convert array with dict to variables
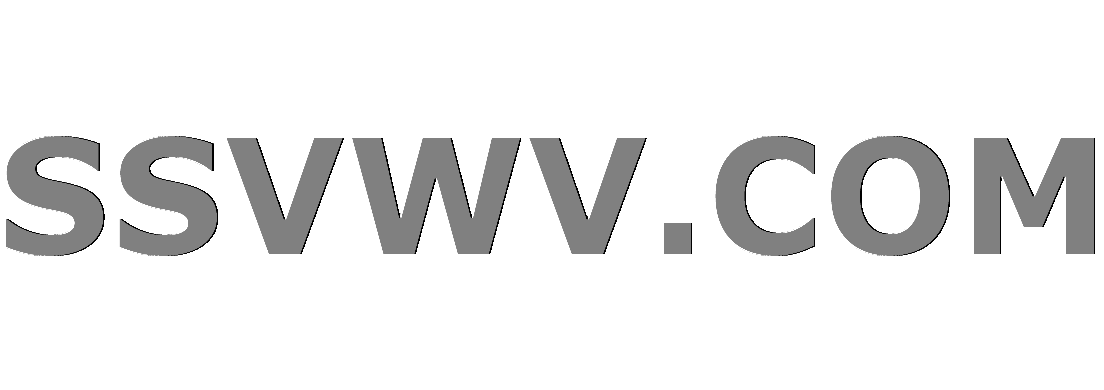
Multi tool use
convert array with dict to variables
I have an array with dict
tag_list = ['Key': 'backup', 'Value': 'true',
'Key': 'backup_daily', 'Value': '7']
I'd like to convert it to variables, such as:
tag_backup = 'true'
tag_backup_daily = '7'
Any easy way to do that?
If no need to convert it to variables, any other better way to reference these key/value directly?
The best I want to do is to reference as below ways.
tag_list['backup'] = "true"
tag_list['backup_daily'] = "7"
Thanks to @Lev Zakharov 's answer, I did with below ways.
>>> new_list = x['Key']: x['Value'] for x in tag_list
>>> new_list['backup']
'true'
>>> new_list['backup_daily']
'7'
3 Answers
3
Use simple dict comprehension:
x['Key']:x['Value'] for x in tag_list
Result:
'backup': 'true', 'backup_daily': '7'
@Bill what do you mean by 'use it by rest codes'?
– Lev Zakharov
Aug 31 at 3:34
I got your point now. You ask to generate a new dict with key/value directly.
new_list = x['Key']:x['Value'] for x in tag_list['TagList']
– Bill
Aug 31 at 3:37
new_list = x['Key']:x['Value'] for x in tag_list['TagList']
You could use globals()
:
globals()
tag_list = ['Key': 'backup', 'Value': 'true',
'Key': 'backup_daily', 'Value': '7']
for x in tag_list:
globals()[f"tag_x['Key']"] = x['Value']
print(tag_backup) # true
print(tag_backup_daily) # 7
What's
f
? Where did you define it?– Frank AK
Aug 31 at 3:43
f
"How to make your code more incomprehensible":) But really interesting solution!
– Lev Zakharov
Aug 31 at 3:44
@FrankAK, it's from Python 3.6
– aydow
Aug 31 at 3:47
@Austin Cool! Good to know it. Thank you.
– Frank AK
Aug 31 at 3:48
@FrankAK, if you using a Python version < 3.6 you can use
globals()['tag_Key'.format(**t)] = t['Value']
– aydow
Aug 31 at 3:54
globals()['tag_Key'.format(**t)] = t['Value']
You just use a for
loop
for
In [184]: def to_dict(tags):
...: d =
...: for t in tags:
...: d[t['Key']] = t['Value']
...: return d
...:
In [185]: to_dict(tag_list)
Out[185]: 'backup': 'true', 'backup_daily': '7'
You could also use map
and lambda
to create a container of tuple
s and then convert to a dict
with its constructor
map
lambda
tuple
dict
In [178]: dict(map(lambda d: (d['Key'], d['Value']), tag_list))
Out[178]: 'backup': 'true', 'backup_daily': '7'
Note, that this is a lot slower due to the dict
constructor
dict
In [207]: %timeit to_dict(tag_list)
The slowest run took 31.74 times longer than the fastest. This could mean that an intermediate result is being cached.
1000000 loops, best of 3: 431 ns per loop
In [181]: %timeit d['Key']: d['Value'] for d in tag_list
The slowest run took 44.42 times longer than the fastest. This could mean that an intermediate result is being cached.
1000000 loops, best of 3: 457 ns per loop
In [182]: %timeit dict(map(lambda d: (d['Key'], d['Value']), tag_list))
The slowest run took 7.72 times longer than the fastest. This could mean that an intermediate result is being cached.
1000000 loops, best of 3: 1.21 µs per loop
Good point with execute time.
– Bill
Aug 31 at 3:52
I think to use this as solution, because I run the codes in
aws lambda
. Less time is better, this is an extra reward I got.– Bill
Aug 31 at 4:02
aws lambda
@Bill But
for
loop solution slower than solution with dict comprehension.– Lev Zakharov
Aug 31 at 4:12
for
I use the lambda one, see my updates in my question. No need
for loop
– Bill
Aug 31 at 4:17
for loop
@Bill yeah, but solution with lambda also slower:) Dict comprehension takes 457 nanoseconds vs 1.21 microseconds!
– Lev Zakharov
Aug 31 at 4:36
Thanks for contributing an answer to Stack Overflow!
But avoid …
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
But avoid …
To learn more, see our tips on writing great answers.
Required, but never shown
Required, but never shown
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
thanks a lot, but how to use it by rest codes?
– Bill
Aug 31 at 3:33