can’t inherit from superclass [closed]
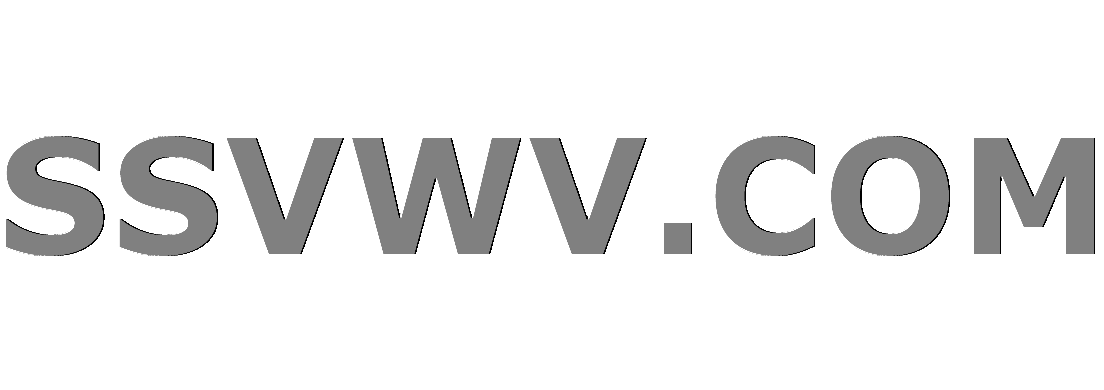
Multi tool use
can’t inherit from superclass [closed]
I have a simple code like below where the class Employee should inherit from the class Person.
class Person:
def __init__(self, firstname, lastname, age):
self.firstname = firstname
self.lastname = lastname
self.age = age
def getname(self):
return self.firstname, self.lastname
def getage(self):
return self.age
class Employee(Person):
def __init__(self, first, last, empid, age):
Person.__init__(self, first, last, age):
self.empid = empid
def getemp(self):
return self.getname() + ", " + self.empid
employee = Employee("Bart", "Simpson", 1006, 16)
print(employee.getemp())
It gives me this error:
File "/tmp/pyadv.py", line 156
Person.__init__(self, first, last, age):
^
SyntaxError: invalid syntax
I checked Python documentation about classes and it didn’t have that superclass initialization inside the __init__()
of the subclass. But I found that in other websites like here, where Dog inherits from Pet.
So, what am I missing?
__init__()
This question appears to be off-topic. The users who voted to close gave this specific reason:
@kindall, ok, I hadn´t noticed that. But how is this off-topic???
– Adriano_epifas
Sep 14 at 14:48
"This question was caused by a problem that can no longer be reproduced or a simple typographical error"
– kindall
Sep 14 at 15:00
2 Answers
2
You're not missing anything. You need to get rid of the :
on that line.
:
:
only comes after the original definition ie: def getname(self):
and is always followed by an indented line that declares the function. When you are calling a function you don't do this.
:
def getname(self):
Change
Person.__init__(self, first, last, age):
to
Person.__init__(self, first, last, age):
super().__init__(first, last, age)
super().__init__(first, last, age)
You have a spurious colon at the end of that line.
– kindall
Aug 26 at 2:46