Query to get items based on difference of min,max in group_concat
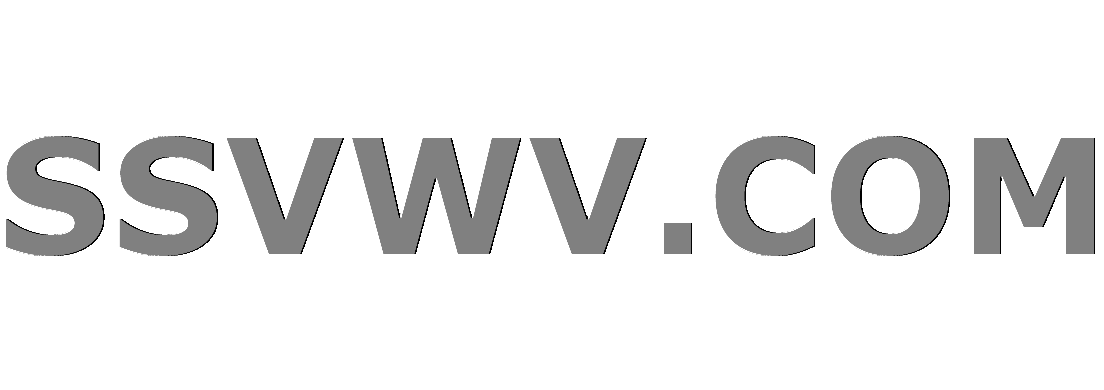
Multi tool use
up vote
0
down vote
favorite
Let's say I have the following entries:
ID Title Year
1 Avatar 2009
2 Avatar 2020
3 Hello 2014
4 Hello 2013
5 New 2017
Here is how I would get each title with all its years:
SELECT title, GROUP_CONCAT(DISTINCT Year) FROM table
And it would give me:
Title Years
Avatar 2009,2020
Hello 2013,2014
New 2017
How would I do a query to get all results where the min(Year) is more than 5 years away from the max(Year). The correct result would then be:
Title Years > 5 Year Diff
Avatar 2009,2020 YES
Hello 2013,2014 NO
New 2017 NO
mysql sql
add a comment |
up vote
0
down vote
favorite
Let's say I have the following entries:
ID Title Year
1 Avatar 2009
2 Avatar 2020
3 Hello 2014
4 Hello 2013
5 New 2017
Here is how I would get each title with all its years:
SELECT title, GROUP_CONCAT(DISTINCT Year) FROM table
And it would give me:
Title Years
Avatar 2009,2020
Hello 2013,2014
New 2017
How would I do a query to get all results where the min(Year) is more than 5 years away from the max(Year). The correct result would then be:
Title Years > 5 Year Diff
Avatar 2009,2020 YES
Hello 2013,2014 NO
New 2017 NO
mysql sql
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Let's say I have the following entries:
ID Title Year
1 Avatar 2009
2 Avatar 2020
3 Hello 2014
4 Hello 2013
5 New 2017
Here is how I would get each title with all its years:
SELECT title, GROUP_CONCAT(DISTINCT Year) FROM table
And it would give me:
Title Years
Avatar 2009,2020
Hello 2013,2014
New 2017
How would I do a query to get all results where the min(Year) is more than 5 years away from the max(Year). The correct result would then be:
Title Years > 5 Year Diff
Avatar 2009,2020 YES
Hello 2013,2014 NO
New 2017 NO
mysql sql
Let's say I have the following entries:
ID Title Year
1 Avatar 2009
2 Avatar 2020
3 Hello 2014
4 Hello 2013
5 New 2017
Here is how I would get each title with all its years:
SELECT title, GROUP_CONCAT(DISTINCT Year) FROM table
And it would give me:
Title Years
Avatar 2009,2020
Hello 2013,2014
New 2017
How would I do a query to get all results where the min(Year) is more than 5 years away from the max(Year). The correct result would then be:
Title Years > 5 Year Diff
Avatar 2009,2020 YES
Hello 2013,2014 NO
New 2017 NO
mysql sql
mysql sql
asked Nov 8 at 22:13
David542
32.2k89245446
32.2k89245446
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
Your first query is wrong. It should be:
SELECT title, GROUP_CONCAT(DISTINCT Year)
FROM table
GROUP BY title;
To implement what you want is simply a CASE
expression:
SELECT title, GROUP_CONCAT(DISTINCT Year),
(CASE WHEN MAX(YEAR) - MIN(YEAR) > 5 THEN 'YES' ELSE 'NO' END) as flag
FROM table
GROUP BY title;
Quite often, such a flag would be encoded as "1" for true and "0" for false. In this case, you would just use the boolean expression for the flag:
SELECT title, GROUP_CONCAT(DISTINCT Year),
(MAX(YEAR) - MIN(YEAR) > 5) as flag
FROM table
GROUP BY title;
Note that this usage is a MySQL extension (but it happens to be one that I like).
Thanks -- the second approach is very elegant and easy to read as well. Thanks for pointing out the error/typo in my query as well.
– David542
Nov 8 at 22:25
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
Your first query is wrong. It should be:
SELECT title, GROUP_CONCAT(DISTINCT Year)
FROM table
GROUP BY title;
To implement what you want is simply a CASE
expression:
SELECT title, GROUP_CONCAT(DISTINCT Year),
(CASE WHEN MAX(YEAR) - MIN(YEAR) > 5 THEN 'YES' ELSE 'NO' END) as flag
FROM table
GROUP BY title;
Quite often, such a flag would be encoded as "1" for true and "0" for false. In this case, you would just use the boolean expression for the flag:
SELECT title, GROUP_CONCAT(DISTINCT Year),
(MAX(YEAR) - MIN(YEAR) > 5) as flag
FROM table
GROUP BY title;
Note that this usage is a MySQL extension (but it happens to be one that I like).
Thanks -- the second approach is very elegant and easy to read as well. Thanks for pointing out the error/typo in my query as well.
– David542
Nov 8 at 22:25
add a comment |
up vote
1
down vote
accepted
Your first query is wrong. It should be:
SELECT title, GROUP_CONCAT(DISTINCT Year)
FROM table
GROUP BY title;
To implement what you want is simply a CASE
expression:
SELECT title, GROUP_CONCAT(DISTINCT Year),
(CASE WHEN MAX(YEAR) - MIN(YEAR) > 5 THEN 'YES' ELSE 'NO' END) as flag
FROM table
GROUP BY title;
Quite often, such a flag would be encoded as "1" for true and "0" for false. In this case, you would just use the boolean expression for the flag:
SELECT title, GROUP_CONCAT(DISTINCT Year),
(MAX(YEAR) - MIN(YEAR) > 5) as flag
FROM table
GROUP BY title;
Note that this usage is a MySQL extension (but it happens to be one that I like).
Thanks -- the second approach is very elegant and easy to read as well. Thanks for pointing out the error/typo in my query as well.
– David542
Nov 8 at 22:25
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
Your first query is wrong. It should be:
SELECT title, GROUP_CONCAT(DISTINCT Year)
FROM table
GROUP BY title;
To implement what you want is simply a CASE
expression:
SELECT title, GROUP_CONCAT(DISTINCT Year),
(CASE WHEN MAX(YEAR) - MIN(YEAR) > 5 THEN 'YES' ELSE 'NO' END) as flag
FROM table
GROUP BY title;
Quite often, such a flag would be encoded as "1" for true and "0" for false. In this case, you would just use the boolean expression for the flag:
SELECT title, GROUP_CONCAT(DISTINCT Year),
(MAX(YEAR) - MIN(YEAR) > 5) as flag
FROM table
GROUP BY title;
Note that this usage is a MySQL extension (but it happens to be one that I like).
Your first query is wrong. It should be:
SELECT title, GROUP_CONCAT(DISTINCT Year)
FROM table
GROUP BY title;
To implement what you want is simply a CASE
expression:
SELECT title, GROUP_CONCAT(DISTINCT Year),
(CASE WHEN MAX(YEAR) - MIN(YEAR) > 5 THEN 'YES' ELSE 'NO' END) as flag
FROM table
GROUP BY title;
Quite often, such a flag would be encoded as "1" for true and "0" for false. In this case, you would just use the boolean expression for the flag:
SELECT title, GROUP_CONCAT(DISTINCT Year),
(MAX(YEAR) - MIN(YEAR) > 5) as flag
FROM table
GROUP BY title;
Note that this usage is a MySQL extension (but it happens to be one that I like).
answered Nov 8 at 22:15
Gordon Linoff
745k32285390
745k32285390
Thanks -- the second approach is very elegant and easy to read as well. Thanks for pointing out the error/typo in my query as well.
– David542
Nov 8 at 22:25
add a comment |
Thanks -- the second approach is very elegant and easy to read as well. Thanks for pointing out the error/typo in my query as well.
– David542
Nov 8 at 22:25
Thanks -- the second approach is very elegant and easy to read as well. Thanks for pointing out the error/typo in my query as well.
– David542
Nov 8 at 22:25
Thanks -- the second approach is very elegant and easy to read as well. Thanks for pointing out the error/typo in my query as well.
– David542
Nov 8 at 22:25
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53216959%2fquery-to-get-items-based-on-difference-of-min-max-in-group-concat%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7qPOWd1oqPXs,ya,fL,yteveLiRhENTdG,t Q51u,n8b11WJdrXSg,do5jy f