AT&T Assembly Syntax in C program (GCC compiler)?
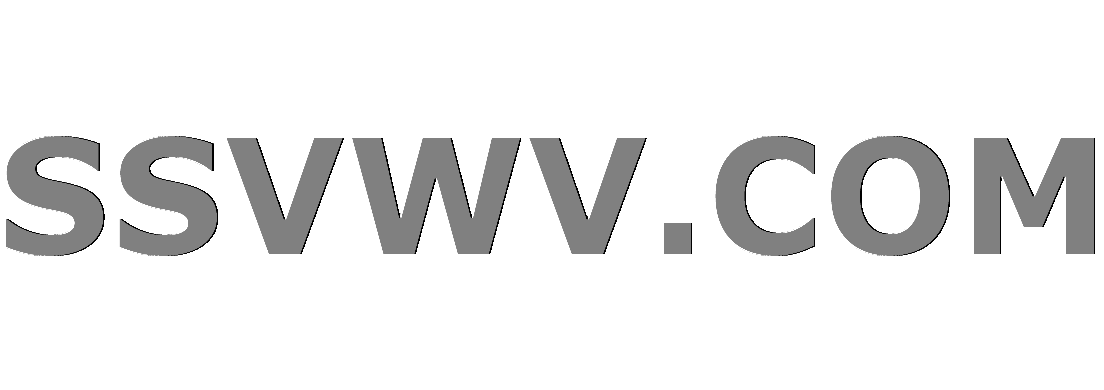
Multi tool use
I have the following program (AT&T Assembly Syntax) that works perfectly on its own if I compile it with GCC compiler on Windows x86:
LC0:
.ascii "Hello, world!"
.globl _main
_main:
pushl %ebp
movl %esp, %ebp
andl $-16, %esp
subl $16, %esp
call ___main
movl $LC0, (%esp)
call _puts
movl $0, %eax
leave
ret
Can this simple program be executed in a C program? I tried with the following:
#include <stdlib.h>
int main()
__asm__ ("LC0:nt"
".ascii 'Welcome Message'nt"
"LC1:nt"
".ascii 'Hello'nt"
"_main:nt"
"LFB11:nt"
"leal 4(%esp), %ecxnt"
"andl $-16, %espnt"
"pushl -4(%ecx)nt"
"pushl %ebpnt"
"movl %esp, %ebpnt"
"pushl %ecxnt"
"subl $20, %espnt"
"call ___mainnt"
"movl $1, 12(%esp)nt"
"movl $LC0, 8(%esp)nt"
"movl $LC1, 4(%esp)nt"
"movl $0, (%esp)nt"
"call _MessageBoxA@16nt"
"subl $16, %espnt"
"movl $0, %eaxnt"
"movl -4(%ebp), %ecxnt"
"leavent"
"leal -4(%ecx), %espnt"
"retnt");
return 0;
I get one error:
Error: junk at end of line, first unrecognized character is `8'
c gcc assembly inline-assembly
|
show 1 more comment
I have the following program (AT&T Assembly Syntax) that works perfectly on its own if I compile it with GCC compiler on Windows x86:
LC0:
.ascii "Hello, world!"
.globl _main
_main:
pushl %ebp
movl %esp, %ebp
andl $-16, %esp
subl $16, %esp
call ___main
movl $LC0, (%esp)
call _puts
movl $0, %eax
leave
ret
Can this simple program be executed in a C program? I tried with the following:
#include <stdlib.h>
int main()
__asm__ ("LC0:nt"
".ascii 'Welcome Message'nt"
"LC1:nt"
".ascii 'Hello'nt"
"_main:nt"
"LFB11:nt"
"leal 4(%esp), %ecxnt"
"andl $-16, %espnt"
"pushl -4(%ecx)nt"
"pushl %ebpnt"
"movl %esp, %ebpnt"
"pushl %ecxnt"
"subl $20, %espnt"
"call ___mainnt"
"movl $1, 12(%esp)nt"
"movl $LC0, 8(%esp)nt"
"movl $LC1, 4(%esp)nt"
"movl $0, (%esp)nt"
"call _MessageBoxA@16nt"
"subl $16, %espnt"
"movl $0, %eaxnt"
"movl -4(%ebp), %ecxnt"
"leavent"
"leal -4(%ecx), %espnt"
"retnt");
return 0;
I get one error:
Error: junk at end of line, first unrecognized character is `8'
c gcc assembly inline-assembly
@Someprogrammerdude GCC does not have an inline assembler. GCC does text replacement on your inline assembly and pastes it into its own assembly output. That said, clang does have an inline assembler which doesn't understand some constructs.
– fuz
Nov 11 '18 at 16:31
1
This isn't a Minimal, Complete, and Verifiable example because your code doesn't even include the character8
that your error message is complaining about. How exactly did your run a compiler on the file containing the__asm__
statement at global scope? And why bother putting asm at global scope instead of a separate.s
file?
– Peter Cordes
Nov 11 '18 at 16:31
The embedded''
could cause problems. You might want to try and escape it like"\0"
. Or use the.asciiz
directive instead.
– Some programmer dude
Nov 11 '18 at 16:33
Junk at the end of the line means there is ... junk at the end of the line. Check for hidden or meaningless characters at ... the end of the line.
– Rob
Nov 11 '18 at 16:33
1
And why do you want even do something like that? What is the purpose of copy some assembly directly into a C function? Why can't you keep the assembly file as it is and use it instead of wrapping the code in a C function?
– Some programmer dude
Nov 11 '18 at 16:35
|
show 1 more comment
I have the following program (AT&T Assembly Syntax) that works perfectly on its own if I compile it with GCC compiler on Windows x86:
LC0:
.ascii "Hello, world!"
.globl _main
_main:
pushl %ebp
movl %esp, %ebp
andl $-16, %esp
subl $16, %esp
call ___main
movl $LC0, (%esp)
call _puts
movl $0, %eax
leave
ret
Can this simple program be executed in a C program? I tried with the following:
#include <stdlib.h>
int main()
__asm__ ("LC0:nt"
".ascii 'Welcome Message'nt"
"LC1:nt"
".ascii 'Hello'nt"
"_main:nt"
"LFB11:nt"
"leal 4(%esp), %ecxnt"
"andl $-16, %espnt"
"pushl -4(%ecx)nt"
"pushl %ebpnt"
"movl %esp, %ebpnt"
"pushl %ecxnt"
"subl $20, %espnt"
"call ___mainnt"
"movl $1, 12(%esp)nt"
"movl $LC0, 8(%esp)nt"
"movl $LC1, 4(%esp)nt"
"movl $0, (%esp)nt"
"call _MessageBoxA@16nt"
"subl $16, %espnt"
"movl $0, %eaxnt"
"movl -4(%ebp), %ecxnt"
"leavent"
"leal -4(%ecx), %espnt"
"retnt");
return 0;
I get one error:
Error: junk at end of line, first unrecognized character is `8'
c gcc assembly inline-assembly
I have the following program (AT&T Assembly Syntax) that works perfectly on its own if I compile it with GCC compiler on Windows x86:
LC0:
.ascii "Hello, world!"
.globl _main
_main:
pushl %ebp
movl %esp, %ebp
andl $-16, %esp
subl $16, %esp
call ___main
movl $LC0, (%esp)
call _puts
movl $0, %eax
leave
ret
Can this simple program be executed in a C program? I tried with the following:
#include <stdlib.h>
int main()
__asm__ ("LC0:nt"
".ascii 'Welcome Message'nt"
"LC1:nt"
".ascii 'Hello'nt"
"_main:nt"
"LFB11:nt"
"leal 4(%esp), %ecxnt"
"andl $-16, %espnt"
"pushl -4(%ecx)nt"
"pushl %ebpnt"
"movl %esp, %ebpnt"
"pushl %ecxnt"
"subl $20, %espnt"
"call ___mainnt"
"movl $1, 12(%esp)nt"
"movl $LC0, 8(%esp)nt"
"movl $LC1, 4(%esp)nt"
"movl $0, (%esp)nt"
"call _MessageBoxA@16nt"
"subl $16, %espnt"
"movl $0, %eaxnt"
"movl -4(%ebp), %ecxnt"
"leavent"
"leal -4(%ecx), %espnt"
"retnt");
return 0;
I get one error:
Error: junk at end of line, first unrecognized character is `8'
c gcc assembly inline-assembly
c gcc assembly inline-assembly
edited Nov 11 '18 at 16:40
Lavonen
asked Nov 11 '18 at 16:27
LavonenLavonen
169211
169211
@Someprogrammerdude GCC does not have an inline assembler. GCC does text replacement on your inline assembly and pastes it into its own assembly output. That said, clang does have an inline assembler which doesn't understand some constructs.
– fuz
Nov 11 '18 at 16:31
1
This isn't a Minimal, Complete, and Verifiable example because your code doesn't even include the character8
that your error message is complaining about. How exactly did your run a compiler on the file containing the__asm__
statement at global scope? And why bother putting asm at global scope instead of a separate.s
file?
– Peter Cordes
Nov 11 '18 at 16:31
The embedded''
could cause problems. You might want to try and escape it like"\0"
. Or use the.asciiz
directive instead.
– Some programmer dude
Nov 11 '18 at 16:33
Junk at the end of the line means there is ... junk at the end of the line. Check for hidden or meaningless characters at ... the end of the line.
– Rob
Nov 11 '18 at 16:33
1
And why do you want even do something like that? What is the purpose of copy some assembly directly into a C function? Why can't you keep the assembly file as it is and use it instead of wrapping the code in a C function?
– Some programmer dude
Nov 11 '18 at 16:35
|
show 1 more comment
@Someprogrammerdude GCC does not have an inline assembler. GCC does text replacement on your inline assembly and pastes it into its own assembly output. That said, clang does have an inline assembler which doesn't understand some constructs.
– fuz
Nov 11 '18 at 16:31
1
This isn't a Minimal, Complete, and Verifiable example because your code doesn't even include the character8
that your error message is complaining about. How exactly did your run a compiler on the file containing the__asm__
statement at global scope? And why bother putting asm at global scope instead of a separate.s
file?
– Peter Cordes
Nov 11 '18 at 16:31
The embedded''
could cause problems. You might want to try and escape it like"\0"
. Or use the.asciiz
directive instead.
– Some programmer dude
Nov 11 '18 at 16:33
Junk at the end of the line means there is ... junk at the end of the line. Check for hidden or meaningless characters at ... the end of the line.
– Rob
Nov 11 '18 at 16:33
1
And why do you want even do something like that? What is the purpose of copy some assembly directly into a C function? Why can't you keep the assembly file as it is and use it instead of wrapping the code in a C function?
– Some programmer dude
Nov 11 '18 at 16:35
@Someprogrammerdude GCC does not have an inline assembler. GCC does text replacement on your inline assembly and pastes it into its own assembly output. That said, clang does have an inline assembler which doesn't understand some constructs.
– fuz
Nov 11 '18 at 16:31
@Someprogrammerdude GCC does not have an inline assembler. GCC does text replacement on your inline assembly and pastes it into its own assembly output. That said, clang does have an inline assembler which doesn't understand some constructs.
– fuz
Nov 11 '18 at 16:31
1
1
This isn't a Minimal, Complete, and Verifiable example because your code doesn't even include the character
8
that your error message is complaining about. How exactly did your run a compiler on the file containing the __asm__
statement at global scope? And why bother putting asm at global scope instead of a separate .s
file?– Peter Cordes
Nov 11 '18 at 16:31
This isn't a Minimal, Complete, and Verifiable example because your code doesn't even include the character
8
that your error message is complaining about. How exactly did your run a compiler on the file containing the __asm__
statement at global scope? And why bother putting asm at global scope instead of a separate .s
file?– Peter Cordes
Nov 11 '18 at 16:31
The embedded
''
could cause problems. You might want to try and escape it like "\0"
. Or use the .asciiz
directive instead.– Some programmer dude
Nov 11 '18 at 16:33
The embedded
''
could cause problems. You might want to try and escape it like "\0"
. Or use the .asciiz
directive instead.– Some programmer dude
Nov 11 '18 at 16:33
Junk at the end of the line means there is ... junk at the end of the line. Check for hidden or meaningless characters at ... the end of the line.
– Rob
Nov 11 '18 at 16:33
Junk at the end of the line means there is ... junk at the end of the line. Check for hidden or meaningless characters at ... the end of the line.
– Rob
Nov 11 '18 at 16:33
1
1
And why do you want even do something like that? What is the purpose of copy some assembly directly into a C function? Why can't you keep the assembly file as it is and use it instead of wrapping the code in a C function?
– Some programmer dude
Nov 11 '18 at 16:35
And why do you want even do something like that? What is the purpose of copy some assembly directly into a C function? Why can't you keep the assembly file as it is and use it instead of wrapping the code in a C function?
– Some programmer dude
Nov 11 '18 at 16:35
|
show 1 more comment
1 Answer
1
active
oldest
votes
This works fine:
__asm__(
"LC0:n"
" .ascii "Hello, world!\0"n"
".globl _mainn"
"_main:n"
" pushl %ebpn"
" movl %esp, %ebpn"
" andl $-16, %espn"
" subl $16, %espn"
" call ___mainn"
" movl $LC0, (%esp)n"
" call _putsn"
" movl $0, %eaxn"
" leaven"
" retn"
);
Simply C-string-literal-escaped the double quoted string and 's/^/"/;s/$/\n"/'
elsewhere.
My gcc's assembler doesn't accept single quoted string literals as in
LC0:
.ascii 'Hello, world!'
.globl _main
_main:
pushl %ebp
movl %esp, %ebp
andl $-16, %esp
subl $16, %esp
call ___main
movl $LC0, (%esp)
call _puts
movl $0, %eax
leave
ret
so I don't see why it should start accepting them if you supply them through __asm__
.
I tried the first example, received error - "symbol `_main' is already defined". Did it work for you on your computer?
– Lavonen
Nov 11 '18 at 16:39
@Lavonen Did you add any other code when compiling this? Such as, a definition of amain
function? Because that's of course not going to work. Put theasm
statement outside of any function.
– fuz
Nov 11 '18 at 16:40
1
@Lavonen: you probably put that inside a C definition of amain
function like in your question. That's incorrect, and not what PSkocik is doing. Put the asm at global scope, or use__attribute__((naked))
if you want to write the function prologue and theret
yourself. Looking at the C compiler's asm output from source that contains inline asm is useful when debugging inline asm (especially with constraints, but also in this case.)
– Peter Cordes
Nov 11 '18 at 16:41
1
BTW, the normal way to make zero-terminated strings is with.asciz
aka.asciiz
, rather than putting ainside the quoted string.
– Peter Cordes
Nov 11 '18 at 16:42
1
Now it works, I had the code inside a function. Didn't understand it has to be outside for whatever reason. Thank you for an awesome answer, will vote it up!))
– Lavonen
Nov 11 '18 at 16:43
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53250768%2fatt-assembly-syntax-in-c-program-gcc-compiler%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This works fine:
__asm__(
"LC0:n"
" .ascii "Hello, world!\0"n"
".globl _mainn"
"_main:n"
" pushl %ebpn"
" movl %esp, %ebpn"
" andl $-16, %espn"
" subl $16, %espn"
" call ___mainn"
" movl $LC0, (%esp)n"
" call _putsn"
" movl $0, %eaxn"
" leaven"
" retn"
);
Simply C-string-literal-escaped the double quoted string and 's/^/"/;s/$/\n"/'
elsewhere.
My gcc's assembler doesn't accept single quoted string literals as in
LC0:
.ascii 'Hello, world!'
.globl _main
_main:
pushl %ebp
movl %esp, %ebp
andl $-16, %esp
subl $16, %esp
call ___main
movl $LC0, (%esp)
call _puts
movl $0, %eax
leave
ret
so I don't see why it should start accepting them if you supply them through __asm__
.
I tried the first example, received error - "symbol `_main' is already defined". Did it work for you on your computer?
– Lavonen
Nov 11 '18 at 16:39
@Lavonen Did you add any other code when compiling this? Such as, a definition of amain
function? Because that's of course not going to work. Put theasm
statement outside of any function.
– fuz
Nov 11 '18 at 16:40
1
@Lavonen: you probably put that inside a C definition of amain
function like in your question. That's incorrect, and not what PSkocik is doing. Put the asm at global scope, or use__attribute__((naked))
if you want to write the function prologue and theret
yourself. Looking at the C compiler's asm output from source that contains inline asm is useful when debugging inline asm (especially with constraints, but also in this case.)
– Peter Cordes
Nov 11 '18 at 16:41
1
BTW, the normal way to make zero-terminated strings is with.asciz
aka.asciiz
, rather than putting ainside the quoted string.
– Peter Cordes
Nov 11 '18 at 16:42
1
Now it works, I had the code inside a function. Didn't understand it has to be outside for whatever reason. Thank you for an awesome answer, will vote it up!))
– Lavonen
Nov 11 '18 at 16:43
|
show 1 more comment
This works fine:
__asm__(
"LC0:n"
" .ascii "Hello, world!\0"n"
".globl _mainn"
"_main:n"
" pushl %ebpn"
" movl %esp, %ebpn"
" andl $-16, %espn"
" subl $16, %espn"
" call ___mainn"
" movl $LC0, (%esp)n"
" call _putsn"
" movl $0, %eaxn"
" leaven"
" retn"
);
Simply C-string-literal-escaped the double quoted string and 's/^/"/;s/$/\n"/'
elsewhere.
My gcc's assembler doesn't accept single quoted string literals as in
LC0:
.ascii 'Hello, world!'
.globl _main
_main:
pushl %ebp
movl %esp, %ebp
andl $-16, %esp
subl $16, %esp
call ___main
movl $LC0, (%esp)
call _puts
movl $0, %eax
leave
ret
so I don't see why it should start accepting them if you supply them through __asm__
.
I tried the first example, received error - "symbol `_main' is already defined". Did it work for you on your computer?
– Lavonen
Nov 11 '18 at 16:39
@Lavonen Did you add any other code when compiling this? Such as, a definition of amain
function? Because that's of course not going to work. Put theasm
statement outside of any function.
– fuz
Nov 11 '18 at 16:40
1
@Lavonen: you probably put that inside a C definition of amain
function like in your question. That's incorrect, and not what PSkocik is doing. Put the asm at global scope, or use__attribute__((naked))
if you want to write the function prologue and theret
yourself. Looking at the C compiler's asm output from source that contains inline asm is useful when debugging inline asm (especially with constraints, but also in this case.)
– Peter Cordes
Nov 11 '18 at 16:41
1
BTW, the normal way to make zero-terminated strings is with.asciz
aka.asciiz
, rather than putting ainside the quoted string.
– Peter Cordes
Nov 11 '18 at 16:42
1
Now it works, I had the code inside a function. Didn't understand it has to be outside for whatever reason. Thank you for an awesome answer, will vote it up!))
– Lavonen
Nov 11 '18 at 16:43
|
show 1 more comment
This works fine:
__asm__(
"LC0:n"
" .ascii "Hello, world!\0"n"
".globl _mainn"
"_main:n"
" pushl %ebpn"
" movl %esp, %ebpn"
" andl $-16, %espn"
" subl $16, %espn"
" call ___mainn"
" movl $LC0, (%esp)n"
" call _putsn"
" movl $0, %eaxn"
" leaven"
" retn"
);
Simply C-string-literal-escaped the double quoted string and 's/^/"/;s/$/\n"/'
elsewhere.
My gcc's assembler doesn't accept single quoted string literals as in
LC0:
.ascii 'Hello, world!'
.globl _main
_main:
pushl %ebp
movl %esp, %ebp
andl $-16, %esp
subl $16, %esp
call ___main
movl $LC0, (%esp)
call _puts
movl $0, %eax
leave
ret
so I don't see why it should start accepting them if you supply them through __asm__
.
This works fine:
__asm__(
"LC0:n"
" .ascii "Hello, world!\0"n"
".globl _mainn"
"_main:n"
" pushl %ebpn"
" movl %esp, %ebpn"
" andl $-16, %espn"
" subl $16, %espn"
" call ___mainn"
" movl $LC0, (%esp)n"
" call _putsn"
" movl $0, %eaxn"
" leaven"
" retn"
);
Simply C-string-literal-escaped the double quoted string and 's/^/"/;s/$/\n"/'
elsewhere.
My gcc's assembler doesn't accept single quoted string literals as in
LC0:
.ascii 'Hello, world!'
.globl _main
_main:
pushl %ebp
movl %esp, %ebp
andl $-16, %esp
subl $16, %esp
call ___main
movl $LC0, (%esp)
call _puts
movl $0, %eax
leave
ret
so I don't see why it should start accepting them if you supply them through __asm__
.
answered Nov 11 '18 at 16:33
PSkocikPSkocik
33.1k65173
33.1k65173
I tried the first example, received error - "symbol `_main' is already defined". Did it work for you on your computer?
– Lavonen
Nov 11 '18 at 16:39
@Lavonen Did you add any other code when compiling this? Such as, a definition of amain
function? Because that's of course not going to work. Put theasm
statement outside of any function.
– fuz
Nov 11 '18 at 16:40
1
@Lavonen: you probably put that inside a C definition of amain
function like in your question. That's incorrect, and not what PSkocik is doing. Put the asm at global scope, or use__attribute__((naked))
if you want to write the function prologue and theret
yourself. Looking at the C compiler's asm output from source that contains inline asm is useful when debugging inline asm (especially with constraints, but also in this case.)
– Peter Cordes
Nov 11 '18 at 16:41
1
BTW, the normal way to make zero-terminated strings is with.asciz
aka.asciiz
, rather than putting ainside the quoted string.
– Peter Cordes
Nov 11 '18 at 16:42
1
Now it works, I had the code inside a function. Didn't understand it has to be outside for whatever reason. Thank you for an awesome answer, will vote it up!))
– Lavonen
Nov 11 '18 at 16:43
|
show 1 more comment
I tried the first example, received error - "symbol `_main' is already defined". Did it work for you on your computer?
– Lavonen
Nov 11 '18 at 16:39
@Lavonen Did you add any other code when compiling this? Such as, a definition of amain
function? Because that's of course not going to work. Put theasm
statement outside of any function.
– fuz
Nov 11 '18 at 16:40
1
@Lavonen: you probably put that inside a C definition of amain
function like in your question. That's incorrect, and not what PSkocik is doing. Put the asm at global scope, or use__attribute__((naked))
if you want to write the function prologue and theret
yourself. Looking at the C compiler's asm output from source that contains inline asm is useful when debugging inline asm (especially with constraints, but also in this case.)
– Peter Cordes
Nov 11 '18 at 16:41
1
BTW, the normal way to make zero-terminated strings is with.asciz
aka.asciiz
, rather than putting ainside the quoted string.
– Peter Cordes
Nov 11 '18 at 16:42
1
Now it works, I had the code inside a function. Didn't understand it has to be outside for whatever reason. Thank you for an awesome answer, will vote it up!))
– Lavonen
Nov 11 '18 at 16:43
I tried the first example, received error - "symbol `_main' is already defined". Did it work for you on your computer?
– Lavonen
Nov 11 '18 at 16:39
I tried the first example, received error - "symbol `_main' is already defined". Did it work for you on your computer?
– Lavonen
Nov 11 '18 at 16:39
@Lavonen Did you add any other code when compiling this? Such as, a definition of a
main
function? Because that's of course not going to work. Put the asm
statement outside of any function.– fuz
Nov 11 '18 at 16:40
@Lavonen Did you add any other code when compiling this? Such as, a definition of a
main
function? Because that's of course not going to work. Put the asm
statement outside of any function.– fuz
Nov 11 '18 at 16:40
1
1
@Lavonen: you probably put that inside a C definition of a
main
function like in your question. That's incorrect, and not what PSkocik is doing. Put the asm at global scope, or use __attribute__((naked))
if you want to write the function prologue and the ret
yourself. Looking at the C compiler's asm output from source that contains inline asm is useful when debugging inline asm (especially with constraints, but also in this case.)– Peter Cordes
Nov 11 '18 at 16:41
@Lavonen: you probably put that inside a C definition of a
main
function like in your question. That's incorrect, and not what PSkocik is doing. Put the asm at global scope, or use __attribute__((naked))
if you want to write the function prologue and the ret
yourself. Looking at the C compiler's asm output from source that contains inline asm is useful when debugging inline asm (especially with constraints, but also in this case.)– Peter Cordes
Nov 11 '18 at 16:41
1
1
BTW, the normal way to make zero-terminated strings is with
.asciz
aka .asciiz
, rather than putting a
inside the quoted string.– Peter Cordes
Nov 11 '18 at 16:42
BTW, the normal way to make zero-terminated strings is with
.asciz
aka .asciiz
, rather than putting a
inside the quoted string.– Peter Cordes
Nov 11 '18 at 16:42
1
1
Now it works, I had the code inside a function. Didn't understand it has to be outside for whatever reason. Thank you for an awesome answer, will vote it up!))
– Lavonen
Nov 11 '18 at 16:43
Now it works, I had the code inside a function. Didn't understand it has to be outside for whatever reason. Thank you for an awesome answer, will vote it up!))
– Lavonen
Nov 11 '18 at 16:43
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53250768%2fatt-assembly-syntax-in-c-program-gcc-compiler%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7YKlP,2 jpxZyzV9a2QYXfW
@Someprogrammerdude GCC does not have an inline assembler. GCC does text replacement on your inline assembly and pastes it into its own assembly output. That said, clang does have an inline assembler which doesn't understand some constructs.
– fuz
Nov 11 '18 at 16:31
1
This isn't a Minimal, Complete, and Verifiable example because your code doesn't even include the character
8
that your error message is complaining about. How exactly did your run a compiler on the file containing the__asm__
statement at global scope? And why bother putting asm at global scope instead of a separate.s
file?– Peter Cordes
Nov 11 '18 at 16:31
The embedded
''
could cause problems. You might want to try and escape it like"\0"
. Or use the.asciiz
directive instead.– Some programmer dude
Nov 11 '18 at 16:33
Junk at the end of the line means there is ... junk at the end of the line. Check for hidden or meaningless characters at ... the end of the line.
– Rob
Nov 11 '18 at 16:33
1
And why do you want even do something like that? What is the purpose of copy some assembly directly into a C function? Why can't you keep the assembly file as it is and use it instead of wrapping the code in a C function?
– Some programmer dude
Nov 11 '18 at 16:35