update only specified element with chrome.storage.local.set
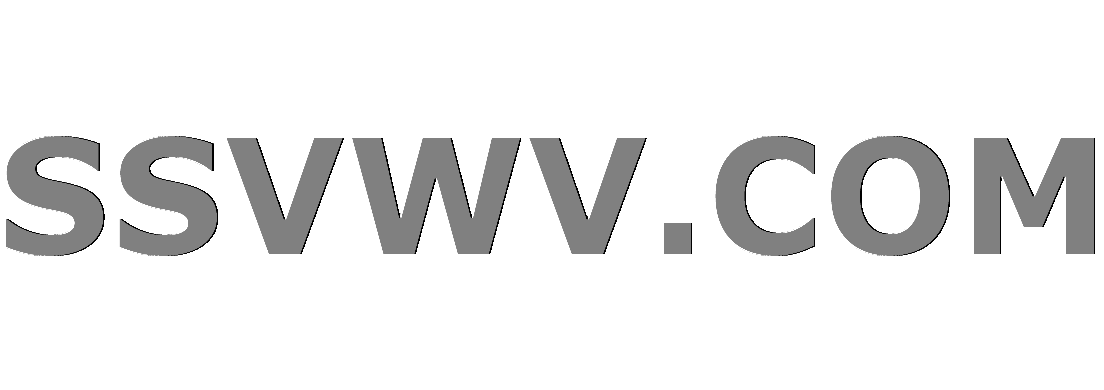
Multi tool use
I have an array in chrome local storage, format:
"flights":
[
"end":"2018-02-10","price":"476","start":"2018-02-01","tabId":1129367822,
"end":"2018-02-11","price":"493","start":"2018-02-01","tabId":1129367825,
"end":"2018-02-12","price":"468","start":"2018-02-01","tabId":1129367828
]
Now I'm updating all data this way:
function updateValue(index, item)
chrome.storage.local.get(['flights'], function (response)
response.flights[index] = item;
chrome.storage.local.set(flights: response.flights);
);
But there is problem with async requests, because I have several request at the time. Some requests get old data and save it again in storage...
I want to update only specified element (for example flights[0] with new data), but it doesn't work...
Something like this, but workable:
chrome.storage.local.set(flights[0]: item);
Is there any way to do this? Or maybe you have some advices to resolve this issue other way.
many thanks for any help
javascript

add a comment |
I have an array in chrome local storage, format:
"flights":
[
"end":"2018-02-10","price":"476","start":"2018-02-01","tabId":1129367822,
"end":"2018-02-11","price":"493","start":"2018-02-01","tabId":1129367825,
"end":"2018-02-12","price":"468","start":"2018-02-01","tabId":1129367828
]
Now I'm updating all data this way:
function updateValue(index, item)
chrome.storage.local.get(['flights'], function (response)
response.flights[index] = item;
chrome.storage.local.set(flights: response.flights);
);
But there is problem with async requests, because I have several request at the time. Some requests get old data and save it again in storage...
I want to update only specified element (for example flights[0] with new data), but it doesn't work...
Something like this, but workable:
chrome.storage.local.set(flights[0]: item);
Is there any way to do this? Or maybe you have some advices to resolve this issue other way.
many thanks for any help
javascript

It's certainly a downside of chrome.storage backend; you only have key-value storage. If you need to modify a top-level value, you need to read it in full, edit it, and write it back in full.
– Xan
Nov 14 '18 at 10:55
add a comment |
I have an array in chrome local storage, format:
"flights":
[
"end":"2018-02-10","price":"476","start":"2018-02-01","tabId":1129367822,
"end":"2018-02-11","price":"493","start":"2018-02-01","tabId":1129367825,
"end":"2018-02-12","price":"468","start":"2018-02-01","tabId":1129367828
]
Now I'm updating all data this way:
function updateValue(index, item)
chrome.storage.local.get(['flights'], function (response)
response.flights[index] = item;
chrome.storage.local.set(flights: response.flights);
);
But there is problem with async requests, because I have several request at the time. Some requests get old data and save it again in storage...
I want to update only specified element (for example flights[0] with new data), but it doesn't work...
Something like this, but workable:
chrome.storage.local.set(flights[0]: item);
Is there any way to do this? Or maybe you have some advices to resolve this issue other way.
many thanks for any help
javascript

I have an array in chrome local storage, format:
"flights":
[
"end":"2018-02-10","price":"476","start":"2018-02-01","tabId":1129367822,
"end":"2018-02-11","price":"493","start":"2018-02-01","tabId":1129367825,
"end":"2018-02-12","price":"468","start":"2018-02-01","tabId":1129367828
]
Now I'm updating all data this way:
function updateValue(index, item)
chrome.storage.local.get(['flights'], function (response)
response.flights[index] = item;
chrome.storage.local.set(flights: response.flights);
);
But there is problem with async requests, because I have several request at the time. Some requests get old data and save it again in storage...
I want to update only specified element (for example flights[0] with new data), but it doesn't work...
Something like this, but workable:
chrome.storage.local.set(flights[0]: item);
Is there any way to do this? Or maybe you have some advices to resolve this issue other way.
many thanks for any help
javascript

javascript

asked Nov 12 '18 at 18:16


MrSmileMrSmile
1,0281618
1,0281618
It's certainly a downside of chrome.storage backend; you only have key-value storage. If you need to modify a top-level value, you need to read it in full, edit it, and write it back in full.
– Xan
Nov 14 '18 at 10:55
add a comment |
It's certainly a downside of chrome.storage backend; you only have key-value storage. If you need to modify a top-level value, you need to read it in full, edit it, and write it back in full.
– Xan
Nov 14 '18 at 10:55
It's certainly a downside of chrome.storage backend; you only have key-value storage. If you need to modify a top-level value, you need to read it in full, edit it, and write it back in full.
– Xan
Nov 14 '18 at 10:55
It's certainly a downside of chrome.storage backend; you only have key-value storage. If you need to modify a top-level value, you need to read it in full, edit it, and write it back in full.
– Xan
Nov 14 '18 at 10:55
add a comment |
2 Answers
2
active
oldest
votes
You can save each flight into a separate key and get all flights by traversing all storage:
cosnt flightPrefix = 'flight_';
function updateValue(index, item)
chrome.storage.local.set(flightPrefix + index: item);
function getFlights()
// Pass in null to get the entire contents of storage.
chrome.storage.sync.get(null, function(items)
let flights = Object.keys(items).filter(key => key.beginsWith(flightPrefix));
console.log(flights);
);
add a comment |
Based on terales' answer (that code has some errors).
I make it this way:
function parseFlight(result)
let flightsArray = ;
Object.keys(result).forEach(function (key)
if (key.includes('flight'))
let index = key.replace('flight_', '');
flightsArray[index] = result[key];
);
return flightsArray;
function updateValue(index, item)
let flightPrefix = 'flight_';
let obj = ;
obj[flightPrefix + index] = item;
chrome.storage.local.set(obj);
chrome.storage.local.get(null, function (result)
let flights = parseFlight(result);
);
Thanks for help!
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53267902%2fupdate-only-specified-element-with-chrome-storage-local-set%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can save each flight into a separate key and get all flights by traversing all storage:
cosnt flightPrefix = 'flight_';
function updateValue(index, item)
chrome.storage.local.set(flightPrefix + index: item);
function getFlights()
// Pass in null to get the entire contents of storage.
chrome.storage.sync.get(null, function(items)
let flights = Object.keys(items).filter(key => key.beginsWith(flightPrefix));
console.log(flights);
);
add a comment |
You can save each flight into a separate key and get all flights by traversing all storage:
cosnt flightPrefix = 'flight_';
function updateValue(index, item)
chrome.storage.local.set(flightPrefix + index: item);
function getFlights()
// Pass in null to get the entire contents of storage.
chrome.storage.sync.get(null, function(items)
let flights = Object.keys(items).filter(key => key.beginsWith(flightPrefix));
console.log(flights);
);
add a comment |
You can save each flight into a separate key and get all flights by traversing all storage:
cosnt flightPrefix = 'flight_';
function updateValue(index, item)
chrome.storage.local.set(flightPrefix + index: item);
function getFlights()
// Pass in null to get the entire contents of storage.
chrome.storage.sync.get(null, function(items)
let flights = Object.keys(items).filter(key => key.beginsWith(flightPrefix));
console.log(flights);
);
You can save each flight into a separate key and get all flights by traversing all storage:
cosnt flightPrefix = 'flight_';
function updateValue(index, item)
chrome.storage.local.set(flightPrefix + index: item);
function getFlights()
// Pass in null to get the entire contents of storage.
chrome.storage.sync.get(null, function(items)
let flights = Object.keys(items).filter(key => key.beginsWith(flightPrefix));
console.log(flights);
);
answered Nov 12 '18 at 18:28


teralesterales
1,7321526
1,7321526
add a comment |
add a comment |
Based on terales' answer (that code has some errors).
I make it this way:
function parseFlight(result)
let flightsArray = ;
Object.keys(result).forEach(function (key)
if (key.includes('flight'))
let index = key.replace('flight_', '');
flightsArray[index] = result[key];
);
return flightsArray;
function updateValue(index, item)
let flightPrefix = 'flight_';
let obj = ;
obj[flightPrefix + index] = item;
chrome.storage.local.set(obj);
chrome.storage.local.get(null, function (result)
let flights = parseFlight(result);
);
Thanks for help!
add a comment |
Based on terales' answer (that code has some errors).
I make it this way:
function parseFlight(result)
let flightsArray = ;
Object.keys(result).forEach(function (key)
if (key.includes('flight'))
let index = key.replace('flight_', '');
flightsArray[index] = result[key];
);
return flightsArray;
function updateValue(index, item)
let flightPrefix = 'flight_';
let obj = ;
obj[flightPrefix + index] = item;
chrome.storage.local.set(obj);
chrome.storage.local.get(null, function (result)
let flights = parseFlight(result);
);
Thanks for help!
add a comment |
Based on terales' answer (that code has some errors).
I make it this way:
function parseFlight(result)
let flightsArray = ;
Object.keys(result).forEach(function (key)
if (key.includes('flight'))
let index = key.replace('flight_', '');
flightsArray[index] = result[key];
);
return flightsArray;
function updateValue(index, item)
let flightPrefix = 'flight_';
let obj = ;
obj[flightPrefix + index] = item;
chrome.storage.local.set(obj);
chrome.storage.local.get(null, function (result)
let flights = parseFlight(result);
);
Thanks for help!
Based on terales' answer (that code has some errors).
I make it this way:
function parseFlight(result)
let flightsArray = ;
Object.keys(result).forEach(function (key)
if (key.includes('flight'))
let index = key.replace('flight_', '');
flightsArray[index] = result[key];
);
return flightsArray;
function updateValue(index, item)
let flightPrefix = 'flight_';
let obj = ;
obj[flightPrefix + index] = item;
chrome.storage.local.set(obj);
chrome.storage.local.get(null, function (result)
let flights = parseFlight(result);
);
Thanks for help!
answered Nov 14 '18 at 7:51


MrSmileMrSmile
1,0281618
1,0281618
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53267902%2fupdate-only-specified-element-with-chrome-storage-local-set%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
2xGBJ,2 cZSsdmYUHlANWcxa2kk,Uqc,QA1kJJSvFq 6XMB G5bVvBXsFz6mGczcB,xyt,av6HQkOeWg sbA,xRkh
It's certainly a downside of chrome.storage backend; you only have key-value storage. If you need to modify a top-level value, you need to read it in full, edit it, and write it back in full.
– Xan
Nov 14 '18 at 10:55