mcrypt and the 1 line we are trying to use (openssl) to decrypt it. It always returns false and we are wondering why.
I am starting to suspect the problem is with the mcrypt library using an 8 bytes IV while open SSL saying it has to be 0 bytes.
Any help would be appreciated to find a way to decrypt the values using openssl.
Thanks in advance.
Here is the code:
$sEncryptionKey = 'aaaabbbbccccddddeeeeffff';
$sDataToEncrypt = 'Foo bar';
echo "Data to be Encrypted: $sDataToEncryptn";
$rMcrypt = mcrypt_module_open(MCRYPT_3DES, '', MCRYPT_MODE_ECB, '');
$iIvSize = mcrypt_enc_get_iv_size($rMcrypt); //This gives 8 bytes
$sInitializationVector = mcrypt_create_iv($iIvSize, MCRYPT_RAND);
$iKeySize = mcrypt_enc_get_key_size($rMcrypt);
if ($iKeySize != strlen($sEncryptionKey))
throw new Exception ('Invalid key length: '.$iKeySize);
mcrypt_generic_init($rMcrypt, $sEncryptionKey, $sInitializationVector);
$sEncryptedString = base64_encode(mcrypt_generic($rMcrypt, $sDataToEncrypt));
echo "Data Encrypted: $sEncryptedStringn";
$sDecryptedString = trim(mdecrypt_generic($rMcrypt, base64_decode($sEncryptedString)));
echo "Data Decrypted: $sDecryptedStringn";
mcrypt_generic_deinit($rMcrypt);
mcrypt_module_close($rMcrypt);
$sDecryptedString2 = openssl_decrypt(base64_decode($sEncryptedString), 'des-ede3', $sEncryptionKey, 0, ''); //this returns false.
echo "Data Decrypted (open SSL): $sDecryptedString2n";
$sDecryptedString2 = openssl_decrypt(base64_decode($sEncryptedString), 'des-ede3', $sEncryptionKey, 0, $sInitializationVector); //Warning: openssl_decrypt(): IV passed is 8 bytes long which is longer than the 0 expected by selected cipher, truncating
?>
The output of the program shows:
Data to be Encrypted: Foo bar
Data Encrypted: 5Mraf9swmaI=
Data Decrypted: Foo bar
Data Decrypted (open SSL):
Warning: openssl_decrypt(): IV passed is 8 bytes long which is longer than the 0 expected by selected cipher, truncating in /usr/local/www/appcluster01.ezmax.ca/pub/web/test/ian/test.cmd on line 31
php openssl mcrypt 3des ecb
I have a database full of data encrypted in 3DES (ECB) that was encrypted with the php Mcrypt library. Since Mcrypt is depreacated, I need to switch to OpenSSL to decrypt it. All the data will be reencrypted using xchacha20-poly1305-ietf.
So I don't need comments about 3DES not being secure and ECB bad etc, we know, this is why we are trying to decrypt to have a better encryption algorithm.
I am providing below the code that was used to encrypt using mcrypt and the 1 line we are trying to use (openssl) to decrypt it. It always returns false and we are wondering why.
I am starting to suspect the problem is with the mcrypt library using an 8 bytes IV while open SSL saying it has to be 0 bytes.
Any help would be appreciated to find a way to decrypt the values using openssl.
Thanks in advance.
Here is the code:
$sEncryptionKey = 'aaaabbbbccccddddeeeeffff';
$sDataToEncrypt = 'Foo bar';
echo "Data to be Encrypted: $sDataToEncryptn";
$rMcrypt = mcrypt_module_open(MCRYPT_3DES, '', MCRYPT_MODE_ECB, '');
$iIvSize = mcrypt_enc_get_iv_size($rMcrypt); //This gives 8 bytes
$sInitializationVector = mcrypt_create_iv($iIvSize, MCRYPT_RAND);
$iKeySize = mcrypt_enc_get_key_size($rMcrypt);
if ($iKeySize != strlen($sEncryptionKey))
throw new Exception ('Invalid key length: '.$iKeySize);
mcrypt_generic_init($rMcrypt, $sEncryptionKey, $sInitializationVector);
$sEncryptedString = base64_encode(mcrypt_generic($rMcrypt, $sDataToEncrypt));
echo "Data Encrypted: $sEncryptedStringn";
$sDecryptedString = trim(mdecrypt_generic($rMcrypt, base64_decode($sEncryptedString)));
echo "Data Decrypted: $sDecryptedStringn";
mcrypt_generic_deinit($rMcrypt);
mcrypt_module_close($rMcrypt);
$sDecryptedString2 = openssl_decrypt(base64_decode($sEncryptedString), 'des-ede3', $sEncryptionKey, 0, ''); //this returns false.
echo "Data Decrypted (open SSL): $sDecryptedString2n";
$sDecryptedString2 = openssl_decrypt(base64_decode($sEncryptedString), 'des-ede3', $sEncryptionKey, 0, $sInitializationVector); //Warning: openssl_decrypt(): IV passed is 8 bytes long which is longer than the 0 expected by selected cipher, truncating
?>
The output of the program shows:
Data to be Encrypted: Foo bar
Data Encrypted: 5Mraf9swmaI=
Data Decrypted: Foo bar
Data Decrypted (open SSL):
Warning: openssl_decrypt(): IV passed is 8 bytes long which is longer than the 0 expected by selected cipher, truncating in /usr/local/www/appcluster01.ezmax.ca/pub/web/test/ian/test.cmd on line 31
php openssl mcrypt 3des ecb
php openssl mcrypt 3des ecb
edited Nov 9 at 19:16
asked Nov 9 at 19:06
Ian Lord
113
113
Any 2 way symmetric encryption is inherently insecure on a webserver. Because the webserver would also possess the key to decrypt it. If you don't need the data decrypted (often) look at asymmetric encryption. But On to your problem, you have to use the same IV that was used to encrypt it to decrypt it. Beyond that I don't think the 2 are compatible.
– ArtisticPhoenix
Nov 9 at 19:29
Possible duplicate of Decrypt mcrypt with openssl
– ArtisticPhoenix
Nov 9 at 19:34
add a comment |
Any 2 way symmetric encryption is inherently insecure on a webserver. Because the webserver would also possess the key to decrypt it. If you don't need the data decrypted (often) look at asymmetric encryption. But On to your problem, you have to use the same IV that was used to encrypt it to decrypt it. Beyond that I don't think the 2 are compatible.
– ArtisticPhoenix
Nov 9 at 19:29
Possible duplicate of Decrypt mcrypt with openssl
– ArtisticPhoenix
Nov 9 at 19:34
Any 2 way symmetric encryption is inherently insecure on a webserver. Because the webserver would also possess the key to decrypt it. If you don't need the data decrypted (often) look at asymmetric encryption. But On to your problem, you have to use the same IV that was used to encrypt it to decrypt it. Beyond that I don't think the 2 are compatible.
– ArtisticPhoenix
Nov 9 at 19:29
Any 2 way symmetric encryption is inherently insecure on a webserver. Because the webserver would also possess the key to decrypt it. If you don't need the data decrypted (often) look at asymmetric encryption. But On to your problem, you have to use the same IV that was used to encrypt it to decrypt it. Beyond that I don't think the 2 are compatible.
– ArtisticPhoenix
Nov 9 at 19:29
Possible duplicate of Decrypt mcrypt with openssl
– ArtisticPhoenix
Nov 9 at 19:34
Possible duplicate of Decrypt mcrypt with openssl
– ArtisticPhoenix
Nov 9 at 19:34
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
I just realized I was using openssl_decrypt incorrectly.
Changing to this works fine:
$sDecryptedString2 = openssl_decrypt(base64_decode($sEncryptedString), 'des-ede3', $sEncryptionKey, OPENSSL_ZERO_PADDING | OPENSSL_RAW_DATA, '');
I hope it will help someone somedays.
Thanks
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53231890%2fphp-decrypt-data-encrypted-with-mcrypt-using-openssl%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
I just realized I was using openssl_decrypt incorrectly.
Changing to this works fine:
$sDecryptedString2 = openssl_decrypt(base64_decode($sEncryptedString), 'des-ede3', $sEncryptionKey, OPENSSL_ZERO_PADDING | OPENSSL_RAW_DATA, '');
I hope it will help someone somedays.
Thanks
add a comment |
up vote
0
down vote
I just realized I was using openssl_decrypt incorrectly.
Changing to this works fine:
$sDecryptedString2 = openssl_decrypt(base64_decode($sEncryptedString), 'des-ede3', $sEncryptionKey, OPENSSL_ZERO_PADDING | OPENSSL_RAW_DATA, '');
I hope it will help someone somedays.
Thanks
add a comment |
up vote
0
down vote
up vote
0
down vote
I just realized I was using openssl_decrypt incorrectly.
Changing to this works fine:
$sDecryptedString2 = openssl_decrypt(base64_decode($sEncryptedString), 'des-ede3', $sEncryptionKey, OPENSSL_ZERO_PADDING | OPENSSL_RAW_DATA, '');
I hope it will help someone somedays.
Thanks
I just realized I was using openssl_decrypt incorrectly.
Changing to this works fine:
$sDecryptedString2 = openssl_decrypt(base64_decode($sEncryptedString), 'des-ede3', $sEncryptionKey, OPENSSL_ZERO_PADDING | OPENSSL_RAW_DATA, '');
I hope it will help someone somedays.
Thanks
edited Nov 9 at 19:55


Funk Forty Niner
80.5k1247100
80.5k1247100
answered Nov 9 at 19:50
Ian Lord
113
113
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53231890%2fphp-decrypt-data-encrypted-with-mcrypt-using-openssl%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
xUu,hCX3FzK1,nZsKkgRyN4kycQzzvIybO1SOuBY,IFgP9vkCLxqdhnl0mslNRROdxz57QGX SykH1NJqpvViuMdatH4N
Any 2 way symmetric encryption is inherently insecure on a webserver. Because the webserver would also possess the key to decrypt it. If you don't need the data decrypted (often) look at asymmetric encryption. But On to your problem, you have to use the same IV that was used to encrypt it to decrypt it. Beyond that I don't think the 2 are compatible.
– ArtisticPhoenix
Nov 9 at 19:29
Possible duplicate of Decrypt mcrypt with openssl
– ArtisticPhoenix
Nov 9 at 19:34