Java synchronized blocks using specific object reference
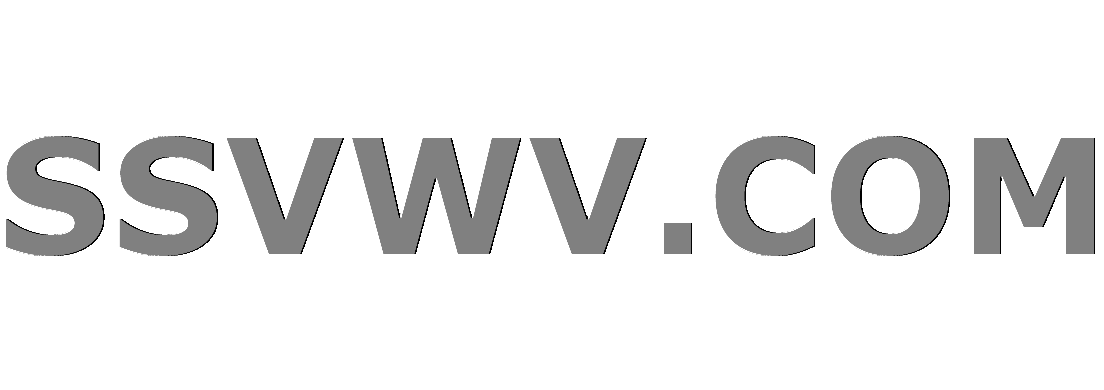
Multi tool use
I'm using the code below to block concurrent access to an Auction object. It gets the object from a hash map so it operates on a wide range of different Auctions.
I've used a synchronized block with a reference to the individual Auction object chosen as the parameter. I'm under the impression that this holds the lock from the objects monitor and will block access to threads also using the same auction (until the first case has finished).
Can anyone confirm that this code is acting in a way that 1) If two thread both reference auction A then only one may proceed at time 2) If one thread references Auction A and another Auction B then they both proceed as they acquire different locks.
//Get auction from hashmap
Auction biddingAuction = (Auction) auctions.get(ID);
//Check that auction is active
if(biddingAuction != null)
//Acquire lock on Auction object
synchronized(biddingAuction)
//Some code that alters values of Auction
else
return "nBid failed - no auction with an ID of " + ID + " was foundn";
any clarity would be appreciated, thanks
java concurrency reference synchronized
add a comment |
I'm using the code below to block concurrent access to an Auction object. It gets the object from a hash map so it operates on a wide range of different Auctions.
I've used a synchronized block with a reference to the individual Auction object chosen as the parameter. I'm under the impression that this holds the lock from the objects monitor and will block access to threads also using the same auction (until the first case has finished).
Can anyone confirm that this code is acting in a way that 1) If two thread both reference auction A then only one may proceed at time 2) If one thread references Auction A and another Auction B then they both proceed as they acquire different locks.
//Get auction from hashmap
Auction biddingAuction = (Auction) auctions.get(ID);
//Check that auction is active
if(biddingAuction != null)
//Acquire lock on Auction object
synchronized(biddingAuction)
//Some code that alters values of Auction
else
return "nBid failed - no auction with an ID of " + ID + " was foundn";
any clarity would be appreciated, thanks
java concurrency reference synchronized
add a comment |
I'm using the code below to block concurrent access to an Auction object. It gets the object from a hash map so it operates on a wide range of different Auctions.
I've used a synchronized block with a reference to the individual Auction object chosen as the parameter. I'm under the impression that this holds the lock from the objects monitor and will block access to threads also using the same auction (until the first case has finished).
Can anyone confirm that this code is acting in a way that 1) If two thread both reference auction A then only one may proceed at time 2) If one thread references Auction A and another Auction B then they both proceed as they acquire different locks.
//Get auction from hashmap
Auction biddingAuction = (Auction) auctions.get(ID);
//Check that auction is active
if(biddingAuction != null)
//Acquire lock on Auction object
synchronized(biddingAuction)
//Some code that alters values of Auction
else
return "nBid failed - no auction with an ID of " + ID + " was foundn";
any clarity would be appreciated, thanks
java concurrency reference synchronized
I'm using the code below to block concurrent access to an Auction object. It gets the object from a hash map so it operates on a wide range of different Auctions.
I've used a synchronized block with a reference to the individual Auction object chosen as the parameter. I'm under the impression that this holds the lock from the objects monitor and will block access to threads also using the same auction (until the first case has finished).
Can anyone confirm that this code is acting in a way that 1) If two thread both reference auction A then only one may proceed at time 2) If one thread references Auction A and another Auction B then they both proceed as they acquire different locks.
//Get auction from hashmap
Auction biddingAuction = (Auction) auctions.get(ID);
//Check that auction is active
if(biddingAuction != null)
//Acquire lock on Auction object
synchronized(biddingAuction)
//Some code that alters values of Auction
else
return "nBid failed - no auction with an ID of " + ID + " was foundn";
any clarity would be appreciated, thanks
java concurrency reference synchronized
java concurrency reference synchronized
asked Nov 11 '18 at 16:29


A_CarolanA_Carolan
62
62
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Can anyone confirm that this code is acting in a way that 1) If two thread both reference auction A then only one may proceed at time
No. Both threads would have to synchronize on the Auction to have that guarantee. If one thread doesn't synchronize, it can access the auction even if another thread holds its lock.
That's why such a way of doing is very fragile: if you ever forget to synchronize before accessing the Auction's mutable shared state (whether it write it or reads it), your code isn't thread-safe. A much cleaner way would be to make the Auction class itself thread-safe, by properly synchronizing the methods that access its shared mutable state.
If one thread references Auction A and another Auction B then they both proceed as they acquire different locks.
Yes. That is correct.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53250783%2fjava-synchronized-blocks-using-specific-object-reference%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Can anyone confirm that this code is acting in a way that 1) If two thread both reference auction A then only one may proceed at time
No. Both threads would have to synchronize on the Auction to have that guarantee. If one thread doesn't synchronize, it can access the auction even if another thread holds its lock.
That's why such a way of doing is very fragile: if you ever forget to synchronize before accessing the Auction's mutable shared state (whether it write it or reads it), your code isn't thread-safe. A much cleaner way would be to make the Auction class itself thread-safe, by properly synchronizing the methods that access its shared mutable state.
If one thread references Auction A and another Auction B then they both proceed as they acquire different locks.
Yes. That is correct.
add a comment |
Can anyone confirm that this code is acting in a way that 1) If two thread both reference auction A then only one may proceed at time
No. Both threads would have to synchronize on the Auction to have that guarantee. If one thread doesn't synchronize, it can access the auction even if another thread holds its lock.
That's why such a way of doing is very fragile: if you ever forget to synchronize before accessing the Auction's mutable shared state (whether it write it or reads it), your code isn't thread-safe. A much cleaner way would be to make the Auction class itself thread-safe, by properly synchronizing the methods that access its shared mutable state.
If one thread references Auction A and another Auction B then they both proceed as they acquire different locks.
Yes. That is correct.
add a comment |
Can anyone confirm that this code is acting in a way that 1) If two thread both reference auction A then only one may proceed at time
No. Both threads would have to synchronize on the Auction to have that guarantee. If one thread doesn't synchronize, it can access the auction even if another thread holds its lock.
That's why such a way of doing is very fragile: if you ever forget to synchronize before accessing the Auction's mutable shared state (whether it write it or reads it), your code isn't thread-safe. A much cleaner way would be to make the Auction class itself thread-safe, by properly synchronizing the methods that access its shared mutable state.
If one thread references Auction A and another Auction B then they both proceed as they acquire different locks.
Yes. That is correct.
Can anyone confirm that this code is acting in a way that 1) If two thread both reference auction A then only one may proceed at time
No. Both threads would have to synchronize on the Auction to have that guarantee. If one thread doesn't synchronize, it can access the auction even if another thread holds its lock.
That's why such a way of doing is very fragile: if you ever forget to synchronize before accessing the Auction's mutable shared state (whether it write it or reads it), your code isn't thread-safe. A much cleaner way would be to make the Auction class itself thread-safe, by properly synchronizing the methods that access its shared mutable state.
If one thread references Auction A and another Auction B then they both proceed as they acquire different locks.
Yes. That is correct.
answered Nov 11 '18 at 16:36
JB NizetJB Nizet
539k558731004
539k558731004
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53250783%2fjava-synchronized-blocks-using-specific-object-reference%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
TQD AjJ6BsMV,lsK4uS,b0O,p23,apLYZpTAae1n748Eqq,uz9w,A0W UNiIw