Node.Js function runs twice when called once on promise return
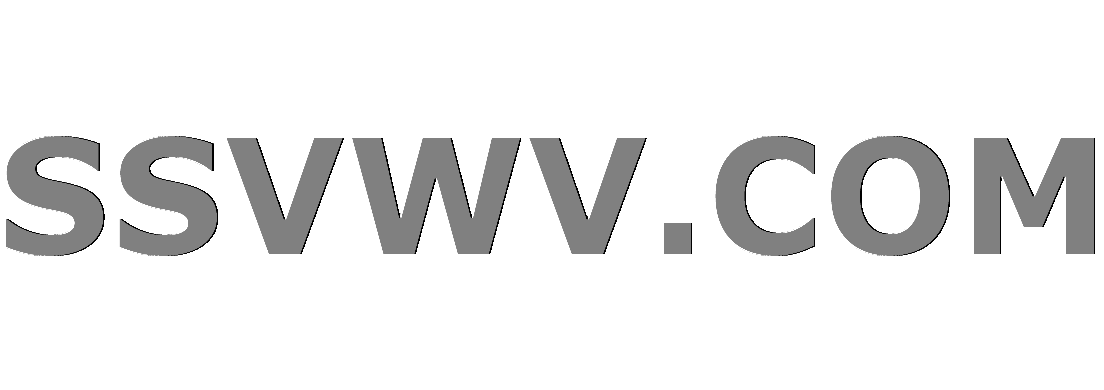
Multi tool use
up vote
1
down vote
favorite
I am attempting to create as many Google Slide slides as needed based on user input. In theory, my code should work if I can fix this one problem. The problem is, I can't. When I run my code, it stops because the createSlide() function runs twice on one call and creates two slides with the same Id. Poking around on the web, I've only found things about Node.Js input from a browser. First time through it runs ok, it does what it's supposed to, but when slide two (id: slide_1) is created it makes the slide, doubles the text such as:
Hi
bye
Would create
Hi
bye
Hi
bye
Then stop due to the fact that there cannot be two Ids that are the same. Here is my code (I've deleted the requests since the slide creation works fine):
function askYOrN(auth)
console.log("HELLO");
const r4 = readline.createInterface(
input: process.stdin,
output: process.stdout
);
r4.question("Do you want to add a slide [Y/N]? ", function(yesOrNo)
yesOrNoLog = yesOrNo;
r4.close();
fAskForNewCounter = fAskForNewCounter + 1;
askForNew(auth);
);
//ASKS IF ANOTHER SLIDE IS NEEDED
function askForNew(auth)
//DECLARATION FOR THE NUMBER COUNTER [ num ]
var num = 0;
function createSlide(auth)
//AUTHENTICATION
const slides = google.slides(version: 'v1', auth);
//CHANGES txtArray TO STRING
var txt = txtArray.join('');
//CHANGING VARS
var slideId = 'slide_' + num;
var pageId = slideId;
var textId = 'text_box_' + num;
var elementId = textId;
var iIndex = num;
//SLIDE NUMBER
var sNum = num + 1;
console.log(pageId);
//ALL REQUESTS GO IN requests
var requests = [
];
//BATCH UPDATE
return slides.presentations.batchUpdate(
presentationId,
resource:
requests,
,
, (err, res) =>
if (err)
error(err);
else
console.log("Slide #" + sNum + " has been created");
//INCREASES COUNTER BY 1
num = num + 1;
//ASKS IF A NEW SLIDE WANTS TO BE CREATED
var delay = 30;
var lastClick = 0;
if (lastClick >= (Date.now() - delay))
return;
lastClick = Date.now();
yesOrNoLog = "";
askYOrNo(auth);
);
Thanks in advance for your help!
EDIT
I have done some more work and have found that in my code r5.on is run twice. I tried deleting the array.push("n"). but this does not fix the problem. The array outputs as
[hi, bye, hi, bye]
This is my new code once again I have deleted the requests:
//ASKS IF YOU WANT A NEW SLIDE
function askYOrN(auth)
console.log("askYOrN");
const r4 = readline.createInterface(
input: process.stdin,
output: process.stdout,
terminal: false
);
r4.question("Do you want to add a slide [Y/N]? ", function(yesOrNo)
console.log("aksYOrN question");
yesOrNoLog = yesOrNo;
r4.close();
yOrNCheckCounter = yOrNCheckCounter + 1;
askYOrNCheck(auth);
);
//ASKS IF ANOTHER SLIDE IS NEEDED
function askYOrNCheck(auth)
//DECLARATION FOR THE NUMBER COUNTER [ num ]
var num = 0;
function createSlide(auth)
//AUTHENTICATION
const slides = google.slides(version: 'v1', auth);
//CHANGES txtArray TO STRING
var text = txtArray.join('n');
//CHANGING VARS
var slideId = 'slide_' + num;
var pageId = slideId;
var textId = 'text_box_' + num;
var elementId = textId;
var iIndex = num;
//SLIDE NUMBER
var sNum = num + 1;
console.log(pageId);
//ALL REQUESTS GO IN [ requests ]
var requests = ;
//BATCH UPDATE
return slides.presentations.batchUpdate(
presentationId,
resource:
requests,
,
, (err, res) =>
if (err) return error(err);
console.log("Slide #" + sNum + " has been created");
//INCREASES COUNTER BY 1
num = num + 1;
//ASKS IF A NEW SLIDE WANTS TO BE CREATED
askYOrN(auth);
);
Note:
I renamed askForNew() to askYOrNoCheck().
Once again thanks for your help.
javascript node.js

add a comment |
up vote
1
down vote
favorite
I am attempting to create as many Google Slide slides as needed based on user input. In theory, my code should work if I can fix this one problem. The problem is, I can't. When I run my code, it stops because the createSlide() function runs twice on one call and creates two slides with the same Id. Poking around on the web, I've only found things about Node.Js input from a browser. First time through it runs ok, it does what it's supposed to, but when slide two (id: slide_1) is created it makes the slide, doubles the text such as:
Hi
bye
Would create
Hi
bye
Hi
bye
Then stop due to the fact that there cannot be two Ids that are the same. Here is my code (I've deleted the requests since the slide creation works fine):
function askYOrN(auth)
console.log("HELLO");
const r4 = readline.createInterface(
input: process.stdin,
output: process.stdout
);
r4.question("Do you want to add a slide [Y/N]? ", function(yesOrNo)
yesOrNoLog = yesOrNo;
r4.close();
fAskForNewCounter = fAskForNewCounter + 1;
askForNew(auth);
);
//ASKS IF ANOTHER SLIDE IS NEEDED
function askForNew(auth)
//DECLARATION FOR THE NUMBER COUNTER [ num ]
var num = 0;
function createSlide(auth)
//AUTHENTICATION
const slides = google.slides(version: 'v1', auth);
//CHANGES txtArray TO STRING
var txt = txtArray.join('');
//CHANGING VARS
var slideId = 'slide_' + num;
var pageId = slideId;
var textId = 'text_box_' + num;
var elementId = textId;
var iIndex = num;
//SLIDE NUMBER
var sNum = num + 1;
console.log(pageId);
//ALL REQUESTS GO IN requests
var requests = [
];
//BATCH UPDATE
return slides.presentations.batchUpdate(
presentationId,
resource:
requests,
,
, (err, res) =>
if (err)
error(err);
else
console.log("Slide #" + sNum + " has been created");
//INCREASES COUNTER BY 1
num = num + 1;
//ASKS IF A NEW SLIDE WANTS TO BE CREATED
var delay = 30;
var lastClick = 0;
if (lastClick >= (Date.now() - delay))
return;
lastClick = Date.now();
yesOrNoLog = "";
askYOrNo(auth);
);
Thanks in advance for your help!
EDIT
I have done some more work and have found that in my code r5.on is run twice. I tried deleting the array.push("n"). but this does not fix the problem. The array outputs as
[hi, bye, hi, bye]
This is my new code once again I have deleted the requests:
//ASKS IF YOU WANT A NEW SLIDE
function askYOrN(auth)
console.log("askYOrN");
const r4 = readline.createInterface(
input: process.stdin,
output: process.stdout,
terminal: false
);
r4.question("Do you want to add a slide [Y/N]? ", function(yesOrNo)
console.log("aksYOrN question");
yesOrNoLog = yesOrNo;
r4.close();
yOrNCheckCounter = yOrNCheckCounter + 1;
askYOrNCheck(auth);
);
//ASKS IF ANOTHER SLIDE IS NEEDED
function askYOrNCheck(auth)
//DECLARATION FOR THE NUMBER COUNTER [ num ]
var num = 0;
function createSlide(auth)
//AUTHENTICATION
const slides = google.slides(version: 'v1', auth);
//CHANGES txtArray TO STRING
var text = txtArray.join('n');
//CHANGING VARS
var slideId = 'slide_' + num;
var pageId = slideId;
var textId = 'text_box_' + num;
var elementId = textId;
var iIndex = num;
//SLIDE NUMBER
var sNum = num + 1;
console.log(pageId);
//ALL REQUESTS GO IN [ requests ]
var requests = ;
//BATCH UPDATE
return slides.presentations.batchUpdate(
presentationId,
resource:
requests,
,
, (err, res) =>
if (err) return error(err);
console.log("Slide #" + sNum + " has been created");
//INCREASES COUNTER BY 1
num = num + 1;
//ASKS IF A NEW SLIDE WANTS TO BE CREATED
askYOrN(auth);
);
Note:
I renamed askForNew() to askYOrNoCheck().
Once again thanks for your help.
javascript node.js

I highly recommend checking out Inquirer
– FrankerZ
Nov 13 at 0:57
First, I would suggest getting rid of your global variables. Without looking much at the code, it could either cause your current problem or future problems. Instead, pass the necessary variables around (e.g.checkAnswer(providedAnswer) /* ... */
). Second, make sure to separate your functions into meaningful units. For example,askYOrNCheck()
performs 2 actions: checking the user's answer and prompting for the slide text. This will help you think about what you're doing more clearly. Third, consider revisiting your names (e.g.askYOrNCheck
should becheckAnswer
).
– c1moore
Nov 13 at 1:21
The last tip makes the intent of the function more clear. The first few times I read it, I assumed you were going to prompt for the user's answer. While these don't solve your problem, addressing them will make it easier for others to help you and may help you figure out what is happening yourself because your program will be more semantically meaningful and better defined.
– c1moore
Nov 13 at 1:23
Actually, instead ofcheckAnswer
, you may want to use something likeshouldAddSlide(providedAnswer)
that returnstrue
if the user enteredyes
ory
,false
if the user enteredno
orn
, or throws an error if the user entered anything else.
– c1moore
Nov 13 at 1:28
@c1moore Thank you for your suggestions I will definitely go through my code. However I have found the issue, I forgot to close the readline so it was pushing 2 answers instead of one.
– Arkin Solomon
Nov 13 at 1:41
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am attempting to create as many Google Slide slides as needed based on user input. In theory, my code should work if I can fix this one problem. The problem is, I can't. When I run my code, it stops because the createSlide() function runs twice on one call and creates two slides with the same Id. Poking around on the web, I've only found things about Node.Js input from a browser. First time through it runs ok, it does what it's supposed to, but when slide two (id: slide_1) is created it makes the slide, doubles the text such as:
Hi
bye
Would create
Hi
bye
Hi
bye
Then stop due to the fact that there cannot be two Ids that are the same. Here is my code (I've deleted the requests since the slide creation works fine):
function askYOrN(auth)
console.log("HELLO");
const r4 = readline.createInterface(
input: process.stdin,
output: process.stdout
);
r4.question("Do you want to add a slide [Y/N]? ", function(yesOrNo)
yesOrNoLog = yesOrNo;
r4.close();
fAskForNewCounter = fAskForNewCounter + 1;
askForNew(auth);
);
//ASKS IF ANOTHER SLIDE IS NEEDED
function askForNew(auth)
//DECLARATION FOR THE NUMBER COUNTER [ num ]
var num = 0;
function createSlide(auth)
//AUTHENTICATION
const slides = google.slides(version: 'v1', auth);
//CHANGES txtArray TO STRING
var txt = txtArray.join('');
//CHANGING VARS
var slideId = 'slide_' + num;
var pageId = slideId;
var textId = 'text_box_' + num;
var elementId = textId;
var iIndex = num;
//SLIDE NUMBER
var sNum = num + 1;
console.log(pageId);
//ALL REQUESTS GO IN requests
var requests = [
];
//BATCH UPDATE
return slides.presentations.batchUpdate(
presentationId,
resource:
requests,
,
, (err, res) =>
if (err)
error(err);
else
console.log("Slide #" + sNum + " has been created");
//INCREASES COUNTER BY 1
num = num + 1;
//ASKS IF A NEW SLIDE WANTS TO BE CREATED
var delay = 30;
var lastClick = 0;
if (lastClick >= (Date.now() - delay))
return;
lastClick = Date.now();
yesOrNoLog = "";
askYOrNo(auth);
);
Thanks in advance for your help!
EDIT
I have done some more work and have found that in my code r5.on is run twice. I tried deleting the array.push("n"). but this does not fix the problem. The array outputs as
[hi, bye, hi, bye]
This is my new code once again I have deleted the requests:
//ASKS IF YOU WANT A NEW SLIDE
function askYOrN(auth)
console.log("askYOrN");
const r4 = readline.createInterface(
input: process.stdin,
output: process.stdout,
terminal: false
);
r4.question("Do you want to add a slide [Y/N]? ", function(yesOrNo)
console.log("aksYOrN question");
yesOrNoLog = yesOrNo;
r4.close();
yOrNCheckCounter = yOrNCheckCounter + 1;
askYOrNCheck(auth);
);
//ASKS IF ANOTHER SLIDE IS NEEDED
function askYOrNCheck(auth)
//DECLARATION FOR THE NUMBER COUNTER [ num ]
var num = 0;
function createSlide(auth)
//AUTHENTICATION
const slides = google.slides(version: 'v1', auth);
//CHANGES txtArray TO STRING
var text = txtArray.join('n');
//CHANGING VARS
var slideId = 'slide_' + num;
var pageId = slideId;
var textId = 'text_box_' + num;
var elementId = textId;
var iIndex = num;
//SLIDE NUMBER
var sNum = num + 1;
console.log(pageId);
//ALL REQUESTS GO IN [ requests ]
var requests = ;
//BATCH UPDATE
return slides.presentations.batchUpdate(
presentationId,
resource:
requests,
,
, (err, res) =>
if (err) return error(err);
console.log("Slide #" + sNum + " has been created");
//INCREASES COUNTER BY 1
num = num + 1;
//ASKS IF A NEW SLIDE WANTS TO BE CREATED
askYOrN(auth);
);
Note:
I renamed askForNew() to askYOrNoCheck().
Once again thanks for your help.
javascript node.js

I am attempting to create as many Google Slide slides as needed based on user input. In theory, my code should work if I can fix this one problem. The problem is, I can't. When I run my code, it stops because the createSlide() function runs twice on one call and creates two slides with the same Id. Poking around on the web, I've only found things about Node.Js input from a browser. First time through it runs ok, it does what it's supposed to, but when slide two (id: slide_1) is created it makes the slide, doubles the text such as:
Hi
bye
Would create
Hi
bye
Hi
bye
Then stop due to the fact that there cannot be two Ids that are the same. Here is my code (I've deleted the requests since the slide creation works fine):
function askYOrN(auth)
console.log("HELLO");
const r4 = readline.createInterface(
input: process.stdin,
output: process.stdout
);
r4.question("Do you want to add a slide [Y/N]? ", function(yesOrNo)
yesOrNoLog = yesOrNo;
r4.close();
fAskForNewCounter = fAskForNewCounter + 1;
askForNew(auth);
);
//ASKS IF ANOTHER SLIDE IS NEEDED
function askForNew(auth)
//DECLARATION FOR THE NUMBER COUNTER [ num ]
var num = 0;
function createSlide(auth)
//AUTHENTICATION
const slides = google.slides(version: 'v1', auth);
//CHANGES txtArray TO STRING
var txt = txtArray.join('');
//CHANGING VARS
var slideId = 'slide_' + num;
var pageId = slideId;
var textId = 'text_box_' + num;
var elementId = textId;
var iIndex = num;
//SLIDE NUMBER
var sNum = num + 1;
console.log(pageId);
//ALL REQUESTS GO IN requests
var requests = [
];
//BATCH UPDATE
return slides.presentations.batchUpdate(
presentationId,
resource:
requests,
,
, (err, res) =>
if (err)
error(err);
else
console.log("Slide #" + sNum + " has been created");
//INCREASES COUNTER BY 1
num = num + 1;
//ASKS IF A NEW SLIDE WANTS TO BE CREATED
var delay = 30;
var lastClick = 0;
if (lastClick >= (Date.now() - delay))
return;
lastClick = Date.now();
yesOrNoLog = "";
askYOrNo(auth);
);
Thanks in advance for your help!
EDIT
I have done some more work and have found that in my code r5.on is run twice. I tried deleting the array.push("n"). but this does not fix the problem. The array outputs as
[hi, bye, hi, bye]
This is my new code once again I have deleted the requests:
//ASKS IF YOU WANT A NEW SLIDE
function askYOrN(auth)
console.log("askYOrN");
const r4 = readline.createInterface(
input: process.stdin,
output: process.stdout,
terminal: false
);
r4.question("Do you want to add a slide [Y/N]? ", function(yesOrNo)
console.log("aksYOrN question");
yesOrNoLog = yesOrNo;
r4.close();
yOrNCheckCounter = yOrNCheckCounter + 1;
askYOrNCheck(auth);
);
//ASKS IF ANOTHER SLIDE IS NEEDED
function askYOrNCheck(auth)
//DECLARATION FOR THE NUMBER COUNTER [ num ]
var num = 0;
function createSlide(auth)
//AUTHENTICATION
const slides = google.slides(version: 'v1', auth);
//CHANGES txtArray TO STRING
var text = txtArray.join('n');
//CHANGING VARS
var slideId = 'slide_' + num;
var pageId = slideId;
var textId = 'text_box_' + num;
var elementId = textId;
var iIndex = num;
//SLIDE NUMBER
var sNum = num + 1;
console.log(pageId);
//ALL REQUESTS GO IN [ requests ]
var requests = ;
//BATCH UPDATE
return slides.presentations.batchUpdate(
presentationId,
resource:
requests,
,
, (err, res) =>
if (err) return error(err);
console.log("Slide #" + sNum + " has been created");
//INCREASES COUNTER BY 1
num = num + 1;
//ASKS IF A NEW SLIDE WANTS TO BE CREATED
askYOrN(auth);
);
Note:
I renamed askForNew() to askYOrNoCheck().
Once again thanks for your help.
javascript node.js

javascript node.js

edited Nov 13 at 0:50
asked Nov 9 at 6:41


Arkin Solomon
697
697
I highly recommend checking out Inquirer
– FrankerZ
Nov 13 at 0:57
First, I would suggest getting rid of your global variables. Without looking much at the code, it could either cause your current problem or future problems. Instead, pass the necessary variables around (e.g.checkAnswer(providedAnswer) /* ... */
). Second, make sure to separate your functions into meaningful units. For example,askYOrNCheck()
performs 2 actions: checking the user's answer and prompting for the slide text. This will help you think about what you're doing more clearly. Third, consider revisiting your names (e.g.askYOrNCheck
should becheckAnswer
).
– c1moore
Nov 13 at 1:21
The last tip makes the intent of the function more clear. The first few times I read it, I assumed you were going to prompt for the user's answer. While these don't solve your problem, addressing them will make it easier for others to help you and may help you figure out what is happening yourself because your program will be more semantically meaningful and better defined.
– c1moore
Nov 13 at 1:23
Actually, instead ofcheckAnswer
, you may want to use something likeshouldAddSlide(providedAnswer)
that returnstrue
if the user enteredyes
ory
,false
if the user enteredno
orn
, or throws an error if the user entered anything else.
– c1moore
Nov 13 at 1:28
@c1moore Thank you for your suggestions I will definitely go through my code. However I have found the issue, I forgot to close the readline so it was pushing 2 answers instead of one.
– Arkin Solomon
Nov 13 at 1:41
add a comment |
I highly recommend checking out Inquirer
– FrankerZ
Nov 13 at 0:57
First, I would suggest getting rid of your global variables. Without looking much at the code, it could either cause your current problem or future problems. Instead, pass the necessary variables around (e.g.checkAnswer(providedAnswer) /* ... */
). Second, make sure to separate your functions into meaningful units. For example,askYOrNCheck()
performs 2 actions: checking the user's answer and prompting for the slide text. This will help you think about what you're doing more clearly. Third, consider revisiting your names (e.g.askYOrNCheck
should becheckAnswer
).
– c1moore
Nov 13 at 1:21
The last tip makes the intent of the function more clear. The first few times I read it, I assumed you were going to prompt for the user's answer. While these don't solve your problem, addressing them will make it easier for others to help you and may help you figure out what is happening yourself because your program will be more semantically meaningful and better defined.
– c1moore
Nov 13 at 1:23
Actually, instead ofcheckAnswer
, you may want to use something likeshouldAddSlide(providedAnswer)
that returnstrue
if the user enteredyes
ory
,false
if the user enteredno
orn
, or throws an error if the user entered anything else.
– c1moore
Nov 13 at 1:28
@c1moore Thank you for your suggestions I will definitely go through my code. However I have found the issue, I forgot to close the readline so it was pushing 2 answers instead of one.
– Arkin Solomon
Nov 13 at 1:41
I highly recommend checking out Inquirer
– FrankerZ
Nov 13 at 0:57
I highly recommend checking out Inquirer
– FrankerZ
Nov 13 at 0:57
First, I would suggest getting rid of your global variables. Without looking much at the code, it could either cause your current problem or future problems. Instead, pass the necessary variables around (e.g.
checkAnswer(providedAnswer) /* ... */
). Second, make sure to separate your functions into meaningful units. For example, askYOrNCheck()
performs 2 actions: checking the user's answer and prompting for the slide text. This will help you think about what you're doing more clearly. Third, consider revisiting your names (e.g. askYOrNCheck
should be checkAnswer
).– c1moore
Nov 13 at 1:21
First, I would suggest getting rid of your global variables. Without looking much at the code, it could either cause your current problem or future problems. Instead, pass the necessary variables around (e.g.
checkAnswer(providedAnswer) /* ... */
). Second, make sure to separate your functions into meaningful units. For example, askYOrNCheck()
performs 2 actions: checking the user's answer and prompting for the slide text. This will help you think about what you're doing more clearly. Third, consider revisiting your names (e.g. askYOrNCheck
should be checkAnswer
).– c1moore
Nov 13 at 1:21
The last tip makes the intent of the function more clear. The first few times I read it, I assumed you were going to prompt for the user's answer. While these don't solve your problem, addressing them will make it easier for others to help you and may help you figure out what is happening yourself because your program will be more semantically meaningful and better defined.
– c1moore
Nov 13 at 1:23
The last tip makes the intent of the function more clear. The first few times I read it, I assumed you were going to prompt for the user's answer. While these don't solve your problem, addressing them will make it easier for others to help you and may help you figure out what is happening yourself because your program will be more semantically meaningful and better defined.
– c1moore
Nov 13 at 1:23
Actually, instead of
checkAnswer
, you may want to use something like shouldAddSlide(providedAnswer)
that returns true
if the user entered yes
or y
, false
if the user entered no
or n
, or throws an error if the user entered anything else.– c1moore
Nov 13 at 1:28
Actually, instead of
checkAnswer
, you may want to use something like shouldAddSlide(providedAnswer)
that returns true
if the user entered yes
or y
, false
if the user entered no
or n
, or throws an error if the user entered anything else.– c1moore
Nov 13 at 1:28
@c1moore Thank you for your suggestions I will definitely go through my code. However I have found the issue, I forgot to close the readline so it was pushing 2 answers instead of one.
– Arkin Solomon
Nov 13 at 1:41
@c1moore Thank you for your suggestions I will definitely go through my code. However I have found the issue, I forgot to close the readline so it was pushing 2 answers instead of one.
– Arkin Solomon
Nov 13 at 1:41
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
Needed to add r5.close(). It took multiple inputs from one input.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
Needed to add r5.close(). It took multiple inputs from one input.
add a comment |
up vote
0
down vote
accepted
Needed to add r5.close(). It took multiple inputs from one input.
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Needed to add r5.close(). It took multiple inputs from one input.
Needed to add r5.close(). It took multiple inputs from one input.
answered Nov 13 at 1:41


Arkin Solomon
697
697
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53220975%2fnode-js-function-runs-twice-when-called-once-on-promise-return%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6xx8knzslFAUfQtwWzllTramxMKc8j,9bsegWySrM0,SLtI6LDhJd9r1jdlBpZ l,rOaqsgmpl
I highly recommend checking out Inquirer
– FrankerZ
Nov 13 at 0:57
First, I would suggest getting rid of your global variables. Without looking much at the code, it could either cause your current problem or future problems. Instead, pass the necessary variables around (e.g.
checkAnswer(providedAnswer) /* ... */
). Second, make sure to separate your functions into meaningful units. For example,askYOrNCheck()
performs 2 actions: checking the user's answer and prompting for the slide text. This will help you think about what you're doing more clearly. Third, consider revisiting your names (e.g.askYOrNCheck
should becheckAnswer
).– c1moore
Nov 13 at 1:21
The last tip makes the intent of the function more clear. The first few times I read it, I assumed you were going to prompt for the user's answer. While these don't solve your problem, addressing them will make it easier for others to help you and may help you figure out what is happening yourself because your program will be more semantically meaningful and better defined.
– c1moore
Nov 13 at 1:23
Actually, instead of
checkAnswer
, you may want to use something likeshouldAddSlide(providedAnswer)
that returnstrue
if the user enteredyes
ory
,false
if the user enteredno
orn
, or throws an error if the user entered anything else.– c1moore
Nov 13 at 1:28
@c1moore Thank you for your suggestions I will definitely go through my code. However I have found the issue, I forgot to close the readline so it was pushing 2 answers instead of one.
– Arkin Solomon
Nov 13 at 1:41