Merging keras models before compile or fit?
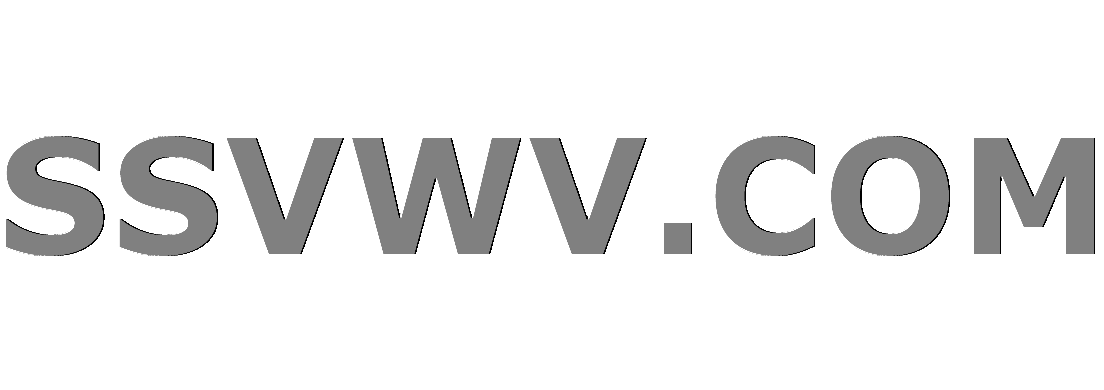
Multi tool use
up vote
0
down vote
favorite
working in Keras 2.2.4
I have three data features (feature1, feature2, feature3) that correspond to a set of users and how they rank certain items. At present, I have modelled them all separately using a model architecture as follows:
input_vecs = add([feature1_vec, user_vec])
nn = Dropout(0.5)(Dense(128, activation='relu')(input_vecs))
nn = BatchNormalization()(nn)
nn = Dropout(0.5)(Dense(128, activation='relu')(nn))
nn = BatchNormalization()(nn)
nn = Dense(128, activation='relu')(nn)
feature1result = Dense(9, activation='softmax')(nn)
feature1model = Model([feature1_input, user_input], feature1result)
featuremodel.compile('adam', 'categorical_crossentropy')
Each of the models have similar architectures (each separately tuned), and the same style/shape of outputs. I would like to take the preliminary result of the three models and put those into a new layer and then create a final result.
I guess I could run the three models separately, take their output, and then put that output into an entirely new (likely Sequential) model using a structure like this:
model1 = load_model("feature1.h5")
model2 = load_model("feature2.h5")
model3 = load_model("feature3.h5")
merged_model = Sequential()
merged_model.add(merge([model1.layers[-1].output,model2.layers[-1].output,model3.layers[-1].output]))
merged_model.add(Dense(units = 9, activation='relu')) #or whatever units
merged_model.add(Dense(units = 12, activation='relu'))#or whatever units
merged_model.add(Dense(9, activation='softmax'))
However, I'd like to merge them beforehand as it will make managing the models easier later on (i.e., only updating one large model instead of updating 4 smaller models).
How can I do this before doing the compile()
and fit()
steps?
python machine-learning keras
add a comment |
up vote
0
down vote
favorite
working in Keras 2.2.4
I have three data features (feature1, feature2, feature3) that correspond to a set of users and how they rank certain items. At present, I have modelled them all separately using a model architecture as follows:
input_vecs = add([feature1_vec, user_vec])
nn = Dropout(0.5)(Dense(128, activation='relu')(input_vecs))
nn = BatchNormalization()(nn)
nn = Dropout(0.5)(Dense(128, activation='relu')(nn))
nn = BatchNormalization()(nn)
nn = Dense(128, activation='relu')(nn)
feature1result = Dense(9, activation='softmax')(nn)
feature1model = Model([feature1_input, user_input], feature1result)
featuremodel.compile('adam', 'categorical_crossentropy')
Each of the models have similar architectures (each separately tuned), and the same style/shape of outputs. I would like to take the preliminary result of the three models and put those into a new layer and then create a final result.
I guess I could run the three models separately, take their output, and then put that output into an entirely new (likely Sequential) model using a structure like this:
model1 = load_model("feature1.h5")
model2 = load_model("feature2.h5")
model3 = load_model("feature3.h5")
merged_model = Sequential()
merged_model.add(merge([model1.layers[-1].output,model2.layers[-1].output,model3.layers[-1].output]))
merged_model.add(Dense(units = 9, activation='relu')) #or whatever units
merged_model.add(Dense(units = 12, activation='relu'))#or whatever units
merged_model.add(Dense(9, activation='softmax'))
However, I'd like to merge them beforehand as it will make managing the models easier later on (i.e., only updating one large model instead of updating 4 smaller models).
How can I do this before doing the compile()
and fit()
steps?
python machine-learning keras
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
working in Keras 2.2.4
I have three data features (feature1, feature2, feature3) that correspond to a set of users and how they rank certain items. At present, I have modelled them all separately using a model architecture as follows:
input_vecs = add([feature1_vec, user_vec])
nn = Dropout(0.5)(Dense(128, activation='relu')(input_vecs))
nn = BatchNormalization()(nn)
nn = Dropout(0.5)(Dense(128, activation='relu')(nn))
nn = BatchNormalization()(nn)
nn = Dense(128, activation='relu')(nn)
feature1result = Dense(9, activation='softmax')(nn)
feature1model = Model([feature1_input, user_input], feature1result)
featuremodel.compile('adam', 'categorical_crossentropy')
Each of the models have similar architectures (each separately tuned), and the same style/shape of outputs. I would like to take the preliminary result of the three models and put those into a new layer and then create a final result.
I guess I could run the three models separately, take their output, and then put that output into an entirely new (likely Sequential) model using a structure like this:
model1 = load_model("feature1.h5")
model2 = load_model("feature2.h5")
model3 = load_model("feature3.h5")
merged_model = Sequential()
merged_model.add(merge([model1.layers[-1].output,model2.layers[-1].output,model3.layers[-1].output]))
merged_model.add(Dense(units = 9, activation='relu')) #or whatever units
merged_model.add(Dense(units = 12, activation='relu'))#or whatever units
merged_model.add(Dense(9, activation='softmax'))
However, I'd like to merge them beforehand as it will make managing the models easier later on (i.e., only updating one large model instead of updating 4 smaller models).
How can I do this before doing the compile()
and fit()
steps?
python machine-learning keras
working in Keras 2.2.4
I have three data features (feature1, feature2, feature3) that correspond to a set of users and how they rank certain items. At present, I have modelled them all separately using a model architecture as follows:
input_vecs = add([feature1_vec, user_vec])
nn = Dropout(0.5)(Dense(128, activation='relu')(input_vecs))
nn = BatchNormalization()(nn)
nn = Dropout(0.5)(Dense(128, activation='relu')(nn))
nn = BatchNormalization()(nn)
nn = Dense(128, activation='relu')(nn)
feature1result = Dense(9, activation='softmax')(nn)
feature1model = Model([feature1_input, user_input], feature1result)
featuremodel.compile('adam', 'categorical_crossentropy')
Each of the models have similar architectures (each separately tuned), and the same style/shape of outputs. I would like to take the preliminary result of the three models and put those into a new layer and then create a final result.
I guess I could run the three models separately, take their output, and then put that output into an entirely new (likely Sequential) model using a structure like this:
model1 = load_model("feature1.h5")
model2 = load_model("feature2.h5")
model3 = load_model("feature3.h5")
merged_model = Sequential()
merged_model.add(merge([model1.layers[-1].output,model2.layers[-1].output,model3.layers[-1].output]))
merged_model.add(Dense(units = 9, activation='relu')) #or whatever units
merged_model.add(Dense(units = 12, activation='relu'))#or whatever units
merged_model.add(Dense(9, activation='softmax'))
However, I'd like to merge them beforehand as it will make managing the models easier later on (i.e., only updating one large model instead of updating 4 smaller models).
How can I do this before doing the compile()
and fit()
steps?
python machine-learning keras
python machine-learning keras
asked 2 days ago
user1563247
9710
9710
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
You need to use the functional API to use the merge layer. I hope this gives you the idea:
input = Input( ... )
model1 = load_model("feature1.h5")
model2 = load_model("feature2.h5")
model3 = load_model("feature3.h5")
m1 = model1(input)
m2 = model2(input)
m3 = model3(input)
merged_model = merge([m1,m2,m3])
...
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
You need to use the functional API to use the merge layer. I hope this gives you the idea:
input = Input( ... )
model1 = load_model("feature1.h5")
model2 = load_model("feature2.h5")
model3 = load_model("feature3.h5")
m1 = model1(input)
m2 = model2(input)
m3 = model3(input)
merged_model = merge([m1,m2,m3])
...
add a comment |
up vote
1
down vote
accepted
You need to use the functional API to use the merge layer. I hope this gives you the idea:
input = Input( ... )
model1 = load_model("feature1.h5")
model2 = load_model("feature2.h5")
model3 = load_model("feature3.h5")
m1 = model1(input)
m2 = model2(input)
m3 = model3(input)
merged_model = merge([m1,m2,m3])
...
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
You need to use the functional API to use the merge layer. I hope this gives you the idea:
input = Input( ... )
model1 = load_model("feature1.h5")
model2 = load_model("feature2.h5")
model3 = load_model("feature3.h5")
m1 = model1(input)
m2 = model2(input)
m3 = model3(input)
merged_model = merge([m1,m2,m3])
...
You need to use the functional API to use the merge layer. I hope this gives you the idea:
input = Input( ... )
model1 = load_model("feature1.h5")
model2 = load_model("feature2.h5")
model3 = load_model("feature3.h5")
m1 = model1(input)
m2 = model2(input)
m3 = model3(input)
merged_model = merge([m1,m2,m3])
...
answered 2 days ago
Daniel GL
698316
698316
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53206147%2fmerging-keras-models-before-compile-or-fit%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Mwe2hff,nT66m zfh,UK5Xud6K,0VRzcVy NuB3mCFBp5xSbuqTn,6 b8QA3bDuBEb