Should HTTP codes be used to represent Business Failure?
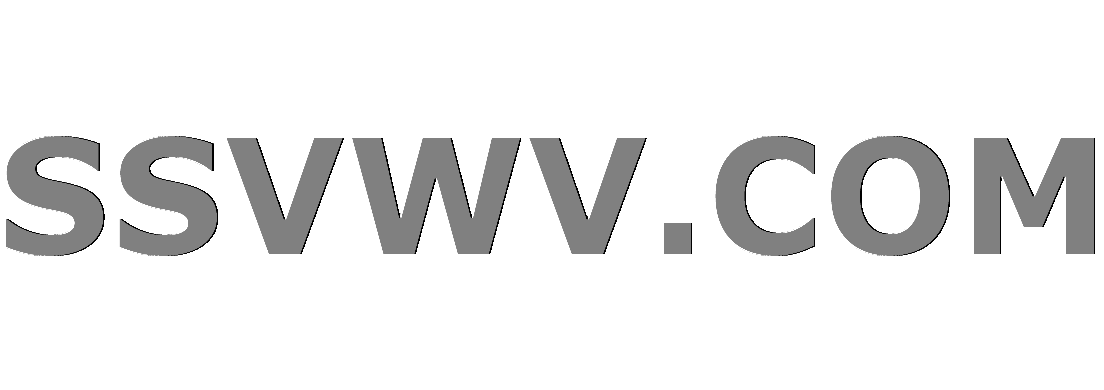
Multi tool use
My team had a discussion early this week about if HTTP Codes should represent Business Failures.
Imagine a scenario where we have a Customer
REST API. Within that API, we have lots of operations, like:
POST -mydomain.com/customers
(receive a JSON body and create a new Customer)
GET -mydomain.com/customers/id
(search for a specific Customer)
PATCH -mydomain.com/customers/id
(receive a JSON body and patch a specific Customer)
DELETE -mydomain.com;customers/id
(deletes a specific Customer)
Now, imagine a situation where I'm looking for a Customer
which has the id = 5
.
There's no Customer
with the id = 5
. What should I do in terms of HTTP status code?
Customer not found is a Business Failure. Should I return a 404 - NOT FOUND? Should I return a 200 - OK (with a JSON body describing that the Customer with ID 5 do not exist)?
We had a discussion exactly on that behavior.
Controller.java (example)
@GetMapping("/customers/id")
public ResponseEntity<?> handleRequestOfRetrieveCustomerById(@PathVariable("id") Integer id) throws CustomerNotFoundException
try
ResponseEntity.ok(customerService.findCustomerById(id));
catch(CustomerNotFoundException e)
// log at Controller level and rethrow
throw e;
Handler.java (example)
@ExceptionHandler(BusinessResourceNotFoundException.class)
@ResponseBody
protected ResponseEntity<Fault> handleExceptionOfBusinessResourceNotFound(BusinessResourceNotFoundException exception)
return new ResponseEntity<Fault>(exception.getFault(), HttpStatus.NOT_FOUND);
In this example, a 404 - NOT FOUND is returned with a body giving more details to the client.
From reading the HTTP/1.1 Specification:
404 Not Found
The server has not found anything matching the Request-URI. No
indication is given of whether the condition is temporary or
permanent. The 410 (Gone) status code SHOULD be used if the server
knows, through some internally configurable mechanism, that an old
resource is permanently unavailable and has no forwarding address.
This status code is commonly used when the server does not wish to
reveal exactly why the request has been refused, or when no other
response is applicable.
If "The server has not found anything matching the Request-URI...", I understand that returning a 404 - NOT FOUND would be the correct approach., since the /id composes my URI (mydomain.com/customers/id)
Am I right?
Which one is the better / right (if there's a wrong way) approach?
java spring rest api http
add a comment |
My team had a discussion early this week about if HTTP Codes should represent Business Failures.
Imagine a scenario where we have a Customer
REST API. Within that API, we have lots of operations, like:
POST -mydomain.com/customers
(receive a JSON body and create a new Customer)
GET -mydomain.com/customers/id
(search for a specific Customer)
PATCH -mydomain.com/customers/id
(receive a JSON body and patch a specific Customer)
DELETE -mydomain.com;customers/id
(deletes a specific Customer)
Now, imagine a situation where I'm looking for a Customer
which has the id = 5
.
There's no Customer
with the id = 5
. What should I do in terms of HTTP status code?
Customer not found is a Business Failure. Should I return a 404 - NOT FOUND? Should I return a 200 - OK (with a JSON body describing that the Customer with ID 5 do not exist)?
We had a discussion exactly on that behavior.
Controller.java (example)
@GetMapping("/customers/id")
public ResponseEntity<?> handleRequestOfRetrieveCustomerById(@PathVariable("id") Integer id) throws CustomerNotFoundException
try
ResponseEntity.ok(customerService.findCustomerById(id));
catch(CustomerNotFoundException e)
// log at Controller level and rethrow
throw e;
Handler.java (example)
@ExceptionHandler(BusinessResourceNotFoundException.class)
@ResponseBody
protected ResponseEntity<Fault> handleExceptionOfBusinessResourceNotFound(BusinessResourceNotFoundException exception)
return new ResponseEntity<Fault>(exception.getFault(), HttpStatus.NOT_FOUND);
In this example, a 404 - NOT FOUND is returned with a body giving more details to the client.
From reading the HTTP/1.1 Specification:
404 Not Found
The server has not found anything matching the Request-URI. No
indication is given of whether the condition is temporary or
permanent. The 410 (Gone) status code SHOULD be used if the server
knows, through some internally configurable mechanism, that an old
resource is permanently unavailable and has no forwarding address.
This status code is commonly used when the server does not wish to
reveal exactly why the request has been refused, or when no other
response is applicable.
If "The server has not found anything matching the Request-URI...", I understand that returning a 404 - NOT FOUND would be the correct approach., since the /id composes my URI (mydomain.com/customers/id)
Am I right?
Which one is the better / right (if there's a wrong way) approach?
java spring rest api http
@pzaenger it was one of the approaches we discussed
– Matheus Cirillo
Nov 7 '18 at 14:18
While mapping HTTP operations to simple CRUD operations might sound like a good idea, HTTP operations in genearal are to general to support such a mapping blindly. I.e. DELETE only gurantees that the connection between the URL and the resource is removed but leaves the client clueless whether the server also removes the data belonging to the resource as well. A client in general is usually not interested in such internal decisions anyway as long as the resource state isn't retrievable any longer.
– Roman Vottner
Nov 7 '18 at 15:04
Further, the spec leaves room for interpretation. DELETE i.e. only states that certain response codes should be returned if the operation actually removed something but not what should happen if i.e. the resource was not available at all. Here it is more or less individual preference. All a client wants is that a certain URI shouldn't return a resource's state after the invocation. A 404 tells the client the the resource didn't exist before while a 200 response informs a client that no representation for the requeted URI is available any further. ...
– Roman Vottner
Nov 7 '18 at 15:20
... The effect, however, are pretty similar that invoking the URL doesn't return any resource's state further. It is therefore more or less an implementation detail not that important to clients
– Roman Vottner
Nov 7 '18 at 15:20
add a comment |
My team had a discussion early this week about if HTTP Codes should represent Business Failures.
Imagine a scenario where we have a Customer
REST API. Within that API, we have lots of operations, like:
POST -mydomain.com/customers
(receive a JSON body and create a new Customer)
GET -mydomain.com/customers/id
(search for a specific Customer)
PATCH -mydomain.com/customers/id
(receive a JSON body and patch a specific Customer)
DELETE -mydomain.com;customers/id
(deletes a specific Customer)
Now, imagine a situation where I'm looking for a Customer
which has the id = 5
.
There's no Customer
with the id = 5
. What should I do in terms of HTTP status code?
Customer not found is a Business Failure. Should I return a 404 - NOT FOUND? Should I return a 200 - OK (with a JSON body describing that the Customer with ID 5 do not exist)?
We had a discussion exactly on that behavior.
Controller.java (example)
@GetMapping("/customers/id")
public ResponseEntity<?> handleRequestOfRetrieveCustomerById(@PathVariable("id") Integer id) throws CustomerNotFoundException
try
ResponseEntity.ok(customerService.findCustomerById(id));
catch(CustomerNotFoundException e)
// log at Controller level and rethrow
throw e;
Handler.java (example)
@ExceptionHandler(BusinessResourceNotFoundException.class)
@ResponseBody
protected ResponseEntity<Fault> handleExceptionOfBusinessResourceNotFound(BusinessResourceNotFoundException exception)
return new ResponseEntity<Fault>(exception.getFault(), HttpStatus.NOT_FOUND);
In this example, a 404 - NOT FOUND is returned with a body giving more details to the client.
From reading the HTTP/1.1 Specification:
404 Not Found
The server has not found anything matching the Request-URI. No
indication is given of whether the condition is temporary or
permanent. The 410 (Gone) status code SHOULD be used if the server
knows, through some internally configurable mechanism, that an old
resource is permanently unavailable and has no forwarding address.
This status code is commonly used when the server does not wish to
reveal exactly why the request has been refused, or when no other
response is applicable.
If "The server has not found anything matching the Request-URI...", I understand that returning a 404 - NOT FOUND would be the correct approach., since the /id composes my URI (mydomain.com/customers/id)
Am I right?
Which one is the better / right (if there's a wrong way) approach?
java spring rest api http
My team had a discussion early this week about if HTTP Codes should represent Business Failures.
Imagine a scenario where we have a Customer
REST API. Within that API, we have lots of operations, like:
POST -mydomain.com/customers
(receive a JSON body and create a new Customer)
GET -mydomain.com/customers/id
(search for a specific Customer)
PATCH -mydomain.com/customers/id
(receive a JSON body and patch a specific Customer)
DELETE -mydomain.com;customers/id
(deletes a specific Customer)
Now, imagine a situation where I'm looking for a Customer
which has the id = 5
.
There's no Customer
with the id = 5
. What should I do in terms of HTTP status code?
Customer not found is a Business Failure. Should I return a 404 - NOT FOUND? Should I return a 200 - OK (with a JSON body describing that the Customer with ID 5 do not exist)?
We had a discussion exactly on that behavior.
Controller.java (example)
@GetMapping("/customers/id")
public ResponseEntity<?> handleRequestOfRetrieveCustomerById(@PathVariable("id") Integer id) throws CustomerNotFoundException
try
ResponseEntity.ok(customerService.findCustomerById(id));
catch(CustomerNotFoundException e)
// log at Controller level and rethrow
throw e;
Handler.java (example)
@ExceptionHandler(BusinessResourceNotFoundException.class)
@ResponseBody
protected ResponseEntity<Fault> handleExceptionOfBusinessResourceNotFound(BusinessResourceNotFoundException exception)
return new ResponseEntity<Fault>(exception.getFault(), HttpStatus.NOT_FOUND);
In this example, a 404 - NOT FOUND is returned with a body giving more details to the client.
From reading the HTTP/1.1 Specification:
404 Not Found
The server has not found anything matching the Request-URI. No
indication is given of whether the condition is temporary or
permanent. The 410 (Gone) status code SHOULD be used if the server
knows, through some internally configurable mechanism, that an old
resource is permanently unavailable and has no forwarding address.
This status code is commonly used when the server does not wish to
reveal exactly why the request has been refused, or when no other
response is applicable.
If "The server has not found anything matching the Request-URI...", I understand that returning a 404 - NOT FOUND would be the correct approach., since the /id composes my URI (mydomain.com/customers/id)
Am I right?
Which one is the better / right (if there's a wrong way) approach?
java spring rest api http
java spring rest api http
edited Nov 8 '18 at 10:39
Matheus Cirillo
asked Nov 7 '18 at 14:15


Matheus CirilloMatheus Cirillo
102110
102110
@pzaenger it was one of the approaches we discussed
– Matheus Cirillo
Nov 7 '18 at 14:18
While mapping HTTP operations to simple CRUD operations might sound like a good idea, HTTP operations in genearal are to general to support such a mapping blindly. I.e. DELETE only gurantees that the connection between the URL and the resource is removed but leaves the client clueless whether the server also removes the data belonging to the resource as well. A client in general is usually not interested in such internal decisions anyway as long as the resource state isn't retrievable any longer.
– Roman Vottner
Nov 7 '18 at 15:04
Further, the spec leaves room for interpretation. DELETE i.e. only states that certain response codes should be returned if the operation actually removed something but not what should happen if i.e. the resource was not available at all. Here it is more or less individual preference. All a client wants is that a certain URI shouldn't return a resource's state after the invocation. A 404 tells the client the the resource didn't exist before while a 200 response informs a client that no representation for the requeted URI is available any further. ...
– Roman Vottner
Nov 7 '18 at 15:20
... The effect, however, are pretty similar that invoking the URL doesn't return any resource's state further. It is therefore more or less an implementation detail not that important to clients
– Roman Vottner
Nov 7 '18 at 15:20
add a comment |
@pzaenger it was one of the approaches we discussed
– Matheus Cirillo
Nov 7 '18 at 14:18
While mapping HTTP operations to simple CRUD operations might sound like a good idea, HTTP operations in genearal are to general to support such a mapping blindly. I.e. DELETE only gurantees that the connection between the URL and the resource is removed but leaves the client clueless whether the server also removes the data belonging to the resource as well. A client in general is usually not interested in such internal decisions anyway as long as the resource state isn't retrievable any longer.
– Roman Vottner
Nov 7 '18 at 15:04
Further, the spec leaves room for interpretation. DELETE i.e. only states that certain response codes should be returned if the operation actually removed something but not what should happen if i.e. the resource was not available at all. Here it is more or less individual preference. All a client wants is that a certain URI shouldn't return a resource's state after the invocation. A 404 tells the client the the resource didn't exist before while a 200 response informs a client that no representation for the requeted URI is available any further. ...
– Roman Vottner
Nov 7 '18 at 15:20
... The effect, however, are pretty similar that invoking the URL doesn't return any resource's state further. It is therefore more or less an implementation detail not that important to clients
– Roman Vottner
Nov 7 '18 at 15:20
@pzaenger it was one of the approaches we discussed
– Matheus Cirillo
Nov 7 '18 at 14:18
@pzaenger it was one of the approaches we discussed
– Matheus Cirillo
Nov 7 '18 at 14:18
While mapping HTTP operations to simple CRUD operations might sound like a good idea, HTTP operations in genearal are to general to support such a mapping blindly. I.e. DELETE only gurantees that the connection between the URL and the resource is removed but leaves the client clueless whether the server also removes the data belonging to the resource as well. A client in general is usually not interested in such internal decisions anyway as long as the resource state isn't retrievable any longer.
– Roman Vottner
Nov 7 '18 at 15:04
While mapping HTTP operations to simple CRUD operations might sound like a good idea, HTTP operations in genearal are to general to support such a mapping blindly. I.e. DELETE only gurantees that the connection between the URL and the resource is removed but leaves the client clueless whether the server also removes the data belonging to the resource as well. A client in general is usually not interested in such internal decisions anyway as long as the resource state isn't retrievable any longer.
– Roman Vottner
Nov 7 '18 at 15:04
Further, the spec leaves room for interpretation. DELETE i.e. only states that certain response codes should be returned if the operation actually removed something but not what should happen if i.e. the resource was not available at all. Here it is more or less individual preference. All a client wants is that a certain URI shouldn't return a resource's state after the invocation. A 404 tells the client the the resource didn't exist before while a 200 response informs a client that no representation for the requeted URI is available any further. ...
– Roman Vottner
Nov 7 '18 at 15:20
Further, the spec leaves room for interpretation. DELETE i.e. only states that certain response codes should be returned if the operation actually removed something but not what should happen if i.e. the resource was not available at all. Here it is more or less individual preference. All a client wants is that a certain URI shouldn't return a resource's state after the invocation. A 404 tells the client the the resource didn't exist before while a 200 response informs a client that no representation for the requeted URI is available any further. ...
– Roman Vottner
Nov 7 '18 at 15:20
... The effect, however, are pretty similar that invoking the URL doesn't return any resource's state further. It is therefore more or less an implementation detail not that important to clients
– Roman Vottner
Nov 7 '18 at 15:20
... The effect, however, are pretty similar that invoking the URL doesn't return any resource's state further. It is therefore more or less an implementation detail not that important to clients
– Roman Vottner
Nov 7 '18 at 15:20
add a comment |
6 Answers
6
active
oldest
votes
Status codes are meant to describe the result of the server's attempt to understand and satisfy the client's corresponding request.
Ultimately, if a client requests a representation of a resource that doesn't exist, the server should return 404
to indicate that. It's essentially a client error and should be reported as such.
Returning 200
would be misleading and would cause confusion to API clients.
Sometimes the HTTP status codes are not sufficient to convey enough information about an error to be helpful.
The RFC 7807 was created to define simple JSON and XML document formats to inform the client about a problem in a HTTP API. It's a great start point for reporting errors in your API. It also defines the application/problem+json
and application/problem+xml
media types.
add a comment |
Technically and from the http point of view, 404 should also be returned for any misspelling of the entity name (cutsomer instead of customer).
So even if you decide that "customer not found" will result in http 404, you cannot conclude that http 404 will imply "entity occurrence not found".
Oh, sure. But I can conclude that something was wrong within the Requested-URI
– Matheus Cirillo
Nov 8 '18 at 11:07
1
Well yes but "something wrong with the requested URI" is not something that necessarily caries business meaning and your question was quite particularly about "should represent business failures". You do want to distinguish "business-level" failures from the other ones.
– Erwin Smout
Nov 8 '18 at 13:10
add a comment |
HTTP codes exist for a reason. Whoever consumes your API should be able to handle the response straight away, without having to result on the body contents.
In your case, 404(Not Found) looks quite suitable.
Alternatively, if you always return a 200, doesn't that beat the purpose of a response code altogether? If you are getting a response, you already know that your request got through to some extent.
TLDR;
Use 404 :)
add a comment |
I have recently worked on a Rest API
with Spring Boot
and the best practices found on the internet said this :
- Parameters null or value not set :
400 / Bad request
- Returned value not found (record or list empty) :
404 / Not found
- Exception from server (database error, network error etc.) :
500 / Internal server error
Those links will help you : best practice error handling, ControllerAdvice, error message
add a comment |
A REST API is part of the integration domain, not the business domain. It's a skin that your domain model wears to disguise itself as a web site aka an HTTP compliant key value store.
Here, 404 is an appropriate choice because it mimics the response that would be returned by a key value store if you tried to get a key that wasn't currently stored.
add a comment |
I would follow the meaning of HTTP status codes.
Wiki says (https://en.wikipedia.org/wiki/List_of_HTTP_status_codes):
This class of status code is intended for situations in which the error seems to have been caused by the client
So to be clear: mydomain.com/customers/id is a valid URL an the server understands the request. The fact that customer with id=5 doesn't exists has nothing to do with a "false URL" or "not understandable request".
In my opinion this should return some 2xx status code with further information inside json (definitions made by your REST API)
1
Wikipedia is not a reference for HTTP status codes. Refer to the IANA status code registry for the atual document that defines each status code.404
, for instance, is defined in the RFC 7231, the document that defines the semantics of the HTTP/1.1.
– Cassio Mazzochi Molin
Nov 7 '18 at 14:49
1
@CassioMazzochiMolin Thanks for the link. It seems that the preferred way will be your answer. Learned from that :-)
– Mankeldor
Nov 7 '18 at 15:04
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53191230%2fshould-http-codes-be-used-to-represent-business-failure%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
Status codes are meant to describe the result of the server's attempt to understand and satisfy the client's corresponding request.
Ultimately, if a client requests a representation of a resource that doesn't exist, the server should return 404
to indicate that. It's essentially a client error and should be reported as such.
Returning 200
would be misleading and would cause confusion to API clients.
Sometimes the HTTP status codes are not sufficient to convey enough information about an error to be helpful.
The RFC 7807 was created to define simple JSON and XML document formats to inform the client about a problem in a HTTP API. It's a great start point for reporting errors in your API. It also defines the application/problem+json
and application/problem+xml
media types.
add a comment |
Status codes are meant to describe the result of the server's attempt to understand and satisfy the client's corresponding request.
Ultimately, if a client requests a representation of a resource that doesn't exist, the server should return 404
to indicate that. It's essentially a client error and should be reported as such.
Returning 200
would be misleading and would cause confusion to API clients.
Sometimes the HTTP status codes are not sufficient to convey enough information about an error to be helpful.
The RFC 7807 was created to define simple JSON and XML document formats to inform the client about a problem in a HTTP API. It's a great start point for reporting errors in your API. It also defines the application/problem+json
and application/problem+xml
media types.
add a comment |
Status codes are meant to describe the result of the server's attempt to understand and satisfy the client's corresponding request.
Ultimately, if a client requests a representation of a resource that doesn't exist, the server should return 404
to indicate that. It's essentially a client error and should be reported as such.
Returning 200
would be misleading and would cause confusion to API clients.
Sometimes the HTTP status codes are not sufficient to convey enough information about an error to be helpful.
The RFC 7807 was created to define simple JSON and XML document formats to inform the client about a problem in a HTTP API. It's a great start point for reporting errors in your API. It also defines the application/problem+json
and application/problem+xml
media types.
Status codes are meant to describe the result of the server's attempt to understand and satisfy the client's corresponding request.
Ultimately, if a client requests a representation of a resource that doesn't exist, the server should return 404
to indicate that. It's essentially a client error and should be reported as such.
Returning 200
would be misleading and would cause confusion to API clients.
Sometimes the HTTP status codes are not sufficient to convey enough information about an error to be helpful.
The RFC 7807 was created to define simple JSON and XML document formats to inform the client about a problem in a HTTP API. It's a great start point for reporting errors in your API. It also defines the application/problem+json
and application/problem+xml
media types.
edited Nov 12 '18 at 8:18
answered Nov 7 '18 at 14:27


Cassio Mazzochi MolinCassio Mazzochi Molin
58.8k17113187
58.8k17113187
add a comment |
add a comment |
Technically and from the http point of view, 404 should also be returned for any misspelling of the entity name (cutsomer instead of customer).
So even if you decide that "customer not found" will result in http 404, you cannot conclude that http 404 will imply "entity occurrence not found".
Oh, sure. But I can conclude that something was wrong within the Requested-URI
– Matheus Cirillo
Nov 8 '18 at 11:07
1
Well yes but "something wrong with the requested URI" is not something that necessarily caries business meaning and your question was quite particularly about "should represent business failures". You do want to distinguish "business-level" failures from the other ones.
– Erwin Smout
Nov 8 '18 at 13:10
add a comment |
Technically and from the http point of view, 404 should also be returned for any misspelling of the entity name (cutsomer instead of customer).
So even if you decide that "customer not found" will result in http 404, you cannot conclude that http 404 will imply "entity occurrence not found".
Oh, sure. But I can conclude that something was wrong within the Requested-URI
– Matheus Cirillo
Nov 8 '18 at 11:07
1
Well yes but "something wrong with the requested URI" is not something that necessarily caries business meaning and your question was quite particularly about "should represent business failures". You do want to distinguish "business-level" failures from the other ones.
– Erwin Smout
Nov 8 '18 at 13:10
add a comment |
Technically and from the http point of view, 404 should also be returned for any misspelling of the entity name (cutsomer instead of customer).
So even if you decide that "customer not found" will result in http 404, you cannot conclude that http 404 will imply "entity occurrence not found".
Technically and from the http point of view, 404 should also be returned for any misspelling of the entity name (cutsomer instead of customer).
So even if you decide that "customer not found" will result in http 404, you cannot conclude that http 404 will imply "entity occurrence not found".
answered Nov 8 '18 at 11:00
Erwin SmoutErwin Smout
15.1k42148
15.1k42148
Oh, sure. But I can conclude that something was wrong within the Requested-URI
– Matheus Cirillo
Nov 8 '18 at 11:07
1
Well yes but "something wrong with the requested URI" is not something that necessarily caries business meaning and your question was quite particularly about "should represent business failures". You do want to distinguish "business-level" failures from the other ones.
– Erwin Smout
Nov 8 '18 at 13:10
add a comment |
Oh, sure. But I can conclude that something was wrong within the Requested-URI
– Matheus Cirillo
Nov 8 '18 at 11:07
1
Well yes but "something wrong with the requested URI" is not something that necessarily caries business meaning and your question was quite particularly about "should represent business failures". You do want to distinguish "business-level" failures from the other ones.
– Erwin Smout
Nov 8 '18 at 13:10
Oh, sure. But I can conclude that something was wrong within the Requested-URI
– Matheus Cirillo
Nov 8 '18 at 11:07
Oh, sure. But I can conclude that something was wrong within the Requested-URI
– Matheus Cirillo
Nov 8 '18 at 11:07
1
1
Well yes but "something wrong with the requested URI" is not something that necessarily caries business meaning and your question was quite particularly about "should represent business failures". You do want to distinguish "business-level" failures from the other ones.
– Erwin Smout
Nov 8 '18 at 13:10
Well yes but "something wrong with the requested URI" is not something that necessarily caries business meaning and your question was quite particularly about "should represent business failures". You do want to distinguish "business-level" failures from the other ones.
– Erwin Smout
Nov 8 '18 at 13:10
add a comment |
HTTP codes exist for a reason. Whoever consumes your API should be able to handle the response straight away, without having to result on the body contents.
In your case, 404(Not Found) looks quite suitable.
Alternatively, if you always return a 200, doesn't that beat the purpose of a response code altogether? If you are getting a response, you already know that your request got through to some extent.
TLDR;
Use 404 :)
add a comment |
HTTP codes exist for a reason. Whoever consumes your API should be able to handle the response straight away, without having to result on the body contents.
In your case, 404(Not Found) looks quite suitable.
Alternatively, if you always return a 200, doesn't that beat the purpose of a response code altogether? If you are getting a response, you already know that your request got through to some extent.
TLDR;
Use 404 :)
add a comment |
HTTP codes exist for a reason. Whoever consumes your API should be able to handle the response straight away, without having to result on the body contents.
In your case, 404(Not Found) looks quite suitable.
Alternatively, if you always return a 200, doesn't that beat the purpose of a response code altogether? If you are getting a response, you already know that your request got through to some extent.
TLDR;
Use 404 :)
HTTP codes exist for a reason. Whoever consumes your API should be able to handle the response straight away, without having to result on the body contents.
In your case, 404(Not Found) looks quite suitable.
Alternatively, if you always return a 200, doesn't that beat the purpose of a response code altogether? If you are getting a response, you already know that your request got through to some extent.
TLDR;
Use 404 :)
answered Nov 7 '18 at 14:30
Sofo GialSofo Gial
470515
470515
add a comment |
add a comment |
I have recently worked on a Rest API
with Spring Boot
and the best practices found on the internet said this :
- Parameters null or value not set :
400 / Bad request
- Returned value not found (record or list empty) :
404 / Not found
- Exception from server (database error, network error etc.) :
500 / Internal server error
Those links will help you : best practice error handling, ControllerAdvice, error message
add a comment |
I have recently worked on a Rest API
with Spring Boot
and the best practices found on the internet said this :
- Parameters null or value not set :
400 / Bad request
- Returned value not found (record or list empty) :
404 / Not found
- Exception from server (database error, network error etc.) :
500 / Internal server error
Those links will help you : best practice error handling, ControllerAdvice, error message
add a comment |
I have recently worked on a Rest API
with Spring Boot
and the best practices found on the internet said this :
- Parameters null or value not set :
400 / Bad request
- Returned value not found (record or list empty) :
404 / Not found
- Exception from server (database error, network error etc.) :
500 / Internal server error
Those links will help you : best practice error handling, ControllerAdvice, error message
I have recently worked on a Rest API
with Spring Boot
and the best practices found on the internet said this :
- Parameters null or value not set :
400 / Bad request
- Returned value not found (record or list empty) :
404 / Not found
- Exception from server (database error, network error etc.) :
500 / Internal server error
Those links will help you : best practice error handling, ControllerAdvice, error message
answered Nov 7 '18 at 17:05
Harry CoderHarry Coder
2021417
2021417
add a comment |
add a comment |
A REST API is part of the integration domain, not the business domain. It's a skin that your domain model wears to disguise itself as a web site aka an HTTP compliant key value store.
Here, 404 is an appropriate choice because it mimics the response that would be returned by a key value store if you tried to get a key that wasn't currently stored.
add a comment |
A REST API is part of the integration domain, not the business domain. It's a skin that your domain model wears to disguise itself as a web site aka an HTTP compliant key value store.
Here, 404 is an appropriate choice because it mimics the response that would be returned by a key value store if you tried to get a key that wasn't currently stored.
add a comment |
A REST API is part of the integration domain, not the business domain. It's a skin that your domain model wears to disguise itself as a web site aka an HTTP compliant key value store.
Here, 404 is an appropriate choice because it mimics the response that would be returned by a key value store if you tried to get a key that wasn't currently stored.
A REST API is part of the integration domain, not the business domain. It's a skin that your domain model wears to disguise itself as a web site aka an HTTP compliant key value store.
Here, 404 is an appropriate choice because it mimics the response that would be returned by a key value store if you tried to get a key that wasn't currently stored.
answered Nov 8 '18 at 10:21
VoiceOfUnreasonVoiceOfUnreason
20.8k22047
20.8k22047
add a comment |
add a comment |
I would follow the meaning of HTTP status codes.
Wiki says (https://en.wikipedia.org/wiki/List_of_HTTP_status_codes):
This class of status code is intended for situations in which the error seems to have been caused by the client
So to be clear: mydomain.com/customers/id is a valid URL an the server understands the request. The fact that customer with id=5 doesn't exists has nothing to do with a "false URL" or "not understandable request".
In my opinion this should return some 2xx status code with further information inside json (definitions made by your REST API)
1
Wikipedia is not a reference for HTTP status codes. Refer to the IANA status code registry for the atual document that defines each status code.404
, for instance, is defined in the RFC 7231, the document that defines the semantics of the HTTP/1.1.
– Cassio Mazzochi Molin
Nov 7 '18 at 14:49
1
@CassioMazzochiMolin Thanks for the link. It seems that the preferred way will be your answer. Learned from that :-)
– Mankeldor
Nov 7 '18 at 15:04
add a comment |
I would follow the meaning of HTTP status codes.
Wiki says (https://en.wikipedia.org/wiki/List_of_HTTP_status_codes):
This class of status code is intended for situations in which the error seems to have been caused by the client
So to be clear: mydomain.com/customers/id is a valid URL an the server understands the request. The fact that customer with id=5 doesn't exists has nothing to do with a "false URL" or "not understandable request".
In my opinion this should return some 2xx status code with further information inside json (definitions made by your REST API)
1
Wikipedia is not a reference for HTTP status codes. Refer to the IANA status code registry for the atual document that defines each status code.404
, for instance, is defined in the RFC 7231, the document that defines the semantics of the HTTP/1.1.
– Cassio Mazzochi Molin
Nov 7 '18 at 14:49
1
@CassioMazzochiMolin Thanks for the link. It seems that the preferred way will be your answer. Learned from that :-)
– Mankeldor
Nov 7 '18 at 15:04
add a comment |
I would follow the meaning of HTTP status codes.
Wiki says (https://en.wikipedia.org/wiki/List_of_HTTP_status_codes):
This class of status code is intended for situations in which the error seems to have been caused by the client
So to be clear: mydomain.com/customers/id is a valid URL an the server understands the request. The fact that customer with id=5 doesn't exists has nothing to do with a "false URL" or "not understandable request".
In my opinion this should return some 2xx status code with further information inside json (definitions made by your REST API)
I would follow the meaning of HTTP status codes.
Wiki says (https://en.wikipedia.org/wiki/List_of_HTTP_status_codes):
This class of status code is intended for situations in which the error seems to have been caused by the client
So to be clear: mydomain.com/customers/id is a valid URL an the server understands the request. The fact that customer with id=5 doesn't exists has nothing to do with a "false URL" or "not understandable request".
In my opinion this should return some 2xx status code with further information inside json (definitions made by your REST API)
edited Nov 7 '18 at 14:43
answered Nov 7 '18 at 14:38
MankeldorMankeldor
13
13
1
Wikipedia is not a reference for HTTP status codes. Refer to the IANA status code registry for the atual document that defines each status code.404
, for instance, is defined in the RFC 7231, the document that defines the semantics of the HTTP/1.1.
– Cassio Mazzochi Molin
Nov 7 '18 at 14:49
1
@CassioMazzochiMolin Thanks for the link. It seems that the preferred way will be your answer. Learned from that :-)
– Mankeldor
Nov 7 '18 at 15:04
add a comment |
1
Wikipedia is not a reference for HTTP status codes. Refer to the IANA status code registry for the atual document that defines each status code.404
, for instance, is defined in the RFC 7231, the document that defines the semantics of the HTTP/1.1.
– Cassio Mazzochi Molin
Nov 7 '18 at 14:49
1
@CassioMazzochiMolin Thanks for the link. It seems that the preferred way will be your answer. Learned from that :-)
– Mankeldor
Nov 7 '18 at 15:04
1
1
Wikipedia is not a reference for HTTP status codes. Refer to the IANA status code registry for the atual document that defines each status code.
404
, for instance, is defined in the RFC 7231, the document that defines the semantics of the HTTP/1.1.– Cassio Mazzochi Molin
Nov 7 '18 at 14:49
Wikipedia is not a reference for HTTP status codes. Refer to the IANA status code registry for the atual document that defines each status code.
404
, for instance, is defined in the RFC 7231, the document that defines the semantics of the HTTP/1.1.– Cassio Mazzochi Molin
Nov 7 '18 at 14:49
1
1
@CassioMazzochiMolin Thanks for the link. It seems that the preferred way will be your answer. Learned from that :-)
– Mankeldor
Nov 7 '18 at 15:04
@CassioMazzochiMolin Thanks for the link. It seems that the preferred way will be your answer. Learned from that :-)
– Mankeldor
Nov 7 '18 at 15:04
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53191230%2fshould-http-codes-be-used-to-represent-business-failure%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
t1Usn707CxurkfGVJob,d
@pzaenger it was one of the approaches we discussed
– Matheus Cirillo
Nov 7 '18 at 14:18
While mapping HTTP operations to simple CRUD operations might sound like a good idea, HTTP operations in genearal are to general to support such a mapping blindly. I.e. DELETE only gurantees that the connection between the URL and the resource is removed but leaves the client clueless whether the server also removes the data belonging to the resource as well. A client in general is usually not interested in such internal decisions anyway as long as the resource state isn't retrievable any longer.
– Roman Vottner
Nov 7 '18 at 15:04
Further, the spec leaves room for interpretation. DELETE i.e. only states that certain response codes should be returned if the operation actually removed something but not what should happen if i.e. the resource was not available at all. Here it is more or less individual preference. All a client wants is that a certain URI shouldn't return a resource's state after the invocation. A 404 tells the client the the resource didn't exist before while a 200 response informs a client that no representation for the requeted URI is available any further. ...
– Roman Vottner
Nov 7 '18 at 15:20
... The effect, however, are pretty similar that invoking the URL doesn't return any resource's state further. It is therefore more or less an implementation detail not that important to clients
– Roman Vottner
Nov 7 '18 at 15:20